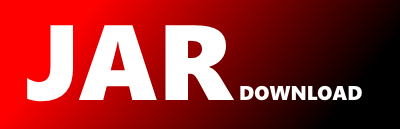
org.eclipse.rdf4j.common.iteration.ExceptionConvertingIteration Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2015 Eclipse RDF4J contributors, Aduna, and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Distribution License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*******************************************************************************/
package org.eclipse.rdf4j.common.iteration;
import java.util.NoSuchElementException;
/**
* A CloseableIteration that converts an arbitrary iteration to an iteration with exceptions of type
* X. Subclasses need to override {@link #convert(Exception)} to do the conversion.
*/
public abstract class ExceptionConvertingIteration
extends AbstractCloseableIteration
{
/*-----------*
* Variables *
*-----------*/
/**
* The underlying Iteration.
*/
private final Iteration extends E, ? extends Exception> iter;
/*--------------*
* Constructors *
*--------------*/
/**
* Creates a new ExceptionConvertingIteration that operates on the supplied iteration.
*
* @param iter
* The Iteration that this ExceptionConvertingIteration operates on, must not be
* null.
*/
public ExceptionConvertingIteration(Iteration extends E, ? extends Exception> iter) {
assert iter != null;
this.iter = iter;
}
/*---------*
* Methods *
*---------*/
/**
* Converts an exception from the underlying iteration to an exception of type X.
*/
protected abstract X convert(Exception e);
/**
* Checks whether the underlying Iteration contains more elements.
*
* @return true if the underlying Iteration contains more elements, false otherwise.
* @throws X
*/
public boolean hasNext()
throws X
{
try {
return iter.hasNext();
}
catch (Exception e) {
throw convert(e);
}
}
/**
* Returns the next element from the wrapped Iteration.
*
* @throws X
* @throws java.util.NoSuchElementException
* If all elements have been returned.
* @throws IllegalStateException
* If the Iteration has been closed.
*/
public E next()
throws X
{
try {
return iter.next();
}
catch (NoSuchElementException e) {
throw e;
}
catch (IllegalStateException e) {
throw e;
}
catch (Exception e) {
throw convert(e);
}
}
/**
* Calls remove() on the underlying Iteration.
*
* @throws UnsupportedOperationException
* If the wrapped Iteration does not support the remove operation.
* @throws IllegalStateException
* If the Iteration has been closed, or if {@link #next} has not yet been called, or
* {@link #remove} has already been called after the last call to {@link #next}.
*/
public void remove()
throws X
{
try {
iter.remove();
}
catch (UnsupportedOperationException e) {
throw e;
}
catch (IllegalStateException e) {
throw e;
}
catch (Exception e) {
throw convert(e);
}
}
/**
* Closes this Iteration as well as the wrapped Iteration if it happens to be a {@link CloseableIteration}
* .
*/
@Override
protected void handleClose()
throws X
{
super.handleClose();
try {
Iterations.closeCloseable(iter);
}
catch (Exception e) {
throw convert(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy