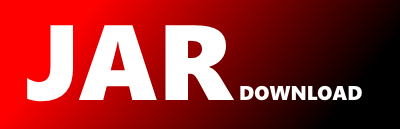
org.eclipse.rdf4j.rio.jsonld.JSONLDHierarchicalProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdf4j-rio-jsonld Show documentation
Show all versions of rdf4j-rio-jsonld Show documentation
Rio parser and writer implementation for the JSON-LD file format.
/*******************************************************************************
* Copyright (c) 2018 Eclipse RDF4J contributors.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*******************************************************************************/
package org.eclipse.rdf4j.rio.jsonld;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Converts a JSON-LD object to a hierarchical form
*
* @author Yasen Marinov
*/
public class JSONLDHierarchicalProcessor {
public static final String ID = "@id";
public static final String GRAPH = "@graph";
/**
* Converts a JSON-LD object to a hierarchical JSON-LD object
*
* @param jsonLdObject JSON-LD object to be converted. Gets modified during processing
* @return hierarchical JSON-LD object
*/
public static Object fromJsonLdObject(Object jsonLdObject) {
return expandInDepth(jsonLdObject);
}
/**
* Expands the JSON-LD object to a hierarchical shape.
*
* As the first level of nodes in the object can be either a triple or a whole graph we first expand the graph nodes
* and after that we expand the default graph.
*
* The different graphs are processed independently to keep them in insulation.
*
* @param input the JSON-LD object. Gets modified during processing.
* @return
*/
private static Object expandInDepth(Object input) {
for (Map graph : (ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy