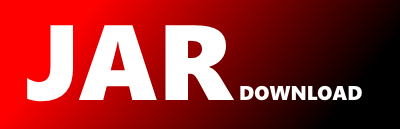
org.eclipse.scout.rt.rest.RestApplication Maven / Gradle / Ivy
/*
* Copyright (c) 2010, 2023 BSI Business Systems Integration AG
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*/
package org.eclipse.scout.rt.rest;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.stream.Collectors;
import jakarta.ws.rs.core.Application;
import org.eclipse.scout.rt.platform.ApplicationScoped;
import org.eclipse.scout.rt.platform.BEANS;
import org.eclipse.scout.rt.platform.util.StreamUtility;
import org.eclipse.scout.rt.platform.util.StringUtility;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* JAX-RS REST application registering all provided classes, singletons and properties to the JAX-RS context.
*
* @see IRestApplicationClassesContributor
* @see IRestApplicationSingletonsContributor
* @see IRestApplicationPropertiesContributor
*/
public class RestApplication extends Application {
private static final Logger LOG = LoggerFactory.getLogger(RestApplication.class);
private final Set> m_classes;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy