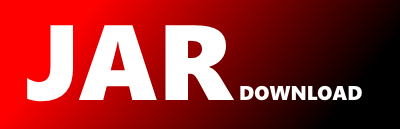
org.eclipse.uml2.uml.StateMachine Maven / Gradle / Ivy
/*
* Copyright (c) 2005, 2007 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM - initial API and implementation
*
* $Id: StateMachine.java,v 1.14 2007/10/23 15:54:22 jbruck Exp $
*/
package org.eclipse.uml2.uml;
import java.util.Map;
import org.eclipse.emf.common.util.DiagnosticChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
/**
*
* A representation of the model object 'State Machine'.
*
*
*
* State machines can be used to express the behavior of part of a system. Behavior is modeled as a traversal of a graph of state nodes interconnected by one or more joined transition arcs that are triggered by the dispatching of series of (event) occurrences. During this traversal, the state machine executes a series of activities associated with various elements of the state machine.
*
*
*
* The following features are supported:
*
* - {@link org.eclipse.uml2.uml.StateMachine#getRegions Region}
* - {@link org.eclipse.uml2.uml.StateMachine#getSubmachineStates Submachine State}
* - {@link org.eclipse.uml2.uml.StateMachine#getConnectionPoints Connection Point}
* - {@link org.eclipse.uml2.uml.StateMachine#getExtendedStateMachines Extended State Machine}
*
*
*
* @see org.eclipse.uml2.uml.UMLPackage#getStateMachine()
* @model
* @generated
*/
public interface StateMachine
extends Behavior {
/**
* Returns the value of the 'Region' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Region}.
* It is bidirectional and its opposite is '{@link org.eclipse.uml2.uml.Region#getStateMachine State Machine}'.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* The regions owned directly by the state machine.
*
* @return the value of the 'Region' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getStateMachine_Region()
* @see org.eclipse.uml2.uml.Region#getStateMachine
* @model opposite="stateMachine" containment="true" resolveProxies="true" required="true" ordered="false"
* @generated
*/
EList getRegions();
/**
* Creates a new {@link org.eclipse.uml2.uml.Region}, with the specified 'Name', and appends it to the 'Region' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Region}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Region}.
* @see #getRegions()
* @generated
*/
Region createRegion(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Region} with the specified 'Name' from the 'Region' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Region} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Region} with the specified 'Name', or null
.
* @see #getRegions()
* @generated
*/
Region getRegion(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Region} with the specified 'Name' from the 'Region' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Region} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Region} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Region} with the specified 'Name', or null
.
* @see #getRegions()
* @generated
*/
Region getRegion(String name, boolean ignoreCase, boolean createOnDemand);
/**
* Returns the value of the 'Submachine State' reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.State}.
* It is bidirectional and its opposite is '{@link org.eclipse.uml2.uml.State#getSubmachine Submachine}'.
*
*
*
* References the submachine(s) in case of a submachine state. Multiple machines are referenced in case of a concurrent state.
*
* @return the value of the 'Submachine State' reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getStateMachine_SubmachineState()
* @see org.eclipse.uml2.uml.State#getSubmachine
* @model opposite="submachine" ordered="false"
* @generated
*/
EList getSubmachineStates();
/**
* Retrieves the first {@link org.eclipse.uml2.uml.State} with the specified 'Name' from the 'Submachine State' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.State} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.State} with the specified 'Name', or null
.
* @see #getSubmachineStates()
* @generated
*/
State getSubmachineState(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.State} with the specified 'Name' from the 'Submachine State' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.State} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.State} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.State} with the specified 'Name', or null
.
* @see #getSubmachineStates()
* @generated
*/
State getSubmachineState(String name, boolean ignoreCase, EClass eClass);
/**
* Returns the value of the 'Connection Point' containment reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.Pseudostate}.
* It is bidirectional and its opposite is '{@link org.eclipse.uml2.uml.Pseudostate#getStateMachine State Machine}'.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.Namespace#getOwnedMembers() Owned Member}'
*
*
*
*
*
* The connection points defined for this state machine. They represent the interface of the state machine when used as part of submachine state.
*
* @return the value of the 'Connection Point' containment reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getStateMachine_ConnectionPoint()
* @see org.eclipse.uml2.uml.Pseudostate#getStateMachine
* @model opposite="stateMachine" containment="true" resolveProxies="true" ordered="false"
* @generated
*/
EList getConnectionPoints();
/**
* Creates a new {@link org.eclipse.uml2.uml.Pseudostate}, with the specified 'Name', and appends it to the 'Connection Point' containment reference list.
*
*
* @param name The 'Name' for the new {@link org.eclipse.uml2.uml.Pseudostate}, or null
.
* @return The new {@link org.eclipse.uml2.uml.Pseudostate}.
* @see #getConnectionPoints()
* @generated
*/
Pseudostate createConnectionPoint(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Pseudostate} with the specified 'Name' from the 'Connection Point' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Pseudostate} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.Pseudostate} with the specified 'Name', or null
.
* @see #getConnectionPoints()
* @generated
*/
Pseudostate getConnectionPoint(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.Pseudostate} with the specified 'Name' from the 'Connection Point' containment reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.Pseudostate} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param createOnDemand Whether to create a {@link org.eclipse.uml2.uml.Pseudostate} on demand if not found.
* @return The first {@link org.eclipse.uml2.uml.Pseudostate} with the specified 'Name', or null
.
* @see #getConnectionPoints()
* @generated
*/
Pseudostate getConnectionPoint(String name, boolean ignoreCase,
boolean createOnDemand);
/**
* Returns the value of the 'Extended State Machine' reference list.
* The list contents are of type {@link org.eclipse.uml2.uml.StateMachine}.
*
* This feature subsets the following features:
*
* - '{@link org.eclipse.uml2.uml.RedefinableElement#getRedefinedElements() Redefined Element}'
*
*
*
*
*
* The state machines of which this is an extension.
*
* @return the value of the 'Extended State Machine' reference list.
* @see org.eclipse.uml2.uml.UMLPackage#getStateMachine_ExtendedStateMachine()
* @model ordered="false"
* @generated
*/
EList getExtendedStateMachines();
/**
* Retrieves the first {@link org.eclipse.uml2.uml.StateMachine} with the specified 'Name' from the 'Extended State Machine' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.StateMachine} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.StateMachine} with the specified 'Name', or null
.
* @see #getExtendedStateMachines()
* @generated
*/
StateMachine getExtendedStateMachine(String name);
/**
* Retrieves the first {@link org.eclipse.uml2.uml.StateMachine} with the specified 'Name' from the 'Extended State Machine' reference list.
*
*
* @param name The 'Name' of the {@link org.eclipse.uml2.uml.StateMachine} to retrieve, or null
.
* @param ignoreCase Whether to ignore case in {@link java.lang.String} comparisons.
* @param eClass The Ecore class of the {@link org.eclipse.uml2.uml.StateMachine} to retrieve, or null
.
* @return The first {@link org.eclipse.uml2.uml.StateMachine} with the specified 'Name', or null
.
* @see #getExtendedStateMachines()
* @generated
*/
StateMachine getExtendedStateMachine(String name, boolean ignoreCase,
EClass eClass);
/**
*
*
*
* The classifier context of a state machine cannot be an interface.
* context->notEmpty() implies not context.oclIsKindOf(Interface)
* @param diagnostics The chain of diagnostics to which problems are to be appended.
* @param context The cache of context-specific information.
*
* @model
* @generated
*/
boolean validateClassifierContext(DiagnosticChain diagnostics,
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy