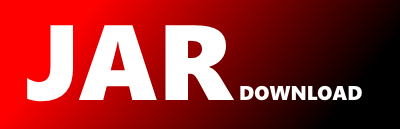
org.eclipse.steady.shared.json.model.ConstructChangeInDependency Maven / Gradle / Ivy
/**
* This file is part of Eclipse Steady.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* SPDX-FileCopyrightText: Copyright (c) 2018-2020 SAP SE or an SAP affiliate company and Eclipse Steady contributors
*/
package org.eclipse.steady.shared.json.model;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
/**
* ConstructChangeInDependency class.
*/
@JsonInclude(JsonInclude.Include.ALWAYS)
@JsonIgnoreProperties(ignoreUnknown = false)
public class ConstructChangeInDependency implements Serializable {
private Trace trace;
private Boolean traced;
private Boolean reachable;
private Boolean inArchive;
private Boolean affected;
private Boolean classInArchive;
private Boolean equalChangeType;
private String overall_change;
private ConstructChange constructChange;
/**
* Constructor for ConstructChangeInDependency.
*/
public ConstructChangeInDependency() {
super();
}
/**
* Getter for the field constructChange
.
*
* @return a {@link org.eclipse.steady.shared.json.model.ConstructChange} object.
*/
public ConstructChange getConstructChange() {
return constructChange;
}
/**
* Setter for the field constructChange
.
*
* @param constructChange a {@link org.eclipse.steady.shared.json.model.ConstructChange} object.
*/
public void setConstructChange(ConstructChange constructChange) {
this.constructChange = constructChange;
}
/**
* Getter for the field trace
.
*
* @return a {@link org.eclipse.steady.shared.json.model.Trace} object.
*/
public Trace getTrace() {
return trace;
}
/**
* Setter for the field trace
.
*
* @param trace a {@link org.eclipse.steady.shared.json.model.Trace} object.
*/
public void setTrace(Trace trace) {
this.trace = trace;
}
/**
* Getter for the field traced
.
*
* @return a {@link java.lang.Boolean} object.
*/
public Boolean getTraced() {
return traced;
}
/**
* Setter for the field traced
.
*
* @param traced a {@link java.lang.Boolean} object.
*/
public void setTraced(Boolean traced) {
this.traced = traced;
}
/**
* Getter for the field inArchive
.
*
* @return a {@link java.lang.Boolean} object.
*/
public Boolean getInArchive() {
return inArchive;
}
/**
* Setter for the field inArchive
.
*
* @param inArchive a {@link java.lang.Boolean} object.
*/
public void setInArchive(Boolean inArchive) {
this.inArchive = inArchive;
}
/**
* Getter for the field reachable
.
*
* @return a {@link java.lang.Boolean} object.
*/
public Boolean getReachable() {
return reachable;
}
/**
* Setter for the field reachable
.
*
* @param reachable a {@link java.lang.Boolean} object.
*/
public void setReachable(Boolean reachable) {
this.reachable = reachable;
}
/**
* isAffected.
*
* @return a boolean.
*/
public boolean isAffected() {
return affected;
}
/**
* Setter for the field affected
.
*
* @param affected a boolean.
*/
public void setAffected(boolean affected) {
this.affected = affected;
}
/**
* isClassInArchive.
*
* @return a boolean.
*/
public boolean isClassInArchive() {
return classInArchive;
}
/**
* Setter for the field classInArchive
.
*
* @param classInArchive a boolean.
*/
public void setClassInArchive(boolean classInArchive) {
this.classInArchive = classInArchive;
}
/**
* isEqualChangeType.
*
* @return a boolean.
*/
public boolean isEqualChangeType() {
return equalChangeType;
}
/**
* Setter for the field equalChangeType
.
*
* @param equalChangeType a boolean.
*/
public void setEqualChangeType(boolean equalChangeType) {
this.equalChangeType = equalChangeType;
}
/**
* Getter for the field overall_change
.
*
* @return a {@link java.lang.String} object.
*/
public String getOverall_change() {
return overall_change;
}
/**
* Setter for the field overall_change
.
*
* @param overall_change a {@link java.lang.String} object.
*/
public void setOverall_change(String overall_change) {
this.overall_change = overall_change;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy