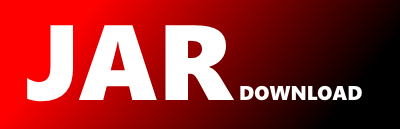
org.eclipse.uml2.uml.Node Maven / Gradle / Ivy
/*
* Copyright (c) 2005, 2009 IBM Corporation, Embarcadero Technologies, and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM - initial API and implementation
* Kenn Hussey (Embarcadero Technologies) - 205188
*
* $Id: Node.java,v 1.13 2009/08/12 21:05:19 jbruck Exp $
*/
package org.eclipse.uml2.uml;
import java.util.Map;
import org.eclipse.emf.common.util.DiagnosticChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* A representation of the model object 'Node'.
*
*
*
* A node is computational resource upon which artifacts may be deployed for execution.
* Nodes can be interconnected through communication paths to define network structures.
*
*
*
* The following features are supported:
*
* - {@link org.eclipse.uml2.uml.Node#getNestedNodes Nested Node}
*
*
*
* @see org.eclipse.uml2.uml.UMLPackage#getNode()
* @model
* @generated
*/
public interface Node
extends org.eclipse.uml2.uml.Class, DeploymentTarget {
/**
* Returns the value of the 'Nested Node' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Node}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* The Nodes that are defined (nested) within the Node.
*
* @return the value of the 'Nested Node' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getNode_NestedNode()
* @model containment="true" resolveProxies="true" ordered="false"
* @generated
*/
EList getNestedNodes();
/**
* Creates a new {@link org.eclipse.uml2.uml.Node}, with the specified 'Name', and appends it to the 'Nested Node' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Node}, or null
.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Node} to create.
* @return The new {@link org.eclipse.uml2.uml.Node}.
* @see #getNestedNodes()
* @generated
*/
Node createNestedNode(String name, EClass eClass);
/**
* Creates a new {@link org.eclipse.uml2.uml.Node}, with the specified 'Name', and appends it to the 'Nested Node' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Node}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Node}.
* @see #getNestedNodes()
* @generated
*/
Node createNestedNode(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Node} with the specified 'Name' from the 'Nested Node' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Node} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Node} with the specified 'Name', or null
.
* @see #getNestedNodes()
* @generated
*/
Node getNestedNode(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Node} with the specified 'Name' from the 'Nested Node' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Node} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.Node} to retrieve, or null
.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Node} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Node} with the specified 'Name', or null
.
* @see #getNestedNodes()
* @generated
*/
Node getNestedNode(String name, boolean ignoreCase, EClass eClass,
boolean createOnDemand);
/**
*
*
*
* The internal structure of a Node (if defined) consists solely of parts of type Node.
* true
* @param diagnostics The chain of diagnostics to which problems are to be appended.
* @param context The cache of context-specific information.
*
* @model
* @generated
*/
boolean validateInternalStructure(DiagnosticChain diagnostics,
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy