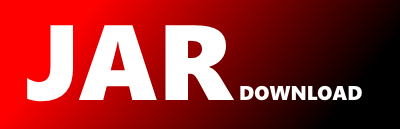
org.eclipse.rdf4j.spring.resultcache.CachedGraphQueryResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdf4j-spring Show documentation
Show all versions of rdf4j-spring Show documentation
Spring integration for RDF4J
The newest version!
/*******************************************************************************
* Copyright (c) 2021 Eclipse RDF4J contributors.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*******************************************************************************/
package org.eclipse.rdf4j.spring.resultcache;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.function.Consumer;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import org.eclipse.rdf4j.model.Statement;
import org.eclipse.rdf4j.query.GraphQueryResult;
import org.eclipse.rdf4j.query.QueryEvaluationException;
/**
* @author Florian Kleedorfer
* @since 4.0.0
*/
public class CachedGraphQueryResult implements GraphQueryResult {
private final List statements;
private Iterator replayingIterator;
private final Map namespaces;
CachedGraphQueryResult(List statements, Map namespaces) {
this.statements = new ArrayList<>(statements);
this.namespaces = new HashMap<>();
this.namespaces.putAll(namespaces);
this.replayingIterator = statements.iterator();
}
@Override
public Map getNamespaces() throws QueryEvaluationException {
return namespaces;
}
@Override
public Iterator iterator() {
return replayingIterator;
}
@Override
public void close() throws QueryEvaluationException {
this.replayingIterator = null;
}
@Override
public boolean hasNext() throws QueryEvaluationException {
return replayingIterator.hasNext();
}
@Override
public Statement next() throws QueryEvaluationException {
return this.replayingIterator.next();
}
@Override
public void remove() throws QueryEvaluationException {
throw new UnsupportedOperationException("Remove is not supported");
}
@Override
public Stream stream() {
return StreamSupport.stream(
Spliterators.spliteratorUnknownSize(this.replayingIterator, Spliterator.ORDERED),
false);
}
@Override
public void forEach(Consumer super Statement> action) {
statements.forEach(action);
}
@Override
public Spliterator spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), Spliterator.ORDERED);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy