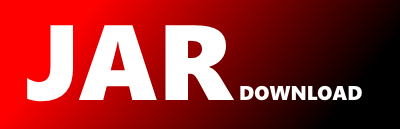
org.eclipse.sisu.equinox.launching.internal.EquinoxLaunchConfiguration Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2008, 2015 Sonatype Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Sonatype Inc. - initial API and implementation
*******************************************************************************/
package org.eclipse.sisu.equinox.launching.internal;
import java.io.File;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.codehaus.plexus.util.cli.Commandline.Argument;
import org.eclipse.sisu.equinox.launching.EquinoxInstallation;
import org.eclipse.tycho.launching.LaunchConfiguration;
public class EquinoxLaunchConfiguration implements LaunchConfiguration {
private String jvmExecutable;
private File workingDirectory;
private final Map env = new LinkedHashMap<>();
private final List args = new ArrayList<>();
private final List vmargs = new ArrayList<>();
private final EquinoxInstallation installation;
public EquinoxLaunchConfiguration(EquinoxInstallation installation) {
this.installation = installation;
}
public void addEnvironmentVariables(Map variables) {
for (String key : variables.keySet()) {
String value = variables.get(key);
env.put(key, (value != null) ? value : "");
}
}
@Override
public Map getEnvironment() {
return env;
}
public void setJvmExecutable(String jvmExecutable) {
this.jvmExecutable = jvmExecutable;
}
@Override
public String getJvmExecutable() {
return jvmExecutable;
}
public void setWorkingDirectory(File workingDirectory) {
this.workingDirectory = workingDirectory;
}
@Override
public File getWorkingDirectory() {
return workingDirectory;
}
public void addProgramArguments(String... args) {
addArguments(this.args, args);
}
private void addArguments(List to, String... args) {
for (String str : args) {
Argument arg = new Argument();
arg.setValue(str);
to.add(arg);
}
}
@Override
public String[] getProgramArguments() {
return toStringArray(args);
}
private static String[] toStringArray(List args) {
ArrayList result = new ArrayList<>();
for (Argument arg : args) {
for (String str : arg.getParts()) {
result.add(str);
}
}
return result.toArray(new String[result.size()]);
}
public void addVMArguments(String... vmargs) {
addArguments(this.vmargs, vmargs);
}
@Override
public String[] getVMArguments() {
return toStringArray(vmargs);
}
@Override
public File getLauncherJar() {
return installation.getLauncherJar();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy