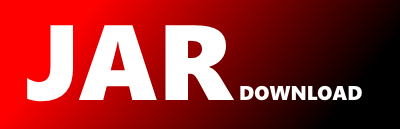
org.eclipse.uml2.uml.Interface Maven / Gradle / Ivy
/*
* Copyright (c) 2005, 2007 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM - initial API and implementation
*
* $Id: Interface.java,v 1.14 2007/10/23 15:54:21 jbruck Exp $
*/
package org.eclipse.uml2.uml;
import java.util.Map;
import org.eclipse.emf.common.util.DiagnosticChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* A representation of the model object 'Interface'.
*
*
*
* An interface is a kind of classifier that represents a declaration of a set of coherent public features and obligations. An interface specifies a contract; any instance of a classifier that realizes the interface must fulfill that contract. The obligations that may be associated with an interface are in the form of various kinds of constraints (such as pre- and post-conditions) or protocol specifications, which may impose ordering restrictions on interactions through the interface.
* Interfaces may include receptions (in addition to operations).
* Since an interface specifies conformance characteristics, it does not own detailed behavior specifications. Instead, interfaces may own a protocol state machine that specifies event sequences and pre/post conditions for the operations and receptions described by the interface.
*
*
*
* The following features are supported:
*
* - {@link org.eclipse.uml2.uml.Interface#getOwnedAttributes Owned Attribute}
* - {@link org.eclipse.uml2.uml.Interface#getOwnedOperations Owned Operation}
* - {@link org.eclipse.uml2.uml.Interface#getNestedClassifiers Nested Classifier}
* - {@link org.eclipse.uml2.uml.Interface#getRedefinedInterfaces Redefined Interface}
* - {@link org.eclipse.uml2.uml.Interface#getOwnedReceptions Owned Reception}
* - {@link org.eclipse.uml2.uml.Interface#getProtocol Protocol}
*
*
*
* @see org.eclipse.uml2.uml.UMLPackage#getInterface()
* @model
* @generated
*/
public interface Interface
extends Classifier {
/**
* Returns the value of the 'Owned Attribute' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Property}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Classifier#getAttributes() Attribute}'
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* The attributes (i.e. the properties) owned by the class.
*
* @return the value of the 'Owned Attribute' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_OwnedAttribute()
* @model containment="true" resolveProxies="true"
* @generated
*/
EList getOwnedAttributes();
/**
* Creates a new {@link org.eclipse.uml2.uml.Property}, with the specified 'Name', and 'Type', and appends it to the 'Owned Attribute' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Property}, or null
.
* @param type The 'Type' for the new {@link org.eclipse.uml2.uml.Property}, or null
.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Property} to create.
* @return The new {@link org.eclipse.uml2.uml.Property}.
* @see #getOwnedAttributes()
* @generated
*/
Property createOwnedAttribute(String name, Type type, EClass eClass);
/**
* Creates a new {@link org.eclipse.uml2.uml.Property}, with the specified 'Name', and 'Type', and appends it to the 'Owned Attribute' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Property}, or null
.
* @param type The 'Type' for the new {@link org.eclipse.uml2.uml.Property}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Property}.
* @see #getOwnedAttributes()
* @generated
*/
Property createOwnedAttribute(String name, Type type);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Property} with the specified 'Name', and 'Type' from the 'Owned Attribute' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Property} to retrieve, or null
.
* @param type The 'Type' of the {@link org.eclipse.uml2.uml.Property} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Property} with the specified 'Name', and 'Type', or null
.
* @see #getOwnedAttributes()
* @generated
*/
Property getOwnedAttribute(String name, Type type);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Property} with the specified 'Name', and 'Type' from the 'Owned Attribute' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Property} to retrieve, or null
.
* @param type The 'Type' of the {@link org.eclipse.uml2.uml.Property} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Property} to retrieve, or null
.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Property} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Property} with the specified 'Name', and 'Type', or null
.
* @see #getOwnedAttributes()
* @generated
*/
Property getOwnedAttribute(String name, Type type, boolean ignoreCase,
EClass eClass, boolean createOnDemand);
/**
* Returns the value of the 'Nested Classifier' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Classifier}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* References all the Classifiers that are defined (nested) within the Class.
*
* @return the value of the 'Nested Classifier' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_NestedClassifier()
* @model containment="true" resolveProxies="true"
* @generated
*/
EList getNestedClassifiers();
/**
* Creates a new {@link org.eclipse.uml2.uml.Classifier}, with the specified 'Name', and appends it to the 'Nested Classifier' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Classifier}, or null
.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Classifier} to create.
* @return The new {@link org.eclipse.uml2.uml.Classifier}.
* @see #getNestedClassifiers()
* @generated
*/
Classifier createNestedClassifier(String name, EClass eClass);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Classifier} with the specified 'Name' from the 'Nested Classifier' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Classifier} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Classifier} with the specified 'Name', or null
.
* @see #getNestedClassifiers()
* @generated
*/
Classifier getNestedClassifier(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Classifier} with the specified 'Name' from the 'Nested Classifier' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Classifier} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Classifier} to retrieve, or null
.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Classifier} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Classifier} with the specified 'Name', or null
.
* @see #getNestedClassifiers()
* @generated
*/
Classifier getNestedClassifier(String name, boolean ignoreCase,
EClass eClass, boolean createOnDemand);
/**
* Returns the value of the 'Redefined Interface' reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Interface}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.RedefinableElement#getRedefinedElements() Redefined Element}'
*
*
*
*
*
* References all the Interfaces redefined by this Interface.
*
* @return the value of the 'Redefined Interface' reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_RedefinedInterface()
* @model ordered="false"
* @generated
*/
EList getRedefinedInterfaces();
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Interface} with the specified 'Name' from the 'Redefined Interface' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Interface} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Interface} with the specified 'Name', or null
.
* @see #getRedefinedInterfaces()
* @generated
*/
Interface getRedefinedInterface(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Interface} with the specified 'Name' from the 'Redefined Interface' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Interface} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @return The first {@link org.eclipse.uml2.uml.Interface} with the specified 'Name', or null
.
* @see #getRedefinedInterfaces()
* @generated
*/
Interface getRedefinedInterface(String name, boolean ignoreCase);
/**
* Returns the value of the 'Owned Reception' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Reception}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Classifier#getFeatures() Feature}'
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* Receptions that objects providing this interface are willing to accept.
*
* @return the value of the 'Owned Reception' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_OwnedReception()
* @model containment="true" resolveProxies="true" ordered="false"
* @generated
*/
EList getOwnedReceptions();
/**
* Creates a new {@link org.eclipse.uml2.uml.Reception}, with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', and appends it to the 'Owned Reception' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Reception}, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' for the new {@link org.eclipse.uml2.uml.Reception}, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' for the new {@link org.eclipse.uml2.uml.Reception}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Reception}.
* @see #getOwnedReceptions()
* @generated
*/
Reception createOwnedReception(String name,
EList ownedParameterNames, EList ownedParameterTypes);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Reception} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types' from the 'Owned Reception' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Reception} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', or null
.
* @see #getOwnedReceptions()
* @generated
*/
Reception getOwnedReception(String name, EList ownedParameterNames,
EList ownedParameterTypes);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Reception} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types' from the 'Owned Reception' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' of the {@link org.eclipse.uml2.uml.Reception} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Reception} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Reception} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', or null
.
* @see #getOwnedReceptions()
* @generated
*/
Reception getOwnedReception(String name, EList ownedParameterNames,
EList ownedParameterTypes, boolean ignoreCase,
boolean createOnDemand);
/**
* Returns the value of the 'Protocol' containment reference.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* References a protocol state machine specifying the legal sequences of the invocation of the behavioral features described in the interface.
*
* @return the value of the 'Protocol' containment reference.
* @see #setProtocol(ProtocolStateMachine)
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_Protocol()
* @model containment="true" resolveProxies="true" ordered="false"
* @generated
*/
ProtocolStateMachine getProtocol();
/**
* Sets the value of the '{@link org.eclipse.uml2.uml.Interface#getProtocol Protocol}' containment reference.
*
*
* @param value the new value of the 'Protocol' containment reference.
* @see #getProtocol()
* @generated
*/
void setProtocol(ProtocolStateMachine value);
/**
* Creates a new {@link org.eclipse.uml2.uml.ProtocolStateMachine},with the specified 'Name', and sets the 'Protocol' containment reference.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.ProtocolStateMachine}, or null
.
* @return The new {@link org.eclipse.uml2.uml.ProtocolStateMachine}.
* @see #getProtocol()
* @generated
*/
ProtocolStateMachine createProtocol(String name);
/**
* Returns the value of the 'Owned Operation' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Operation}.
* It is bidirectional and its opposite is '{@link org.eclipse.uml2.uml.Operation#getInterface Interface}'.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Classifier#getFeatures() Feature}'
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* The operations owned by the class.
*
* @return the value of the 'Owned Operation' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getInterface_OwnedOperation()
* @see org.eclipse.uml2.uml.Operation#getInterface
* @model opposite="interface" containment="true" resolveProxies="true"
* @generated
*/
EList getOwnedOperations();
/**
* Creates a new {@link org.eclipse.uml2.uml.Operation}, with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', and appends it to the 'Owned Operation' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Operation}, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' for the new {@link org.eclipse.uml2.uml.Operation}, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' for the new {@link org.eclipse.uml2.uml.Operation}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Operation}.
* @see #getOwnedOperations()
* @generated
*/
Operation createOwnedOperation(String name,
EList ownedParameterNames, EList ownedParameterTypes);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Operation} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types' from the 'Owned Operation' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Operation} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', or null
.
* @see #getOwnedOperations()
* @generated
*/
Operation getOwnedOperation(String name, EList ownedParameterNames,
EList ownedParameterTypes);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Operation} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types' from the 'Owned Operation' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @param ownedParameterNames The 'Owned Parameter Names' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @param ownedParameterTypes The 'Owned Parameter Types' of the {@link org.eclipse.uml2.uml.Operation} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Operation} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Operation} with the specified 'Name', 'Owned Parameter Names', and 'Owned Parameter Types', or null
.
* @see #getOwnedOperations()
* @generated
*/
Operation getOwnedOperation(String name, EList ownedParameterNames,
EList ownedParameterTypes, boolean ignoreCase,
boolean createOnDemand);
/**
*
*
*
* The visibility of all features owned by an interface must be public.
* self.feature->forAll(f | f.visibility = #public)
* @param diagnostics The chain of diagnostics to which problems are to be appended.
* @param context The cache of context-specific information.
*
* @model
* @generated
*/
boolean validateVisibility(DiagnosticChain diagnostics,
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy