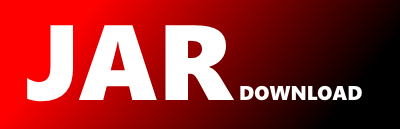
org.eclipse.uml2.uml.UMLFactory Maven / Gradle / Ivy
/*
* Copyright (c) 2005, 2009 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM - initial API and implementation
*
* $Id: UMLFactory.java,v 1.8 2009/02/27 18:37:52 jbruck Exp $
*/
package org.eclipse.uml2.uml;
import org.eclipse.emf.ecore.EFactory;
/**
*
* The Factory for the model.
* It provides a create method for each non-abstract class of the model.
*
* @see org.eclipse.uml2.uml.UMLPackage
* @generated
*/
public interface UMLFactory
extends EFactory {
/**
* The singleton instance of the factory.
*
*
* @generated
*/
UMLFactory eINSTANCE = org.eclipse.uml2.uml.internal.impl.UMLFactoryImpl
.init();
/**
* Returns a new object of class 'Comment'.
*
*
* @return a new object of class 'Comment'.
* @generated
*/
Comment createComment();
/**
* Returns a new object of class 'Dependency'.
*
*
* @return a new object of class 'Dependency'.
* @generated
*/
Dependency createDependency();
/**
* Returns a new object of class 'Template Parameter'.
*
*
* @return a new object of class 'Template Parameter'.
* @generated
*/
TemplateParameter createTemplateParameter();
/**
* Returns a new object of class 'Template Signature'.
*
*
* @return a new object of class 'Template Signature'.
* @generated
*/
TemplateSignature createTemplateSignature();
/**
* Returns a new object of class 'Template Binding'.
*
*
* @return a new object of class 'Template Binding'.
* @generated
*/
TemplateBinding createTemplateBinding();
/**
* Returns a new object of class 'Template Parameter Substitution'.
*
*
* @return a new object of class 'Template Parameter Substitution'.
* @generated
*/
TemplateParameterSubstitution createTemplateParameterSubstitution();
/**
* Returns a new object of class 'Element Import'.
*
*
* @return a new object of class 'Element Import'.
* @generated
*/
ElementImport createElementImport();
/**
* Returns a new object of class 'Package Import'.
*
*
* @return a new object of class 'Package Import'.
* @generated
*/
PackageImport createPackageImport();
/**
* Returns a new object of class 'Package'.
*
*
* @return a new object of class 'Package'.
* @generated
*/
Package createPackage();
/**
* Returns a new object of class 'Package Merge'.
*
*
* @return a new object of class 'Package Merge'.
* @generated
*/
PackageMerge createPackageMerge();
/**
* Returns a new object of class 'Profile Application'.
*
*
* @return a new object of class 'Profile Application'.
* @generated
*/
ProfileApplication createProfileApplication();
/**
* Returns a new object of class 'Profile'.
*
*
* @return a new object of class 'Profile'.
* @generated
*/
Profile createProfile();
/**
* Returns a new object of class 'Stereotype'.
*
*
* @return a new object of class 'Stereotype'.
* @generated
*/
Stereotype createStereotype();
/**
* Returns a new object of class 'Image'.
*
*
* @return a new object of class 'Image'.
* @generated
*/
Image createImage();
/**
* Returns a new object of class 'Class'.
*
*
* @return a new object of class 'Class'.
* @generated
*/
Class createClass();
/**
* Returns a new object of class 'Generalization'.
*
*
* @return a new object of class 'Generalization'.
* @generated
*/
Generalization createGeneralization();
/**
* Returns a new object of class 'Generalization Set'.
*
*
* @return a new object of class 'Generalization Set'.
* @generated
*/
GeneralizationSet createGeneralizationSet();
/**
* Returns a new object of class 'Use Case'.
*
*
* @return a new object of class 'Use Case'.
* @generated
*/
UseCase createUseCase();
/**
* Returns a new object of class 'Include'.
*
*
* @return a new object of class 'Include'.
* @generated
*/
Include createInclude();
/**
* Returns a new object of class 'Extend'.
*
*
* @return a new object of class 'Extend'.
* @generated
*/
Extend createExtend();
/**
* Returns a new object of class 'Constraint'.
*
*
* @return a new object of class 'Constraint'.
* @generated
*/
Constraint createConstraint();
/**
* Returns a new object of class 'Extension Point'.
*
*
* @return a new object of class 'Extension Point'.
* @generated
*/
ExtensionPoint createExtensionPoint();
/**
* Returns a new object of class 'Substitution'.
*
*
* @return a new object of class 'Substitution'.
* @generated
*/
Substitution createSubstitution();
/**
* Returns a new object of class 'Realization'.
*
*
* @return a new object of class 'Realization'.
* @generated
*/
Realization createRealization();
/**
* Returns a new object of class 'Abstraction'.
*
*
* @return a new object of class 'Abstraction'.
* @generated
*/
Abstraction createAbstraction();
/**
* Returns a new object of class 'Opaque Expression'.
*
*
* @return a new object of class 'Opaque Expression'.
* @generated
*/
OpaqueExpression createOpaqueExpression();
/**
* Returns a new object of class 'Parameter'.
*
*
* @return a new object of class 'Parameter'.
* @generated
*/
Parameter createParameter();
/**
* Returns a new object of class 'Connector End'.
*
*
* @return a new object of class 'Connector End'.
* @generated
*/
ConnectorEnd createConnectorEnd();
/**
* Returns a new object of class 'Property'.
*
*
* @return a new object of class 'Property'.
* @generated
*/
Property createProperty();
/**
* Returns a new object of class 'Deployment'.
*
*
* @return a new object of class 'Deployment'.
* @generated
*/
Deployment createDeployment();
/**
* Returns a new object of class 'Deployment Specification'.
*
*
* @return a new object of class 'Deployment Specification'.
* @generated
*/
DeploymentSpecification createDeploymentSpecification();
/**
* Returns a new object of class 'Artifact'.
*
*
* @return a new object of class 'Artifact'.
* @generated
*/
Artifact createArtifact();
/**
* Returns a new object of class 'Manifestation'.
*
*
* @return a new object of class 'Manifestation'.
* @generated
*/
Manifestation createManifestation();
/**
* Returns a new object of class 'Operation'.
*
*
* @return a new object of class 'Operation'.
* @generated
*/
Operation createOperation();
/**
* Returns a new object of class 'Parameter Set'.
*
*
* @return a new object of class 'Parameter Set'.
* @generated
*/
ParameterSet createParameterSet();
/**
* Returns a new object of class 'Data Type'.
*
*
* @return a new object of class 'Data Type'.
* @generated
*/
DataType createDataType();
/**
* Returns a new object of class 'Interface'.
*
*
* @return a new object of class 'Interface'.
* @generated
*/
Interface createInterface();
/**
* Returns a new object of class 'Reception'.
*
*
* @return a new object of class 'Reception'.
* @generated
*/
Reception createReception();
/**
* Returns a new object of class 'Signal'.
*
*
* @return a new object of class 'Signal'.
* @generated
*/
Signal createSignal();
/**
* Returns a new object of class 'Protocol State Machine'.
*
*
* @return a new object of class 'Protocol State Machine'.
* @generated
*/
ProtocolStateMachine createProtocolStateMachine();
/**
* Returns a new object of class 'State Machine'.
*
*
* @return a new object of class 'State Machine'.
* @generated
*/
StateMachine createStateMachine();
/**
* Returns a new object of class 'Region'.
*
*
* @return a new object of class 'Region'.
* @generated
*/
Region createRegion();
/**
* Returns a new object of class 'Transition'.
*
*
* @return a new object of class 'Transition'.
* @generated
*/
Transition createTransition();
/**
* Returns a new object of class 'Trigger'.
*
*
* @return a new object of class 'Trigger'.
* @generated
*/
Trigger createTrigger();
/**
* Returns a new object of class 'Port'.
*
*
* @return a new object of class 'Port'.
* @generated
*/
Port createPort();
/**
* Returns a new object of class 'State'.
*
*
* @return a new object of class 'State'.
* @generated
*/
State createState();
/**
* Returns a new object of class 'Connection Point Reference'.
*
*
* @return a new object of class 'Connection Point Reference'.
* @generated
*/
ConnectionPointReference createConnectionPointReference();
/**
* Returns a new object of class 'Pseudostate'.
*
*
* @return a new object of class 'Pseudostate'.
* @generated
*/
Pseudostate createPseudostate();
/**
* Returns a new object of class 'Protocol Conformance'.
*
*
* @return a new object of class 'Protocol Conformance'.
* @generated
*/
ProtocolConformance createProtocolConformance();
/**
* Returns a new object of class 'Operation Template Parameter'.
*
*
* @return a new object of class 'Operation Template Parameter'.
* @generated
*/
OperationTemplateParameter createOperationTemplateParameter();
/**
* Returns a new object of class 'Association'.
*
*
* @return a new object of class 'Association'.
* @generated
*/
Association createAssociation();
/**
* Returns a new object of class 'Connectable Element Template Parameter'.
*
*
* @return a new object of class 'Connectable Element Template Parameter'.
* @generated
*/
ConnectableElementTemplateParameter createConnectableElementTemplateParameter();
/**
* Returns a new object of class 'Collaboration Use'.
*
*
* @return a new object of class 'Collaboration Use'.
* @generated
*/
CollaborationUse createCollaborationUse();
/**
* Returns a new object of class 'Collaboration'.
*
*
* @return a new object of class 'Collaboration'.
* @generated
*/
Collaboration createCollaboration();
/**
* Returns a new object of class 'Connector'.
*
*
* @return a new object of class 'Connector'.
* @generated
*/
Connector createConnector();
/**
* Returns a new object of class 'Redefinable Template Signature'.
*
*
* @return a new object of class 'Redefinable Template Signature'.
* @generated
*/
RedefinableTemplateSignature createRedefinableTemplateSignature();
/**
* Returns a new object of class 'Classifier Template Parameter'.
*
*
* @return a new object of class 'Classifier Template Parameter'.
* @generated
*/
ClassifierTemplateParameter createClassifierTemplateParameter();
/**
* Returns a new object of class 'Interface Realization'.
*
*
* @return a new object of class 'Interface Realization'.
* @generated
*/
InterfaceRealization createInterfaceRealization();
/**
* Returns a new object of class 'Extension'.
*
*
* @return a new object of class 'Extension'.
* @generated
*/
Extension createExtension();
/**
* Returns a new object of class 'Extension End'.
*
*
* @return a new object of class 'Extension End'.
* @generated
*/
ExtensionEnd createExtensionEnd();
/**
* Returns a new object of class 'String Expression'.
*
*
* @return a new object of class 'String Expression'.
* @generated
*/
StringExpression createStringExpression();
/**
* Returns a new object of class 'Expression'.
*
*
* @return a new object of class 'Expression'.
* @generated
*/
Expression createExpression();
/**
* Returns a new object of class 'Literal Integer'.
*
*
* @return a new object of class 'Literal Integer'.
* @generated
*/
LiteralInteger createLiteralInteger();
/**
* Returns a new object of class 'Literal String'.
*
*
* @return a new object of class 'Literal String'.
* @generated
*/
LiteralString createLiteralString();
/**
* Returns a new object of class 'Literal Boolean'.
*
*
* @return a new object of class 'Literal Boolean'.
* @generated
*/
LiteralBoolean createLiteralBoolean();
/**
* Returns a new object of class 'Literal Null'.
*
*
* @return a new object of class 'Literal Null'.
* @generated
*/
LiteralNull createLiteralNull();
/**
* Returns a new object of class 'Slot'.
*
*
* @return a new object of class 'Slot'.
* @generated
*/
Slot createSlot();
/**
* Returns a new object of class 'Instance Specification'.
*
*
* @return a new object of class 'Instance Specification'.
* @generated
*/
InstanceSpecification createInstanceSpecification();
/**
* Returns a new object of class 'Enumeration'.
*
*
* @return a new object of class 'Enumeration'.
* @generated
*/
Enumeration createEnumeration();
/**
* Returns a new object of class 'Enumeration Literal'.
*
*
* @return a new object of class 'Enumeration Literal'.
* @generated
*/
EnumerationLiteral createEnumerationLiteral();
/**
* Returns a new object of class 'Primitive Type'.
*
*
* @return a new object of class 'Primitive Type'.
* @generated
*/
PrimitiveType createPrimitiveType();
/**
* Returns a new object of class 'Instance Value'.
*
*
* @return a new object of class 'Instance Value'.
* @generated
*/
InstanceValue createInstanceValue();
/**
* Returns a new object of class 'Literal Unlimited Natural'.
*
*
* @return a new object of class 'Literal Unlimited Natural'.
* @generated
*/
LiteralUnlimitedNatural createLiteralUnlimitedNatural();
/**
* Returns a new object of class 'Opaque Behavior'.
*
*
* @return a new object of class 'Opaque Behavior'.
* @generated
*/
OpaqueBehavior createOpaqueBehavior();
/**
* Returns a new object of class 'Function Behavior'.
*
*
* @return a new object of class 'Function Behavior'.
* @generated
*/
FunctionBehavior createFunctionBehavior();
/**
* Returns a new object of class 'Actor'.
*
*
* @return a new object of class 'Actor'.
* @generated
*/
Actor createActor();
/**
* Returns a new object of class 'Usage'.
*
*
* @return a new object of class 'Usage'.
* @generated
*/
Usage createUsage();
/**
* Returns a new object of class 'Message'.
*
*
* @return a new object of class 'Message'.
* @generated
*/
Message createMessage();
/**
* Returns a new object of class 'Interaction'.
*
*
* @return a new object of class 'Interaction'.
* @generated
*/
Interaction createInteraction();
/**
* Returns a new object of class 'Lifeline'.
*
*
* @return a new object of class 'Lifeline'.
* @generated
*/
Lifeline createLifeline();
/**
* Returns a new object of class 'Part Decomposition'.
*
*
* @return a new object of class 'Part Decomposition'.
* @generated
*/
PartDecomposition createPartDecomposition();
/**
* Returns a new object of class 'Interaction Use'.
*
*
* @return a new object of class 'Interaction Use'.
* @generated
*/
InteractionUse createInteractionUse();
/**
* Returns a new object of class 'Gate'.
*
*
* @return a new object of class 'Gate'.
* @generated
*/
Gate createGate();
/**
* Returns a new object of class 'Activity'.
*
*
* @return a new object of class 'Activity'.
* @generated
*/
Activity createActivity();
/**
* Returns a new object of class 'Activity Partition'.
*
*
* @return a new object of class 'Activity Partition'.
* @generated
*/
ActivityPartition createActivityPartition();
/**
* Returns a new object of class 'Structured Activity Node'.
*
*
* @return a new object of class 'Structured Activity Node'.
* @generated
*/
StructuredActivityNode createStructuredActivityNode();
/**
* Returns a new object of class 'Variable'.
*
*
* @return a new object of class 'Variable'.
* @generated
*/
Variable createVariable();
/**
* Returns a new object of class 'Interruptible Activity Region'.
*
*
* @return a new object of class 'Interruptible Activity Region'.
* @generated
*/
InterruptibleActivityRegion createInterruptibleActivityRegion();
/**
* Returns a new object of class 'Exception Handler'.
*
*
* @return a new object of class 'Exception Handler'.
* @generated
*/
ExceptionHandler createExceptionHandler();
/**
* Returns a new object of class 'Output Pin'.
*
*
* @return a new object of class 'Output Pin'.
* @generated
*/
OutputPin createOutputPin();
/**
* Returns a new object of class 'Pin'.
*
*
* @return a new object of class 'Pin'.
* @generated
*/
Pin createPin();
/**
* Returns a new object of class 'Input Pin'.
*
*
* @return a new object of class 'Input Pin'.
* @generated
*/
InputPin createInputPin();
/**
* Returns a new object of class 'General Ordering'.
*
*
* @return a new object of class 'General Ordering'.
* @generated
*/
GeneralOrdering createGeneralOrdering();
/**
* Returns a new object of class 'Occurrence Specification'.
*
*
* @return a new object of class 'Occurrence Specification'.
* @generated
*/
OccurrenceSpecification createOccurrenceSpecification();
/**
* Returns a new object of class 'Interaction Operand'.
*
*
* @return a new object of class 'Interaction Operand'.
* @generated
*/
InteractionOperand createInteractionOperand();
/**
* Returns a new object of class 'Interaction Constraint'.
*
*
* @return a new object of class 'Interaction Constraint'.
* @generated
*/
InteractionConstraint createInteractionConstraint();
/**
* Returns a new object of class 'Execution Occurrence Specification'.
*
*
* @return a new object of class 'Execution Occurrence Specification'.
* @generated
*/
ExecutionOccurrenceSpecification createExecutionOccurrenceSpecification();
/**
* Returns a new object of class 'Execution Event'.
*
*
* @return a new object of class 'Execution Event'.
* @generated
*/
ExecutionEvent createExecutionEvent();
/**
* Returns a new object of class 'State Invariant'.
*
*
* @return a new object of class 'State Invariant'.
* @generated
*/
StateInvariant createStateInvariant();
/**
* Returns a new object of class 'Action Execution Specification'.
*
*
* @return a new object of class 'Action Execution Specification'.
* @generated
*/
ActionExecutionSpecification createActionExecutionSpecification();
/**
* Returns a new object of class 'Behavior Execution Specification'.
*
*
* @return a new object of class 'Behavior Execution Specification'.
* @generated
*/
BehaviorExecutionSpecification createBehaviorExecutionSpecification();
/**
* Returns a new object of class 'Creation Event'.
*
*
* @return a new object of class 'Creation Event'.
* @generated
*/
CreationEvent createCreationEvent();
/**
* Returns a new object of class 'Destruction Event'.
*
*
* @return a new object of class 'Destruction Event'.
* @generated
*/
DestructionEvent createDestructionEvent();
/**
* Returns a new object of class 'Send Operation Event'.
*
*
* @return a new object of class 'Send Operation Event'.
* @generated
*/
SendOperationEvent createSendOperationEvent();
/**
* Returns a new object of class 'Send Signal Event'.
*
*
* @return a new object of class 'Send Signal Event'.
* @generated
*/
SendSignalEvent createSendSignalEvent();
/**
* Returns a new object of class 'Message Occurrence Specification'.
*
*
* @return a new object of class 'Message Occurrence Specification'.
* @generated
*/
MessageOccurrenceSpecification createMessageOccurrenceSpecification();
/**
* Returns a new object of class 'Receive Operation Event'.
*
*
* @return a new object of class 'Receive Operation Event'.
* @generated
*/
ReceiveOperationEvent createReceiveOperationEvent();
/**
* Returns a new object of class 'Receive Signal Event'.
*
*
* @return a new object of class 'Receive Signal Event'.
* @generated
*/
ReceiveSignalEvent createReceiveSignalEvent();
/**
* Returns a new object of class 'Combined Fragment'.
*
*
* @return a new object of class 'Combined Fragment'.
* @generated
*/
CombinedFragment createCombinedFragment();
/**
* Returns a new object of class 'Continuation'.
*
*
* @return a new object of class 'Continuation'.
* @generated
*/
Continuation createContinuation();
/**
* Returns a new object of class 'Consider Ignore Fragment'.
*
*
* @return a new object of class 'Consider Ignore Fragment'.
* @generated
*/
ConsiderIgnoreFragment createConsiderIgnoreFragment();
/**
* Returns a new object of class 'Call Event'.
*
*
* @return a new object of class 'Call Event'.
* @generated
*/
CallEvent createCallEvent();
/**
* Returns a new object of class 'Change Event'.
*
*
* @return a new object of class 'Change Event'.
* @generated
*/
ChangeEvent createChangeEvent();
/**
* Returns a new object of class 'Signal Event'.
*
*
* @return a new object of class 'Signal Event'.
* @generated
*/
SignalEvent createSignalEvent();
/**
* Returns a new object of class 'Any Receive Event'.
*
*
* @return a new object of class 'Any Receive Event'.
* @generated
*/
AnyReceiveEvent createAnyReceiveEvent();
/**
* Returns a new object of class 'Create Object Action'.
*
*
* @return a new object of class 'Create Object Action'.
* @generated
*/
CreateObjectAction createCreateObjectAction();
/**
* Returns a new object of class 'Destroy Object Action'.
*
*
* @return a new object of class 'Destroy Object Action'.
* @generated
*/
DestroyObjectAction createDestroyObjectAction();
/**
* Returns a new object of class 'Test Identity Action'.
*
*
* @return a new object of class 'Test Identity Action'.
* @generated
*/
TestIdentityAction createTestIdentityAction();
/**
* Returns a new object of class 'Read Self Action'.
*
*
* @return a new object of class 'Read Self Action'.
* @generated
*/
ReadSelfAction createReadSelfAction();
/**
* Returns a new object of class 'Read Structural Feature Action'.
*
*
* @return a new object of class 'Read Structural Feature Action'.
* @generated
*/
ReadStructuralFeatureAction createReadStructuralFeatureAction();
/**
* Returns a new object of class 'Clear Structural Feature Action'.
*
*
* @return a new object of class 'Clear Structural Feature Action'.
* @generated
*/
ClearStructuralFeatureAction createClearStructuralFeatureAction();
/**
* Returns a new object of class 'Remove Structural Feature Value Action'.
*
*
* @return a new object of class 'Remove Structural Feature Value Action'.
* @generated
*/
RemoveStructuralFeatureValueAction createRemoveStructuralFeatureValueAction();
/**
* Returns a new object of class 'Add Structural Feature Value Action'.
*
*
* @return a new object of class 'Add Structural Feature Value Action'.
* @generated
*/
AddStructuralFeatureValueAction createAddStructuralFeatureValueAction();
/**
* Returns a new object of class 'Link End Data'.
*
*
* @return a new object of class 'Link End Data'.
* @generated
*/
LinkEndData createLinkEndData();
/**
* Returns a new object of class 'Qualifier Value'.
*
*
* @return a new object of class 'Qualifier Value'.
* @generated
*/
QualifierValue createQualifierValue();
/**
* Returns a new object of class 'Read Link Action'.
*
*
* @return a new object of class 'Read Link Action'.
* @generated
*/
ReadLinkAction createReadLinkAction();
/**
* Returns a new object of class 'Link End Creation Data'.
*
*
* @return a new object of class 'Link End Creation Data'.
* @generated
*/
LinkEndCreationData createLinkEndCreationData();
/**
* Returns a new object of class 'Create Link Action'.
*
*
* @return a new object of class 'Create Link Action'.
* @generated
*/
CreateLinkAction createCreateLinkAction();
/**
* Returns a new object of class 'Destroy Link Action'.
*
*
* @return a new object of class 'Destroy Link Action'.
* @generated
*/
DestroyLinkAction createDestroyLinkAction();
/**
* Returns a new object of class 'Link End Destruction Data'.
*
*
* @return a new object of class 'Link End Destruction Data'.
* @generated
*/
LinkEndDestructionData createLinkEndDestructionData();
/**
* Returns a new object of class 'Clear Association Action'.
*
*
* @return a new object of class 'Clear Association Action'.
* @generated
*/
ClearAssociationAction createClearAssociationAction();
/**
* Returns a new object of class 'Broadcast Signal Action'.
*
*
* @return a new object of class 'Broadcast Signal Action'.
* @generated
*/
BroadcastSignalAction createBroadcastSignalAction();
/**
* Returns a new object of class 'Send Object Action'.
*
*
* @return a new object of class 'Send Object Action'.
* @generated
*/
SendObjectAction createSendObjectAction();
/**
* Returns a new object of class 'Value Specification Action'.
*
*
* @return a new object of class 'Value Specification Action'.
* @generated
*/
ValueSpecificationAction createValueSpecificationAction();
/**
* Returns a new object of class 'Time Expression'.
*
*
* @return a new object of class 'Time Expression'.
* @generated
*/
TimeExpression createTimeExpression();
/**
* Returns a new object of class 'Duration'.
*
*
* @return a new object of class 'Duration'.
* @generated
*/
Duration createDuration();
/**
* Returns a new object of class 'Value Pin'.
*
*
* @return a new object of class 'Value Pin'.
* @generated
*/
ValuePin createValuePin();
/**
* Returns a new object of class 'Duration Interval'.
*
*
* @return a new object of class 'Duration Interval'.
* @generated
*/
DurationInterval createDurationInterval();
/**
* Returns a new object of class 'Interval'.
*
*
* @return a new object of class 'Interval'.
* @generated
*/
Interval createInterval();
/**
* Returns a new object of class 'Time Constraint'.
*
*
* @return a new object of class 'Time Constraint'.
* @generated
*/
TimeConstraint createTimeConstraint();
/**
* Returns a new object of class 'Interval Constraint'.
*
*
* @return a new object of class 'Interval Constraint'.
* @generated
*/
IntervalConstraint createIntervalConstraint();
/**
* Returns a new object of class 'Time Interval'.
*
*
* @return a new object of class 'Time Interval'.
* @generated
*/
TimeInterval createTimeInterval();
/**
* Returns a new object of class 'Duration Constraint'.
*
*
* @return a new object of class 'Duration Constraint'.
* @generated
*/
DurationConstraint createDurationConstraint();
/**
* Returns a new object of class 'Time Observation'.
*
*
* @return a new object of class 'Time Observation'.
* @generated
*/
TimeObservation createTimeObservation();
/**
* Returns a new object of class 'Duration Observation'.
*
*
* @return a new object of class 'Duration Observation'.
* @generated
*/
DurationObservation createDurationObservation();
/**
* Returns a new object of class 'Opaque Action'.
*
*
* @return a new object of class 'Opaque Action'.
* @generated
*/
OpaqueAction createOpaqueAction();
/**
* Returns a new object of class 'Send Signal Action'.
*
*
* @return a new object of class 'Send Signal Action'.
* @generated
*/
SendSignalAction createSendSignalAction();
/**
* Returns a new object of class 'Call Operation Action'.
*
*
* @return a new object of class 'Call Operation Action'.
* @generated
*/
CallOperationAction createCallOperationAction();
/**
* Returns a new object of class 'Call Behavior Action'.
*
*
* @return a new object of class 'Call Behavior Action'.
* @generated
*/
CallBehaviorAction createCallBehaviorAction();
/**
* Returns a new object of class 'Information Item'.
*
*
* @return a new object of class 'Information Item'.
* @generated
*/
InformationItem createInformationItem();
/**
* Returns a new object of class 'Information Flow'.
*
*
* @return a new object of class 'Information Flow'.
* @generated
*/
InformationFlow createInformationFlow();
/**
* Returns a new object of class 'Model'.
*
*
* @return a new object of class 'Model'.
* @generated
*/
Model createModel();
/**
* Returns a new object of class 'Read Variable Action'.
*
*
* @return a new object of class 'Read Variable Action'.
* @generated
*/
ReadVariableAction createReadVariableAction();
/**
* Returns a new object of class 'Clear Variable Action'.
*
*
* @return a new object of class 'Clear Variable Action'.
* @generated
*/
ClearVariableAction createClearVariableAction();
/**
* Returns a new object of class 'Add Variable Value Action'.
*
*
* @return a new object of class 'Add Variable Value Action'.
* @generated
*/
AddVariableValueAction createAddVariableValueAction();
/**
* Returns a new object of class 'Remove Variable Value Action'.
*
*
* @return a new object of class 'Remove Variable Value Action'.
* @generated
*/
RemoveVariableValueAction createRemoveVariableValueAction();
/**
* Returns a new object of class 'Raise Exception Action'.
*
*
* @return a new object of class 'Raise Exception Action'.
* @generated
*/
RaiseExceptionAction createRaiseExceptionAction();
/**
* Returns a new object of class 'Action Input Pin'.
*
*
* @return a new object of class 'Action Input Pin'.
* @generated
*/
ActionInputPin createActionInputPin();
/**
* Returns a new object of class 'Read Extent Action'.
*
*
* @return a new object of class 'Read Extent Action'.
* @generated
*/
ReadExtentAction createReadExtentAction();
/**
* Returns a new object of class 'Reclassify Object Action'.
*
*
* @return a new object of class 'Reclassify Object Action'.
* @generated
*/
ReclassifyObjectAction createReclassifyObjectAction();
/**
* Returns a new object of class 'Read Is Classified Object Action'.
*
*
* @return a new object of class 'Read Is Classified Object Action'.
* @generated
*/
ReadIsClassifiedObjectAction createReadIsClassifiedObjectAction();
/**
* Returns a new object of class 'Start Classifier Behavior Action'.
*
*
* @return a new object of class 'Start Classifier Behavior Action'.
* @generated
*/
StartClassifierBehaviorAction createStartClassifierBehaviorAction();
/**
* Returns a new object of class 'Read Link Object End Action'.
*
*
* @return a new object of class 'Read Link Object End Action'.
* @generated
*/
ReadLinkObjectEndAction createReadLinkObjectEndAction();
/**
* Returns a new object of class 'Read Link Object End Qualifier Action'.
*
*
* @return a new object of class 'Read Link Object End Qualifier Action'.
* @generated
*/
ReadLinkObjectEndQualifierAction createReadLinkObjectEndQualifierAction();
/**
* Returns a new object of class 'Create Link Object Action'.
*
*
* @return a new object of class 'Create Link Object Action'.
* @generated
*/
CreateLinkObjectAction createCreateLinkObjectAction();
/**
* Returns a new object of class 'Accept Event Action'.
*
*
* @return a new object of class 'Accept Event Action'.
* @generated
*/
AcceptEventAction createAcceptEventAction();
/**
* Returns a new object of class 'Accept Call Action'.
*
*
* @return a new object of class 'Accept Call Action'.
* @generated
*/
AcceptCallAction createAcceptCallAction();
/**
* Returns a new object of class 'Reply Action'.
*
*
* @return a new object of class 'Reply Action'.
* @generated
*/
ReplyAction createReplyAction();
/**
* Returns a new object of class 'Unmarshall Action'.
*
*
* @return a new object of class 'Unmarshall Action'.
* @generated
*/
UnmarshallAction createUnmarshallAction();
/**
* Returns a new object of class 'Reduce Action'.
*
*
* @return a new object of class 'Reduce Action'.
* @generated
*/
ReduceAction createReduceAction();
/**
* Returns a new object of class 'Start Object Behavior Action'.
*
*
* @return a new object of class 'Start Object Behavior Action'.
* @generated
*/
StartObjectBehaviorAction createStartObjectBehaviorAction();
/**
* Returns a new object of class 'Control Flow'.
*
*
* @return a new object of class 'Control Flow'.
* @generated
*/
ControlFlow createControlFlow();
/**
* Returns a new object of class 'Initial Node'.
*
*
* @return a new object of class 'Initial Node'.
* @generated
*/
InitialNode createInitialNode();
/**
* Returns a new object of class 'Activity Parameter Node'.
*
*
* @return a new object of class 'Activity Parameter Node'.
* @generated
*/
ActivityParameterNode createActivityParameterNode();
/**
* Returns a new object of class 'Fork Node'.
*
*
* @return a new object of class 'Fork Node'.
* @generated
*/
ForkNode createForkNode();
/**
* Returns a new object of class 'Flow Final Node'.
*
*
* @return a new object of class 'Flow Final Node'.
* @generated
*/
FlowFinalNode createFlowFinalNode();
/**
* Returns a new object of class 'Central Buffer Node'.
*
*
* @return a new object of class 'Central Buffer Node'.
* @generated
*/
CentralBufferNode createCentralBufferNode();
/**
* Returns a new object of class 'Merge Node'.
*
*
* @return a new object of class 'Merge Node'.
* @generated
*/
MergeNode createMergeNode();
/**
* Returns a new object of class 'Decision Node'.
*
*
* @return a new object of class 'Decision Node'.
* @generated
*/
DecisionNode createDecisionNode();
/**
* Returns a new object of class 'Activity Final Node'.
*
*
* @return a new object of class 'Activity Final Node'.
* @generated
*/
ActivityFinalNode createActivityFinalNode();
/**
* Returns a new object of class 'Join Node'.
*
*
* @return a new object of class 'Join Node'.
* @generated
*/
JoinNode createJoinNode();
/**
* Returns a new object of class 'Data Store Node'.
*
*
* @return a new object of class 'Data Store Node'.
* @generated
*/
DataStoreNode createDataStoreNode();
/**
* Returns a new object of class 'Object Flow'.
*
*
* @return a new object of class 'Object Flow'.
* @generated
*/
ObjectFlow createObjectFlow();
/**
* Returns a new object of class 'Sequence Node'.
*
*
* @return a new object of class 'Sequence Node'.
* @generated
*/
SequenceNode createSequenceNode();
/**
* Returns a new object of class 'Conditional Node'.
*
*
* @return a new object of class 'Conditional Node'.
* @generated
*/
ConditionalNode createConditionalNode();
/**
* Returns a new object of class 'Clause'.
*
*
* @return a new object of class 'Clause'.
* @generated
*/
Clause createClause();
/**
* Returns a new object of class 'Loop Node'.
*
*
* @return a new object of class 'Loop Node'.
* @generated
*/
LoopNode createLoopNode();
/**
* Returns a new object of class 'Expansion Node'.
*
*
* @return a new object of class 'Expansion Node'.
* @generated
*/
ExpansionNode createExpansionNode();
/**
* Returns a new object of class 'Expansion Region'.
*
*
* @return a new object of class 'Expansion Region'.
* @generated
*/
ExpansionRegion createExpansionRegion();
/**
* Returns a new object of class 'Component Realization'.
*
*
* @return a new object of class 'Component Realization'.
* @generated
*/
ComponentRealization createComponentRealization();
/**
* Returns a new object of class 'Component'.
*
*
* @return a new object of class 'Component'.
* @generated
*/
Component createComponent();
/**
* Returns a new object of class 'Node'.
*
*
* @return a new object of class 'Node'.
* @generated
*/
Node createNode();
/**
* Returns a new object of class 'Device'.
*
*
* @return a new object of class 'Device'.
* @generated
*/
Device createDevice();
/**
* Returns a new object of class 'Execution Environment'.
*
*
* @return a new object of class 'Execution Environment'.
* @generated
*/
ExecutionEnvironment createExecutionEnvironment();
/**
* Returns a new object of class 'Communication Path'.
*
*
* @return a new object of class 'Communication Path'.
* @generated
*/
CommunicationPath createCommunicationPath();
/**
* Returns a new object of class 'Final State'.
*
*
* @return a new object of class 'Final State'.
* @generated
*/
FinalState createFinalState();
/**
* Returns a new object of class 'Time Event'.
*
*
* @return a new object of class 'Time Event'.
* @generated
*/
TimeEvent createTimeEvent();
/**
* Returns a new object of class 'Protocol Transition'.
*
*
* @return a new object of class 'Protocol Transition'.
* @generated
*/
ProtocolTransition createProtocolTransition();
/**
* Returns a new object of class 'Association Class'.
*
*
* @return a new object of class 'Association Class'.
* @generated
*/
AssociationClass createAssociationClass();
/**
* Returns the package supported by this factory.
*
*
* @return the package supported by this factory.
* @generated
*/
UMLPackage getUMLPackage();
} //UMLFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy