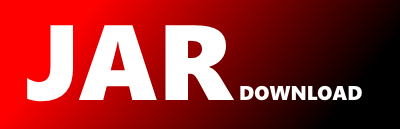
org.eclipse.rdf4j.sail.lucene.PropertyCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdf4j-sail-lucene-api Show documentation
Show all versions of rdf4j-sail-lucene-api Show documentation
StackableSail API offering full-text search on literals, based on Apache Lucene.
The newest version!
/*******************************************************************************
* Copyright (c) 2015 Eclipse RDF4J contributors, Aduna, and others.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*******************************************************************************/
package org.eclipse.rdf4j.sail.lucene;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Class to cache SearchDocument.hasProperty() calls.
*/
class PropertyCache {
private final SearchDocument doc;
private Map> cachedProperties;
PropertyCache(SearchDocument doc) {
this.doc = doc;
}
boolean hasProperty(String name, String value) {
boolean found;
Set cachedValues = getCachedValues(name);
if (cachedValues != null) {
found = cachedValues.contains(value);
} else {
found = false;
List docValues = doc.getProperty(name);
if (docValues != null) {
cachedValues = new HashSet<>(docValues.size());
for (String docValue : docValues) {
cachedValues.add(docValue);
if (docValue.equals(value)) {
found = true;
// don't break - cache all docValues
}
}
} else {
cachedValues = Collections.emptySet();
}
setCachedValues(name, cachedValues);
}
return found;
}
private Set getCachedValues(String name) {
return (cachedProperties != null) ? cachedProperties.get(name) : null;
}
private void setCachedValues(String name, Set values) {
if (cachedProperties == null) {
cachedProperties = new HashMap<>();
}
cachedProperties.put(name, values);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy