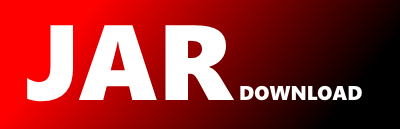
org.eclipse.steady.shared.json.model.Library Maven / Gradle / Ivy
/**
* This file is part of Eclipse Steady.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* SPDX-FileCopyrightText: Copyright (c) 2018-2020 SAP SE or an SAP affiliate company and Eclipse Steady contributors
*/
package org.eclipse.steady.shared.json.model;
import java.io.Serializable;
import java.util.Collection;
import org.eclipse.steady.shared.enums.DigestAlgorithm;
import org.eclipse.steady.shared.json.model.view.Views;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonView;
/**
* Library class.
*/
@JsonInclude(JsonInclude.Include.ALWAYS)
@JsonIgnoreProperties(
ignoreUnknown = true,
value = {"constructCounter", "constructTypeCounters"},
allowGetters = true)
public class Library implements Serializable {
private static final long serialVersionUID = 1L;
private String digest;
private DigestAlgorithm digestAlgorithm;
@JsonFormat(
shape = JsonFormat.Shape.STRING,
pattern = "yyyy-MM-dd'T'HH:mm:ss.SSSZ",
timezone = "GMT")
private java.util.Calendar createdAt;
@JsonView(Views.LibDetails.class)
private Collection properties;
@JsonView(Views.LibDetails.class)
private Collection constructs;
/**
* Library identifier used for publishing the library in package repositories such as Maven Central or PyPI.
*/
private LibraryId libraryId;
/**
* Other library identifiers found within the library.
* Could be many in case a a library bundles (includes) the content of several other libraries (e.g., Uber JARs).
*/
private Collection bundledLibraryIds;
/**
* True if the library provider or a trusted software repository confirms the mapping of SHA1 to human-readable ID, false otherwise.
*/
private Boolean wellknownDigest;
/**
* The URL used to verify the digest. Will be empty if none of the available
* package repositories was able to confirm the digest.
*/
@JsonIgnoreProperties(
value = {"digestVerificationUrl"},
allowGetters = true)
private String digestVerificationUrl;
/**
* Constructor for Library.
*/
public Library() {
super();
}
/**
* Constructor for Library.
*
* @param digest a {@link java.lang.String} object.
*/
public Library(String digest) {
super();
this.digest = digest;
}
/**
* Getter for the field digest
.
*
* @return a {@link java.lang.String} object.
*/
public String getDigest() {
return digest;
}
/**
* Setter for the field digest
.
*
* @param digest a {@link java.lang.String} object.
*/
public void setDigest(String digest) {
this.digest = digest;
}
/**
* Getter for the field digestAlgorithm
.
*
* @return a {@link org.eclipse.steady.shared.enums.DigestAlgorithm} object.
*/
public DigestAlgorithm getDigestAlgorithm() {
return digestAlgorithm;
}
/**
* Setter for the field digestAlgorithm
.
*
* @param digestAlgorithm a {@link org.eclipse.steady.shared.enums.DigestAlgorithm} object.
*/
public void setDigestAlgorithm(DigestAlgorithm digestAlgorithm) {
this.digestAlgorithm = digestAlgorithm;
}
/**
* Returns true if the library has a digest and a digest algorithm, false otherwise.
*
* @return a boolean.
*/
public boolean hasValidDigest() {
return this.getDigest() != null && this.getDigestAlgorithm() != null;
}
/**
* Getter for the field createdAt
.
*
* @return a {@link java.util.Calendar} object.
*/
public java.util.Calendar getCreatedAt() {
return createdAt;
}
/**
* Setter for the field createdAt
.
*
* @param createdAt a {@link java.util.Calendar} object.
*/
public void setCreatedAt(java.util.Calendar createdAt) {
this.createdAt = createdAt;
}
/**
* Getter for the field properties
.
*
* @return a {@link java.util.Collection} object.
*/
public Collection getProperties() {
return properties;
}
/**
* Setter for the field properties
.
*
* @param properties a {@link java.util.Collection} object.
*/
public void setProperties(Collection properties) {
this.properties = properties;
}
/**
* Getter for the field constructs
.
*
* @return a {@link java.util.Collection} object.
*/
public Collection getConstructs() {
return constructs;
}
/**
* Setter for the field constructs
.
*
* @param constructs a {@link java.util.Collection} object.
*/
public void setConstructs(Collection constructs) {
this.constructs = constructs;
}
/**
* Getter for the field libraryId
.
*
* @return a {@link org.eclipse.steady.shared.json.model.LibraryId} object.
*/
public LibraryId getLibraryId() {
return libraryId;
}
/**
* Setter for the field libraryId
.
*
* @param _library_id a {@link org.eclipse.steady.shared.json.model.LibraryId} object.
*/
public void setLibraryId(LibraryId _library_id) {
this.libraryId = _library_id;
}
/**
* Getter for the field bundledLibraryIds
.
*
* @return a {@link java.util.Collection} object.
*/
public Collection getBundledLibraryIds() {
return this.bundledLibraryIds;
}
/**
* Setter for the field bundledLibraryIds
.
*
* @param _libids a {@link java.util.Collection} object.
*/
public void setBundledLibraryIds(Collection _libids) {
this.bundledLibraryIds = _libids;
}
/**
* isWellknownDigest.
*
* @return a boolean.
*/
public boolean isWellknownDigest() {
return wellknownDigest != null && wellknownDigest.equals(true);
}
/**
* Getter for the field wellknownDigest
.
*
* @return a {@link java.lang.Boolean} object.
*/
public Boolean getWellknownDigest() {
return wellknownDigest;
}
/**
* Setter for the field wellknownDigest
.
*
* @param wellknownDigest a {@link java.lang.Boolean} object.
*/
public void setWellknownDigest(Boolean wellknownDigest) {
this.wellknownDigest = wellknownDigest;
}
/**
* Getter for the field digestVerificationUrl
.
*
* @return a {@link java.lang.String} object.
*/
public String getDigestVerificationUrl() {
return digestVerificationUrl;
}
/**
* Setter for the field digestVerificationUrl
.
*
* @param digestVerificationUrl a {@link java.lang.String} object.
*/
public void setDigestVerificationUrl(String digestVerificationUrl) {
this.digestVerificationUrl = digestVerificationUrl;
}
/**
* countConstructs.
*
* @return a int.
*/
@JsonProperty(value = "constructCounter")
@JsonView(Views.LibDetails.class)
public int countConstructs() {
return (this.getConstructs() == null ? 0 : this.getConstructs().size());
}
/**
* countConstructTypes.
*
* @return a {@link org.eclipse.steady.shared.json.model.ConstructIdFilter} object.
*/
@JsonProperty(value = "constructTypeCounters")
@JsonView(Views.LibDetails.class)
public ConstructIdFilter countConstructTypes() {
return new ConstructIdFilter(this.getConstructs());
}
/** {@inheritDoc} */
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((digest == null) ? 0 : digest.hashCode());
return result;
}
/** {@inheritDoc} */
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (getClass() != obj.getClass()) return false;
Library other = (Library) obj;
if (digest == null) {
if (other.digest != null) return false;
} else if (!digest.equals(other.digest)) return false;
return true;
}
/** {@inheritDoc} */
@Override
public final String toString() {
return this.toString(false);
}
/**
* Returns a short or long string representation of the library.
*
* @param _deep a boolean.
* @return a {@link java.lang.String} object.
*/
public final String toString(boolean _deep) {
final StringBuilder builder = new StringBuilder();
if (_deep) {
builder
.append("Library ")
.append(this.toString(false))
.append(System.getProperty("line.separator"));
for (ConstructId cid : this.getConstructs()) {
builder
.append(" ConstructId ")
.append(cid)
.append(System.getProperty("line.separator"));
}
} else {
builder.append("[").append(this.getDigest()).append("]");
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy