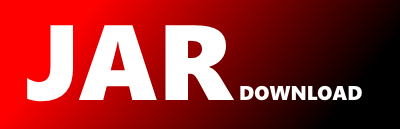
org.eclipse.swt.widgets.Shell Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2000, 2013 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.widgets;
import org.eclipse.swt.*;
import org.eclipse.swt.internal.*;
import org.eclipse.swt.internal.gtk.*;
import org.eclipse.swt.graphics.*;
import org.eclipse.swt.events.*;
/**
* Instances of this class represent the "windows"
* which the desktop or "window manager" is managing.
* Instances that do not have a parent (that is, they
* are built using the constructor, which takes a
* Display
as the argument) are described
* as top level shells. Instances that do have
* a parent are described as secondary or
* dialog shells.
*
* Instances are always displayed in one of the maximized,
* minimized or normal states:
*
* -
* When an instance is marked as maximized, the
* window manager will typically resize it to fill the
* entire visible area of the display, and the instance
* is usually put in a state where it can not be resized
* (even if it has style
RESIZE
) until it is
* no longer maximized.
* -
* When an instance is in the normal state (neither
* maximized or minimized), its appearance is controlled by
* the style constants which were specified when it was created
* and the restrictions of the window manager (see below).
*
-
* When an instance has been marked as minimized,
* its contents (client area) will usually not be visible,
* and depending on the window manager, it may be
* "iconified" (that is, replaced on the desktop by a small
* simplified representation of itself), relocated to a
* distinguished area of the screen, or hidden. Combinations
* of these changes are also possible.
*
*
*
* The modality of an instance may be specified using
* style bits. The modality style bits are used to determine
* whether input is blocked for other shells on the display.
* The PRIMARY_MODAL
style allows an instance to block
* input to its parent. The APPLICATION_MODAL
style
* allows an instance to block input to every other shell in the
* display. The SYSTEM_MODAL
style allows an instance
* to block input to all shells, including shells belonging to
* different applications.
*
* Note: The styles supported by this class are treated
* as HINTs, since the window manager for the
* desktop on which the instance is visible has ultimate
* control over the appearance and behavior of decorations
* and modality. For example, some window managers only
* support resizable windows and will always assume the
* RESIZE style, even if it is not set. In addition, if a
* modality style is not supported, it is "upgraded" to a
* more restrictive modality style that is supported. For
* example, if PRIMARY_MODAL
is not supported,
* it would be upgraded to APPLICATION_MODAL
.
* A modality style may also be "downgraded" to a less
* restrictive style. For example, most operating systems
* no longer support SYSTEM_MODAL
because
* it can freeze up the desktop, so this is typically
* downgraded to APPLICATION_MODAL
.
*
* - Styles:
* - BORDER, CLOSE, MIN, MAX, NO_TRIM, RESIZE, TITLE, ON_TOP, TOOL, SHEET
* - APPLICATION_MODAL, MODELESS, PRIMARY_MODAL, SYSTEM_MODAL
* - Events:
* - Activate, Close, Deactivate, Deiconify, Iconify
*
* Class SWT
provides two "convenience constants"
* for the most commonly required style combinations:
*
* SHELL_TRIM
* -
* the result of combining the constants which are required
* to produce a typical application top level shell: (that
* is,
CLOSE | TITLE | MIN | MAX | RESIZE
)
*
* DIALOG_TRIM
* -
* the result of combining the constants which are required
* to produce a typical application dialog shell: (that
* is,
TITLE | CLOSE | BORDER
)
*
*
*
*
* Note: Only one of the styles APPLICATION_MODAL, MODELESS,
* PRIMARY_MODAL and SYSTEM_MODAL may be specified.
*
* IMPORTANT: This class is not intended to be subclassed.
*
*
* @see Decorations
* @see SWT
* @see Shell snippets
* @see SWT Example: ControlExample
* @see Sample code and further information
* @noextend This class is not intended to be subclassed by clients.
*/
public class Shell extends Decorations {
long /*int*/ shellHandle, tooltipsHandle, tooltipWindow, group, modalGroup;
boolean mapped, moved, resized, opened, fullScreen, showWithParent, modified, center;
int oldX, oldY, oldWidth, oldHeight;
int minWidth, minHeight;
Control lastActive;
ToolTip [] toolTips;
static final int MAXIMUM_TRIM = 128;
static final int BORDER = 3;
/**
* Constructs a new instance of this class. This is equivalent
* to calling Shell((Display) null)
.
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*/
public Shell () {
this ((Display) null);
}
/**
* Constructs a new instance of this class given only the style
* value describing its behavior and appearance. This is equivalent
* to calling Shell((Display) null, style)
.
*
* The style value is either one of the style constants defined in
* class SWT
which is applicable to instances of this
* class, or must be built by bitwise OR'ing together
* (that is, using the int
"|" operator) two or more
* of those SWT
style constants. The class description
* lists the style constants that are applicable to the class.
* Style bits are also inherited from superclasses.
*
*
* @param style the style of control to construct
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*
* @see SWT#BORDER
* @see SWT#CLOSE
* @see SWT#MIN
* @see SWT#MAX
* @see SWT#RESIZE
* @see SWT#TITLE
* @see SWT#TOOL
* @see SWT#NO_TRIM
* @see SWT#SHELL_TRIM
* @see SWT#DIALOG_TRIM
* @see SWT#ON_TOP
* @see SWT#MODELESS
* @see SWT#PRIMARY_MODAL
* @see SWT#APPLICATION_MODAL
* @see SWT#SYSTEM_MODAL
* @see SWT#SHEET
*/
public Shell (int style) {
this ((Display) null, style);
}
/**
* Constructs a new instance of this class given only the display
* to create it on. It is created with style SWT.SHELL_TRIM
.
*
* Note: Currently, null can be passed in for the display argument.
* This has the effect of creating the shell on the currently active
* display if there is one. If there is no current display, the
* shell is created on a "default" display. Passing in null as
* the display argument is not considered to be good coding style,
* and may not be supported in a future release of SWT.
*
*
* @param display the display to create the shell on
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*/
public Shell (Display display) {
this (display, SWT.SHELL_TRIM);
}
/**
* Constructs a new instance of this class given the display
* to create it on and a style value describing its behavior
* and appearance.
*
* The style value is either one of the style constants defined in
* class SWT
which is applicable to instances of this
* class, or must be built by bitwise OR'ing together
* (that is, using the int
"|" operator) two or more
* of those SWT
style constants. The class description
* lists the style constants that are applicable to the class.
* Style bits are also inherited from superclasses.
*
* Note: Currently, null can be passed in for the display argument.
* This has the effect of creating the shell on the currently active
* display if there is one. If there is no current display, the
* shell is created on a "default" display. Passing in null as
* the display argument is not considered to be good coding style,
* and may not be supported in a future release of SWT.
*
*
* @param display the display to create the shell on
* @param style the style of control to construct
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*
* @see SWT#BORDER
* @see SWT#CLOSE
* @see SWT#MIN
* @see SWT#MAX
* @see SWT#RESIZE
* @see SWT#TITLE
* @see SWT#TOOL
* @see SWT#NO_TRIM
* @see SWT#SHELL_TRIM
* @see SWT#DIALOG_TRIM
* @see SWT#ON_TOP
* @see SWT#MODELESS
* @see SWT#PRIMARY_MODAL
* @see SWT#APPLICATION_MODAL
* @see SWT#SYSTEM_MODAL
* @see SWT#SHEET
*/
public Shell (Display display, int style) {
this (display, null, style, 0, false);
}
Shell (Display display, Shell parent, int style, long /*int*/ handle, boolean embedded) {
super ();
checkSubclass ();
if (display == null) display = Display.getCurrent ();
if (display == null) display = Display.getDefault ();
if (!display.isValidThread ()) {
error (SWT.ERROR_THREAD_INVALID_ACCESS);
}
if (parent != null && parent.isDisposed ()) {
error (SWT.ERROR_INVALID_ARGUMENT);
}
this.center = parent != null && (style & SWT.SHEET) != 0;
this.style = checkStyle (parent, style);
this.parent = parent;
this.display = display;
if (handle != 0) {
if (embedded) {
this.handle = handle;
} else {
shellHandle = handle;
state |= FOREIGN_HANDLE;
}
}
reskinWidget();
createWidget (0);
}
/**
* Constructs a new instance of this class given only its
* parent. It is created with style SWT.DIALOG_TRIM
.
*
* Note: Currently, null can be passed in for the parent.
* This has the effect of creating the shell on the currently active
* display if there is one. If there is no current display, the
* shell is created on a "default" display. Passing in null as
* the parent is not considered to be good coding style,
* and may not be supported in a future release of SWT.
*
*
* @param parent a shell which will be the parent of the new instance
*
* @exception IllegalArgumentException
* - ERROR_INVALID_ARGUMENT - if the parent is disposed
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*/
public Shell (Shell parent) {
this (parent, SWT.DIALOG_TRIM);
}
/**
* Constructs a new instance of this class given its parent
* and a style value describing its behavior and appearance.
*
* The style value is either one of the style constants defined in
* class SWT
which is applicable to instances of this
* class, or must be built by bitwise OR'ing together
* (that is, using the int
"|" operator) two or more
* of those SWT
style constants. The class description
* lists the style constants that are applicable to the class.
* Style bits are also inherited from superclasses.
*
* Note: Currently, null can be passed in for the parent.
* This has the effect of creating the shell on the currently active
* display if there is one. If there is no current display, the
* shell is created on a "default" display. Passing in null as
* the parent is not considered to be good coding style,
* and may not be supported in a future release of SWT.
*
*
* @param parent a shell which will be the parent of the new instance
* @param style the style of control to construct
*
* @exception IllegalArgumentException
* - ERROR_INVALID_ARGUMENT - if the parent is disposed
*
* @exception SWTException
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
* - ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*
*
* @see SWT#BORDER
* @see SWT#CLOSE
* @see SWT#MIN
* @see SWT#MAX
* @see SWT#RESIZE
* @see SWT#TITLE
* @see SWT#NO_TRIM
* @see SWT#SHELL_TRIM
* @see SWT#DIALOG_TRIM
* @see SWT#ON_TOP
* @see SWT#TOOL
* @see SWT#MODELESS
* @see SWT#PRIMARY_MODAL
* @see SWT#APPLICATION_MODAL
* @see SWT#SYSTEM_MODAL
* @see SWT#SHEET
*/
public Shell (Shell parent, int style) {
this (parent != null ? parent.display : null, parent, style, 0, false);
}
/**
* Invokes platform specific functionality to allocate a new shell
* that is embedded.
*
* IMPORTANT: This method is not part of the public
* API for Shell
. It is marked public only so that it
* can be shared within the packages provided by SWT. It is not
* available on all platforms, and should never be called from
* application code.
*
*
* @param display the display for the shell
* @param handle the handle for the shell
* @return a new shell object containing the specified display and handle
*
* @noreference This method is not intended to be referenced by clients.
*/
public static Shell gtk_new (Display display, long /*int*/ handle) {
return new Shell (display, null, SWT.NO_TRIM, handle, true);
}
/**
* Invokes platform specific functionality to allocate a new shell
* that is not embedded.
*
* IMPORTANT: This method is not part of the public
* API for Shell
. It is marked public only so that it
* can be shared within the packages provided by SWT. It is not
* available on all platforms, and should never be called from
* application code.
*
*
* @param display the display for the shell
* @param handle the handle for the shell
* @return a new shell object containing the specified display and handle
*
* @noreference This method is not intended to be referenced by clients.
*
* @since 3.3
*/
public static Shell internal_new (Display display, long /*int*/ handle) {
return new Shell (display, null, SWT.NO_TRIM, handle, false);
}
static int checkStyle (Shell parent, int style) {
style = Decorations.checkStyle (style);
style &= ~SWT.TRANSPARENT;
if ((style & SWT.ON_TOP) != 0) style &= ~(SWT.CLOSE | SWT.TITLE | SWT.MIN | SWT.MAX);
int mask = SWT.SYSTEM_MODAL | SWT.APPLICATION_MODAL | SWT.PRIMARY_MODAL;
if ((style & SWT.SHEET) != 0) {
style &= ~SWT.SHEET;
style |= parent == null ? SWT.SHELL_TRIM : SWT.DIALOG_TRIM;
if ((style & mask) == 0) {
style |= parent == null ? SWT.APPLICATION_MODAL : SWT.PRIMARY_MODAL;
}
}
int bits = style & ~mask;
if ((style & SWT.SYSTEM_MODAL) != 0) return bits | SWT.SYSTEM_MODAL;
if ((style & SWT.APPLICATION_MODAL) != 0) return bits | SWT.APPLICATION_MODAL;
if ((style & SWT.PRIMARY_MODAL) != 0) return bits | SWT.PRIMARY_MODAL;
return bits;
}
/**
* Adds the listener to the collection of listeners who will
* be notified when operations are performed on the receiver,
* by sending the listener one of the messages defined in the
* ShellListener
interface.
*
* @param listener the listener which should be notified
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if the listener is null
*
* @exception SWTException
* - ERROR_WIDGET_DISPOSED - if the receiver has been disposed
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*
*
* @see ShellListener
* @see #removeShellListener
*/
public void addShellListener (ShellListener listener) {
checkWidget();
if (listener == null) error (SWT.ERROR_NULL_ARGUMENT);
TypedListener typedListener = new TypedListener (listener);
addListener (SWT.Close,typedListener);
addListener (SWT.Iconify,typedListener);
addListener (SWT.Deiconify,typedListener);
addListener (SWT.Activate, typedListener);
addListener (SWT.Deactivate, typedListener);
}
void addToolTip (ToolTip toolTip) {
if (toolTips == null) toolTips = new ToolTip [4];
for (int i=0; i MAXIMUM_TRIM || trimHeight > MAXIMUM_TRIM) {
display.ignoreTrim = true;
return;
}
boolean hasTitle = false, hasResize = false, hasBorder = false;
if ((style & SWT.NO_TRIM) == 0) {
hasTitle = (style & (SWT.MIN | SWT.MAX | SWT.TITLE | SWT.MENU)) != 0;
hasResize = (style & SWT.RESIZE) != 0;
hasBorder = (style & SWT.BORDER) != 0;
}
if (hasTitle) {
if (hasResize) {
display.titleResizeTrimWidth = trimWidth;
display.titleResizeTrimHeight = trimHeight;
return;
}
if (hasBorder) {
display.titleBorderTrimWidth = trimWidth;
display.titleBorderTrimHeight = trimHeight;
return;
}
display.titleTrimWidth = trimWidth;
display.titleTrimHeight = trimHeight;
return;
}
if (hasResize) {
display.resizeTrimWidth = trimWidth;
display.resizeTrimHeight = trimHeight;
return;
}
if (hasBorder) {
display.borderTrimWidth = trimWidth;
display.borderTrimHeight = trimHeight;
return;
}
}
void bringToTop (boolean force) {
if (!gtk_widget_get_visible (shellHandle)) return;
Display display = this.display;
Shell activeShell = display.activeShell;
if (activeShell == this) return;
if (!force) {
if (activeShell == null) return;
if (!display.activePending) {
long /*int*/ focusHandle = OS.gtk_window_get_focus (activeShell.shellHandle);
if (focusHandle != 0 && !gtk_widget_has_focus (focusHandle)) return;
}
}
/*
* Bug in GTK. When a shell that is not managed by the window
* manage is given focus, GTK gets stuck in "focus follows pointer"
* mode when the pointer is within the shell and its parent when
* the shell is hidden or disposed. The fix is to use XSetInputFocus()
* to assign focus when ever the active shell has not managed by
* the window manager.
*
* NOTE: This bug is fixed in GTK+ 2.6.8 and above.
*/
boolean xFocus = false;
if (activeShell != null) {
if (OS.GTK_VERSION < OS.VERSION (2, 6, 8)) {
xFocus = activeShell.isUndecorated ();
}
display.activeShell = null;
display.activePending = true;
}
/*
* Feature in GTK. When the shell is an override redirect
* window, gdk_window_focus() does not give focus to the
* window. The fix is to use XSetInputFocus() to force
* the focus.
*/
long /*int*/ window = gtk_widget_get_window (shellHandle);
if ((xFocus || (style & SWT.ON_TOP) != 0) && OS.GDK_WINDOWING_X11 ()) {
long /*int*/ xDisplay;
if (OS.GTK_VERSION >= OS.VERSION(2, 24, 0)) {
xDisplay = OS.gdk_x11_display_get_xdisplay(OS.gdk_window_get_display(window));
} else {
xDisplay = OS.gdk_x11_drawable_get_xdisplay (window);
}
long /*int*/ xWindow;
if (OS.GTK3) {
xWindow = OS.gdk_x11_window_get_xid (window);
} else {
xWindow = OS.gdk_x11_drawable_get_xid (window);
}
OS.gdk_error_trap_push ();
/* Use CurrentTime instead of the last event time to ensure that the shell becomes active */
OS.XSetInputFocus (xDisplay, xWindow, OS.RevertToParent, OS.CurrentTime);
OS.gdk_error_trap_pop ();
} else {
/*
* Bug in metacity. Calling gdk_window_focus() with a timestamp more
* recent than the last user interaction time can cause windows not
* to come forward in versions > 2.10.0. The fix is to use the last
* user event time.
*/
if (display.windowManager.toLowerCase ().equals ("metacity")) {
OS.gdk_window_focus (window, display.lastUserEventTime);
} else {
OS.gdk_window_focus (window, OS.GDK_CURRENT_TIME);
}
}
display.activeShell = this;
display.activePending = true;
}
void center () {
if (parent == null) return;
Rectangle rect = getBounds ();
Rectangle parentRect = display.map (parent, null, parent.getClientArea());
int x = Math.max (parentRect.x, parentRect.x + (parentRect.width - rect.width) / 2);
int y = Math.max (parentRect.y, parentRect.y + (parentRect.height - rect.height) / 2);
Rectangle monitorRect = parent.getMonitor ().getClientArea();
if (x + rect.width > monitorRect.x + monitorRect.width) {
x = Math.max (monitorRect.x, monitorRect.x + monitorRect.width - rect.width);
} else {
x = Math.max (x, monitorRect.x);
}
if (y + rect.height > monitorRect.y + monitorRect.height) {
y = Math.max (monitorRect.y, monitorRect.y + monitorRect.height - rect.height);
} else {
y = Math.max (y, monitorRect.y);
}
setLocation (x, y);
}
void checkBorder () {
/* Do nothing */
}
void checkOpen () {
if (!opened) resized = false;
}
long /*int*/ childStyle () {
return 0;
}
/**
* Requests that the window manager close the receiver in
* the same way it would be closed when the user clicks on
* the "close box" or performs some other platform specific
* key or mouse combination that indicates the window
* should be removed.
*
* @exception SWTException
* - ERROR_WIDGET_DISPOSED - if the receiver has been disposed
* - ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*
*
* @see SWT#Close
* @see #dispose
*/
public void close () {
checkWidget ();
closeWidget ();
}
void closeWidget () {
Event event = new Event ();
sendEvent (SWT.Close, event);
if (event.doit && !isDisposed ()) dispose ();
}
public Rectangle computeTrim (int x, int y, int width, int height) {
checkWidget();
Rectangle trim = super.computeTrim (x, y, width, height);
int border = 0;
if ((style & (SWT.NO_TRIM | SWT.BORDER | SWT.SHELL_TRIM)) == 0) {
border = OS.gtk_container_get_border_width (shellHandle);
}
if (isCustomResize ()) {
border = OS.gtk_container_get_border_width (shellHandle);
}
int trimWidth = trimWidth (), trimHeight = trimHeight ();
trim.x -= (trimWidth / 2) + border;
trim.y -= trimHeight - (trimWidth / 2) + border;
trim.width += trimWidth + border * 2;
trim.height += trimHeight + border * 2;
if (menuBar != null) {
forceResize ();
GtkAllocation allocation = new GtkAllocation ();
gtk_widget_get_allocation (menuBar.handle, allocation);
int menuBarHeight = allocation.height;
trim.y -= menuBarHeight;
trim.height += menuBarHeight;
}
return trim;
}
void createHandle (int index) {
state |= HANDLE | CANVAS;
if (shellHandle == 0) {
if (handle == 0) {
int type = OS.GTK_WINDOW_TOPLEVEL;
if ((style & SWT.ON_TOP) != 0) type = OS.GTK_WINDOW_POPUP;
shellHandle = OS.gtk_window_new (type);
} else {
shellHandle = OS.gtk_plug_new (handle);
}
if (shellHandle == 0) error (SWT.ERROR_NO_HANDLES);
if (parent != null) {
OS.gtk_window_set_transient_for (shellHandle, parent.topHandle ());
OS.gtk_window_set_destroy_with_parent (shellHandle, true);
if (!isUndecorated ()) {
OS.gtk_window_set_type_hint (shellHandle, OS.GDK_WINDOW_TYPE_HINT_DIALOG);
} else {
OS.gtk_window_set_skip_taskbar_hint (shellHandle, true);
}
}
/*
* Feature in GTK. The window size must be set when the window
* is created or it will not be allowed to be resized smaller that the
* initial size by the user. The fix is to set the size to zero.
*/
if ((style & SWT.RESIZE) != 0) {
OS.gtk_widget_set_size_request (shellHandle, 0, 0);
OS.gtk_window_set_resizable (shellHandle, true);
} else {
OS.gtk_window_set_resizable (shellHandle, false);
}
OS.gtk_window_set_title (shellHandle, new byte [1]);
if ((style & (SWT.NO_TRIM | SWT.BORDER | SWT.SHELL_TRIM)) == 0) {
OS.gtk_container_set_border_width (shellHandle, 1);
if (OS.GTK3) {
OS.gtk_widget_override_background_color (shellHandle, OS.GTK_STATE_FLAG_NORMAL, new GdkRGBA());
} else {
GdkColor color = new GdkColor ();
OS.gtk_style_get_black (OS.gtk_widget_get_style (shellHandle), color);
OS.gtk_widget_modify_bg (shellHandle, OS.GTK_STATE_NORMAL, color);
}
}
if (isCustomResize ()) {
OS.gtk_container_set_border_width (shellHandle, BORDER);
}
}
vboxHandle = gtk_box_new (OS.GTK_ORIENTATION_VERTICAL, false, 0);
if (vboxHandle == 0) error (SWT.ERROR_NO_HANDLES);
createHandle (index, false, true);
OS.gtk_container_add (vboxHandle, scrolledHandle);
OS.gtk_box_set_child_packing (vboxHandle, scrolledHandle, true, true, 0, OS.GTK_PACK_END);
group = OS.gtk_window_group_new ();
if (group == 0) error (SWT.ERROR_NO_HANDLES);
/*
* Feature in GTK. Realizing the shell triggers a size allocate event,
* which may be confused for a resize event from the window manager if
* received too late. The fix is to realize the window during creation
* to avoid confusion.
*/
OS.gtk_widget_realize (shellHandle);
}
long /*int*/ filterProc (long /*int*/ xEvent, long /*int*/ gdkEvent, long /*int*/ data2) {
int eventType = OS.X_EVENT_TYPE (xEvent);
if (eventType != OS.FocusOut && eventType != OS.FocusIn) return 0;
XFocusChangeEvent xFocusEvent = new XFocusChangeEvent();
OS.memmove (xFocusEvent, xEvent, XFocusChangeEvent.sizeof);
switch (eventType) {
case OS.FocusIn:
if (xFocusEvent.mode == OS.NotifyNormal || xFocusEvent.mode == OS.NotifyWhileGrabbed) {
switch (xFocusEvent.detail) {
case OS.NotifyNonlinear:
case OS.NotifyNonlinearVirtual:
case OS.NotifyAncestor:
if (tooltipsHandle != 0 && OS.GTK_VERSION < OS.VERSION (2, 12, 0)) {
OS.gtk_tooltips_enable (tooltipsHandle);
}
display.activeShell = this;
display.activePending = false;
sendEvent (SWT.Activate);
if (isDisposed ()) return 0;
if (isCustomResize ()) {
OS.gdk_window_invalidate_rect (gtk_widget_get_window (shellHandle), null, false);
}
break;
}
}
break;
case OS.FocusOut:
if (xFocusEvent.mode == OS.NotifyNormal || xFocusEvent.mode == OS.NotifyWhileGrabbed) {
switch (xFocusEvent.detail) {
case OS.NotifyNonlinear:
case OS.NotifyNonlinearVirtual:
case OS.NotifyVirtual:
if (tooltipsHandle != 0 && OS.GTK_VERSION < OS.VERSION (2, 12, 0)) {
OS.gtk_tooltips_disable (tooltipsHandle);
}
Display display = this.display;
sendEvent (SWT.Deactivate);
setActiveControl (null);
if (display.activeShell == this) {
display.activeShell = null;
display.activePending = false;
}
if (isDisposed ()) return 0;
if (isCustomResize ()) {
OS.gdk_window_invalidate_rect (gtk_widget_get_window (shellHandle), null, false);
}
break;
}
}
break;
}
return 0;
}
Control findBackgroundControl () {
return (state & BACKGROUND) != 0 || backgroundImage != null ? this : null;
}
Composite findDeferredControl () {
return layoutCount > 0 ? this : null;
}
/**
* Returns a ToolBar object representing the tool bar that can be shown in the receiver's
* trim. This will return null
if the platform does not support tool bars that
* are not part of the content area of the shell, or if the Shell's style does not support
* having a tool bar.
*
*
* @return a ToolBar object representing the Shell's tool bar, or null
.
*
* @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
if the receiver is currently
* in fullscreen state, and false otherwise.
* * * @return the fullscreen state * * @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
if the receiver is marked as modified, or false
otherwise
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
null
if the shell has the default shape.
*
* @return the region that defines the shape of the shell, or null
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
SWT
:
* NONE
, ROMAN
, DBCS
,
* PHONETIC
, NATIVE
, ALPHA
.
*
* @return the IME mode
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* @return the dialog shells * * @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_NULL_ARGUMENT - if the listener is null *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* This operation requires the operating system's advanced * widgets subsystem which may not be available on some * platforms. *
* @param alpha the alpha value * * @exception SWTException-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
causes the receiver
* to switch to the full screen state, and if the argument is
* false
and the receiver was previously switched
* into full screen state, causes the receiver to switch back
* to either the maximized or normal states.
*
* Note: The result of intermixing calls to setFullScreen(true)
,
* setMaximized(true)
and setMinimized(true)
will
* vary by platform. Typically, the behavior will match the platform user's
* expectations, but not always. This should be avoided if possible.
*
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
SWT
:
* NONE
, ROMAN
, DBCS
,
* PHONETIC
, NATIVE
, ALPHA
.
*
* @param mode the new IME mode
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_NULL_ARGUMENT - if the point is null *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_INVALID_ARGUMENT - if the region has been disposed *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *