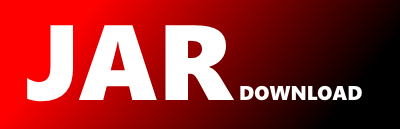
org.eclipse.swt.widgets.TabItem Maven / Gradle / Ivy
/******************************************************************************* * Copyright (c) 2000, 2013 IBM Corporation and others. * All rights reserved. This program and the accompanying materials * are made available under the terms of the Eclipse Public License v1.0 * which accompanies this distribution, and is available at * http://www.eclipse.org/legal/epl-v10.html * * Contributors: * IBM Corporation - initial API and implementation *******************************************************************************/ package org.eclipse.swt.widgets; import org.eclipse.swt.internal.win32.*; import org.eclipse.swt.*; import org.eclipse.swt.graphics.*; /** * Instances of this class represent a selectable user interface object * corresponding to a tab for a page in a tab folder. *
. **
*- Styles:
*- (none)
*- Events:
*- (none)
** IMPORTANT: This class is not intended to be subclassed. *
* * @see TabFolder, TabItem snippets * @see Sample code and further information * @noextend This class is not intended to be subclassed by clients. */ public class TabItem extends Item { TabFolder parent; Control control; String toolTipText; /** * Constructs a new instance of this class given its parent * (which must be aTabFolder
) and a style value * describing its behavior and appearance. The item is added * to the end of the items maintained by its parent. ** The style value is either one of the style constants defined in * class
* * @param parent a composite control which will be the parent of the new instance (cannot be null) * @param style the style of control to construct * * @exception IllegalArgumentExceptionSWT
which is applicable to instances of this * class, or must be built by bitwise OR'ing together * (that is, using theint
"|" operator) two or more * of thoseSWT
style constants. The class description * lists the style constants that are applicable to the class. * Style bits are also inherited from superclasses. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
**
* * @see SWT * @see Widget#checkSubclass * @see Widget#getStyle */ public TabItem (TabFolder parent, int style) { super (parent, style); this.parent = parent; parent.createItem (this, parent.getItemCount ()); } /** * Constructs a new instance of this class given its parent * (which must be a- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*TabFolder
), a style value * describing its behavior and appearance, and the index * at which to place it in the items maintained by its parent. ** The style value is either one of the style constants defined in * class
* * @param parent a composite control which will be the parent of the new instance (cannot be null) * @param style the style of control to construct * @param index the zero-relative index to store the receiver in its parent * * @exception IllegalArgumentExceptionSWT
which is applicable to instances of this * class, or must be built by bitwise OR'ing together * (that is, using theint
"|" operator) two or more * of thoseSWT
style constants. The class description * lists the style constants that are applicable to the class. * Style bits are also inherited from superclasses. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
*- ERROR_INVALID_RANGE - if the index is not between 0 and the number of elements in the parent (inclusive)
**
* * @see SWT * @see Widget#checkSubclass * @see Widget#getStyle */ public TabItem (TabFolder parent, int style, int index) { super (parent, style); this.parent = parent; parent.createItem (this, index); } void _setText (int index, String string) { /* * Bug in Windows. In version 6.00 of COMCTL32.DLL, tab * items with an image and a label that includes '&' cause * the tab to draw incorrectly (even when doubled '&&'). * The image overlaps the label. The fix is to remove * all '&' characters from the string. */ if (OS.COMCTL32_MAJOR >= 6 && image != null) { if (string.indexOf ('&') != -1) { int length = string.length (); char[] text = new char [length]; string.getChars ( 0, length, text, 0); int i = 0, j = 0; for (i=0; i- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*null * @return the control * * @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
TabFolder
.
*
* @return the receiver's parent
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* @param control the new control (or null) * * @exception IllegalArgumentException
-
*
- ERROR_INVALID_ARGUMENT - if the control has been disposed *
- ERROR_INVALID_PARENT - if the control is not in the same widget tree *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* Mnemonics are indicated by an '&' that causes the next * character to be the mnemonic. When the user presses a * key sequence that matches the mnemonic, a selection * event occurs. On most platforms, the mnemonic appears * underlined but may be emphasised in a platform specific * manner. The mnemonic indicator character '&' can be * escaped by doubling it in the string, causing a single * '&' to be displayed. *
* * @param string the new text * * @exception IllegalArgumentException-
*
- ERROR_NULL_ARGUMENT - if the text is null *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* The mnemonic indicator (character '&') is not displayed in a tool tip. * To display a single '&' in the tool tip, the character '&' can be * escaped by doubling it in the string. *
* * @param string the new tool tip text (or null) * * @exception SWTException-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *