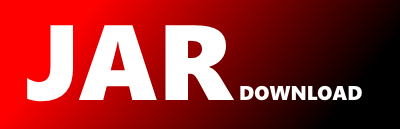
org.eclipse.sisu.space.DeferredClassTest Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (c) 2010, 2013 Sonatype, Inc.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Stuart McCulloch (Sonatype, Inc.) - initial API and implementation
*******************************************************************************/
package org.eclipse.sisu.space;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import junit.framework.TestCase;
import org.eclipse.sisu.inject.DeferredClass;
import org.eclipse.sisu.inject.DeferredProvider;
public class DeferredClassTest
extends TestCase
{
URLClassLoader testLoader;
@Override
protected void setUp()
throws MalformedURLException
{
testLoader = URLClassLoader.newInstance( new URL[] { new File( "target/classes" ).toURI().toURL() }, null );
}
private static class Dummy
{
}
public void testStrongDeferredClass()
{
final String clazzName = Dummy.class.getName();
final ClassSpace space = new URLClassSpace( testLoader );
final DeferredClass> clazz = space.deferLoadClass( clazzName );
assertEquals( clazzName, clazz.getName() );
assertEquals( clazzName, clazz.load().getName() );
assertFalse( Dummy.class.equals( clazz.load() ) );
assertEquals( ( 17 * 31 + clazzName.hashCode() ) * 31 + space.hashCode(), clazz.hashCode() );
assertEquals( clazz, clazz );
assertEquals( new NamedClass
© 2015 - 2025 Weber Informatics LLC | Privacy Policy