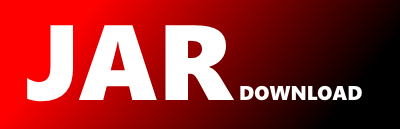
org.eclipse.rdf4j.federated.algebra.NTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rdf4j-tools-federation Show documentation
Show all versions of rdf4j-tools-federation Show documentation
A federation engine for virtually integrating SPARQL endpoints
/*******************************************************************************
* Copyright (c) 2019 Eclipse RDF4J contributors.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*******************************************************************************/
package org.eclipse.rdf4j.federated.algebra;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.eclipse.rdf4j.common.order.AvailableStatementOrder;
import org.eclipse.rdf4j.federated.structures.QueryInfo;
import org.eclipse.rdf4j.query.algebra.AbstractQueryModelNode;
import org.eclipse.rdf4j.query.algebra.QueryModelNode;
import org.eclipse.rdf4j.query.algebra.QueryModelVisitor;
import org.eclipse.rdf4j.query.algebra.TupleExpr;
import org.eclipse.rdf4j.query.algebra.Var;
/**
* Base class for any nary-tuple expression
*
* @author Andreas Schwarte
*
* @see NJoin
* @see NUnion
*/
public abstract class NTuple extends AbstractQueryModelNode implements TupleExpr, QueryRef {
private static final long serialVersionUID = -4899531533519154174L;
protected final List args;
protected final QueryInfo queryInfo;
/**
* Construct an nary-tuple. Note that the parentNode of all arguments is set to this instance.
*
* @param args
*/
public NTuple(List args, QueryInfo queryInfo) {
super();
this.queryInfo = queryInfo;
this.args = args;
for (TupleExpr expr : args) {
expr.setParentNode(this);
}
}
public TupleExpr getArg(int i) {
return args.get(i);
}
public List getArgs() {
return args;
}
public int getNumberOfArguments() {
return args.size();
}
@Override
public void visitChildren(QueryModelVisitor visitor) throws X {
for (TupleExpr expr : args) {
expr.visit(visitor);
}
}
@Override
public NTuple clone() {
return (NTuple) super.clone();
}
@Override
public Set getAssuredBindingNames() {
Set res = new LinkedHashSet<>(16);
for (TupleExpr e : args) {
res.addAll(e.getAssuredBindingNames());
}
return res;
}
@Override
public Set getBindingNames() {
Set res = new LinkedHashSet<>(16);
for (TupleExpr e : args) {
res.addAll(e.getBindingNames());
}
return res;
}
@Override
public void replaceChildNode(QueryModelNode current, QueryModelNode replacement) {
int index = args.indexOf(current);
if (index >= 0) {
args.set(index, (TupleExpr) replacement);
replacement.setParentNode(this);
} else {
throw new IllegalArgumentException("Node is not a child node: " + current);
}
}
@Override
public void visit(QueryModelVisitor visitor) throws X {
visitor.meetOther(this);
}
@Override
public QueryInfo getQueryInfo() {
return queryInfo;
}
@Override
public Set getSupportedOrders(AvailableStatementOrder tripleSource) {
throw new UnsupportedOperationException("Not implemented yet");
}
@Override
public void setOrder(Var var) {
throw new UnsupportedOperationException("Not implemented yet");
}
@Override
public Var getOrder() {
throw new UnsupportedOperationException("Not implemented yet");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy