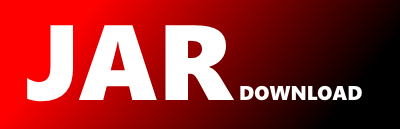
org.eclipse.sisu.bean.BeanPropertiesTest Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (c) 2010, 2013 Sonatype, Inc.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Stuart McCulloch (Sonatype, Inc.) - initial API and implementation
*******************************************************************************/
package org.eclipse.sisu.bean;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.lang.reflect.Field;
import java.lang.reflect.Member;
import java.lang.reflect.Method;
import java.math.BigDecimal;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import javax.inject.Named;
import javax.inject.Singleton;
import junit.framework.TestCase;
import com.google.inject.TypeLiteral;
import com.google.inject.util.Types;
@SuppressWarnings( "unused" )
public class BeanPropertiesTest
extends TestCase
{
@Retention( RetentionPolicy.RUNTIME )
@interface Metadata
{
String value();
}
@Target( ElementType.METHOD )
@Retention( RetentionPolicy.RUNTIME )
@interface MethodMetadata
{
String value();
}
@Target( ElementType.FIELD )
@Retention( RetentionPolicy.RUNTIME )
@interface FieldMetadata
{
String value();
}
@Target( { ElementType.FIELD, ElementType.METHOD } )
@Retention( RetentionPolicy.RUNTIME )
@interface MultiMetadata
{
String value();
}
static interface A
{
String name = "";
void setName( String name );
}
static class B
{
static void setName( final String name )
{
}
}
static class C
{
final String name = "";
}
static class D
{
public D()
{
}
private void setName( final String name )
{
}
}
static class E
{
void setName()
{
}
void setName( final String firstName, final String lastName )
{
}
void name( final String _name )
{
}
}
static class F
{
void setName( final String name )
{
}
void setName()
{
}
String name;
void setName( final String firstName, final String lastName )
{
}
void name( final String _name )
{
}
}
static class G
{
List names;
void setMap( final Map map )
{
}
}
static abstract class IBase
{
public abstract void setId( T id );
}
static class H
extends IBase
{
private volatile String vid = "test";
private static Internal internal = new Internal();
static class Internal
{
String m_id;
}
@Override
public void setId( final String _id )
{
internal.m_id = _id;
}
@Override
public String toString()
{
return vid + "@" + internal.m_id;
}
}
static class I
{
@Singleton
@Named( "bar" )
String bar;
@Singleton
@Named( "foo" )
void setFoo( final String foo )
{
}
}
static class J
{
String a;
String b;
String c;
}
static class K
{
void setName( final String name )
{
throw new RuntimeException();
}
}
static class L
{
void setter( final String value )
{
}
}
static class M
{
void set( final String value )
{
}
}
static class N
{
@Metadata( "Field1" )
@MultiMetadata( "A" )
@FieldMetadata( "1" )
int value;
@MethodMetadata( "1" )
void init()
{
}
@MethodMetadata( "2" )
void setValue( final int value )
{
this.value = value;
}
}
static class O1
{
private String a1;
void setA( final String a )
{
}
}
@IgnoreSetters
static class O2
extends O1
{
private String b2;
void setB( final String b )
{
}
}
static class O3
extends O2
{
void setC( final String c )
{
}
}
public void testInterface()
{
for ( final BeanProperty> bp : new BeanProperties( A.class ) )
{
fail( "Expected no bean properties" );
}
}
public void testEmptyClass()
{
for ( final BeanProperty> bp : new BeanProperties( B.class ) )
{
fail( "Expected no bean properties" );
}
}
public void testPropertyField()
{
final Iterator> i = new BeanProperties( C.class ).iterator();
assertEquals( "name", i.next().getName() );
assertFalse( i.hasNext() );
}
public void testPropertySetter()
{
final Iterator> i = new BeanProperties( D.class ).iterator();
assertEquals( "name", i.next().getName() );
assertFalse( i.hasNext() );
}
public void testHashCodeAndEquals()
throws Exception
{
final BeanProperty
© 2015 - 2025 Weber Informatics LLC | Privacy Policy