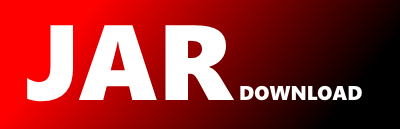
org.eclipse.rdf4j.testsuite.rio.nquads.AbstractNQuadsParserTest Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (c) 2015 Eclipse RDF4J contributors, Aduna, and others.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*******************************************************************************/
package org.eclipse.rdf4j.testsuite.rio.nquads;
import java.io.InputStream;
import org.eclipse.rdf4j.model.IRI;
import org.eclipse.rdf4j.model.Literal;
import org.eclipse.rdf4j.query.BindingSet;
import org.eclipse.rdf4j.query.QueryLanguage;
import org.eclipse.rdf4j.query.TupleQueryResult;
import org.eclipse.rdf4j.repository.Repository;
import org.eclipse.rdf4j.repository.RepositoryConnection;
import org.eclipse.rdf4j.repository.sail.SailRepository;
import org.eclipse.rdf4j.rio.RDFFormat;
import org.eclipse.rdf4j.rio.RDFParser;
import org.eclipse.rdf4j.sail.memory.MemoryStore;
import org.eclipse.rdf4j.testsuite.rio.FailureMode;
import org.eclipse.rdf4j.testsuite.rio.NegativeParserTest;
import org.eclipse.rdf4j.testsuite.rio.PositiveParserTest;
import junit.framework.TestSuite;
/**
* JUnit test for the N-Triples parser that uses the tests that are available
* online.
*/
public abstract class AbstractNQuadsParserTest {
/*-----------*
* Constants *
*-----------*/
/**
* Base directory for W3C N-Triples tests
*/
private static final String TEST_W3C_FILE_BASE_PATH = "/testcases/nquads/";
private static final String TEST_W3C_MANIFEST_URL = TEST_W3C_FILE_BASE_PATH + "manifest.ttl";
private static final String TEST_W3C_MANIFEST_URI_BASE = "http://www.w3.org/2013/N-QuadsTests/manifest.ttl#";
private static final String TEST_W3C_TEST_URI_BASE = "http://www.w3.org/2013/N-QuadsTests/";
/*---------*
* Methods *
*---------*/
public TestSuite createTestSuite() throws Exception {
// Create test suite
TestSuite suite = new TestSuite(this.getClass().getName());
// Add the manifest for W3C test cases to a repository and query it
Repository w3cRepository = new SailRepository(new MemoryStore());
try (RepositoryConnection w3cCon = w3cRepository.getConnection()) {
InputStream inputStream = this.getClass().getResourceAsStream(TEST_W3C_MANIFEST_URL);
w3cCon.add(inputStream, TEST_W3C_MANIFEST_URI_BASE, RDFFormat.TURTLE);
parsePositiveNQuadsSyntaxTests(suite, TEST_W3C_FILE_BASE_PATH, TEST_W3C_TEST_URI_BASE, w3cCon);
parseNegativeNQuadsSyntaxTests(suite, TEST_W3C_FILE_BASE_PATH, TEST_W3C_TEST_URI_BASE, w3cCon);
}
w3cRepository.shutDown();
return suite;
}
private void parsePositiveNQuadsSyntaxTests(TestSuite suite, String fileBasePath, String testLocationBaseUri,
RepositoryConnection con) {
StringBuilder positiveQuery = new StringBuilder();
positiveQuery.append(" PREFIX mf: \n");
positiveQuery.append(" PREFIX qt: \n");
positiveQuery.append(" PREFIX rdft: \n");
positiveQuery.append(" SELECT ?test ?testName ?inputURL ?outputURL \n");
positiveQuery.append(" WHERE { \n");
positiveQuery.append(" ?test a rdft:TestNQuadsPositiveSyntax . ");
positiveQuery.append(" ?test mf:name ?testName . ");
positiveQuery.append(" ?test mf:action ?inputURL . ");
positiveQuery.append(" }");
TupleQueryResult queryResult = con.prepareTupleQuery(QueryLanguage.SPARQL, positiveQuery.toString()).evaluate();
// Add all positive parser tests to the test suite
while (queryResult.hasNext()) {
BindingSet bindingSet = queryResult.next();
IRI nextTestUri = (IRI) bindingSet.getValue("test");
String nextTestName = ((Literal) bindingSet.getValue("testName")).getLabel();
String nextTestFile = removeBase(((IRI) bindingSet.getValue("inputURL")).toString(), testLocationBaseUri);
String nextInputURL = fileBasePath + nextTestFile;
String nextBaseUrl = testLocationBaseUri + nextTestFile;
suite.addTest(new PositiveParserTest(nextTestUri, nextTestName, nextInputURL, null, nextBaseUrl,
createRDFParser(), createRDFParser()));
}
queryResult.close();
}
private void parseNegativeNQuadsSyntaxTests(TestSuite suite, String fileBasePath, String testLocationBaseUri,
RepositoryConnection con) {
StringBuilder negativeQuery = new StringBuilder();
negativeQuery.append(" PREFIX mf: \n");
negativeQuery.append(" PREFIX qt: \n");
negativeQuery.append(" PREFIX rdft: \n");
negativeQuery.append(" SELECT ?test ?testName ?inputURL ?outputURL \n");
negativeQuery.append(" WHERE { \n");
negativeQuery.append(" ?test a rdft:TestNQuadsNegativeSyntax . ");
negativeQuery.append(" ?test mf:name ?testName . ");
negativeQuery.append(" ?test mf:action ?inputURL . ");
negativeQuery.append(" }");
TupleQueryResult queryResult = con.prepareTupleQuery(QueryLanguage.SPARQL, negativeQuery.toString()).evaluate();
// Add all negative parser tests to the test suite
while (queryResult.hasNext()) {
BindingSet bindingSet = queryResult.next();
IRI nextTestUri = (IRI) bindingSet.getValue("test");
String nextTestName = ((Literal) bindingSet.getValue("testName")).toString();
String nextTestFile = removeBase(((IRI) bindingSet.getValue("inputURL")).toString(), testLocationBaseUri);
String nextInputURL = fileBasePath + nextTestFile;
String nextBaseUrl = testLocationBaseUri + nextTestFile;
suite.addTest(new NegativeParserTest(nextTestUri, nextTestName, nextInputURL, nextBaseUrl,
createRDFParser(), FailureMode.DO_NOT_IGNORE_FAILURE));
}
queryResult.close();
}
protected abstract RDFParser createRDFParser();
private String removeBase(String baseUrl, String redundantBaseUrl) {
if (baseUrl.startsWith(redundantBaseUrl)) {
return baseUrl.substring(redundantBaseUrl.length());
}
return baseUrl;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy