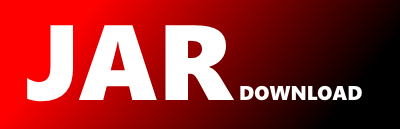
org.eclipse.virgo.util.common.Assert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.eclipse.virgo.util.common Show documentation
Show all versions of org.eclipse.virgo.util.common Show documentation
Virgo from EclipseRT is a completely module-based Java application server
The newest version!
/*******************************************************************************
* Copyright (c) 2008, 2010 VMware Inc.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* VMware Inc. - initial contribution
*******************************************************************************/
package org.eclipse.virgo.util.common;
import java.util.Collection;
import java.util.Map;
/**
* A set of useful assertions based on those provided by the Spring Framework's Assert class.
*
* Concurrent Semantics
*
* This class is thread safe.
*
* @see org.junit.Assert
*/
public final class Assert {
/**
* Assert a boolean expression, throwing a IllegalArgumentException
if the test result is
* false
.
*
*
* Assert.isTrue(i > 0, "The value must be greater than zero");
*
*
* @param expression a boolean expression
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if expression is false
*/
public static void isTrue(boolean expression, String message, Object... inserts) {
if (!expression) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert a boolean expression, throwing a IllegalArgumentException
if the test result is
* true
.
*
*
* Assert.isFalse(state.isBroken(), "The state is broken");
*
*
* @param expression a boolean expression
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if expression is false
*/
public static void isFalse(boolean expression, String message, Object... inserts) {
if (expression) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that an object is null
.
*
*
* Assert.isNull(value, "The value must be null");
*
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the object is not null
*/
public static void isNull(Object object, String message, Object... inserts) {
if (object != null) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that an object is not null
.
*
*
* Assert.notNull(clazz, "The class must not be null");
*
*
* @param object the object to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the object is null
*/
public static void notNull(Object object, String message, Object... inserts) {
if (object == null) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that the given String is not empty; that is, it must not be null
and not the empty String.
*
*
* Assert.hasLength(name, "Name must not be empty");
*
*
* @param text the String to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @see StringUtils#hasLength
*/
public static void hasLength(String text, String message, Object... inserts) {
if (text == null || text.length() == 0) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that an array has elements; that is, it must not be null
and must have at least one
* element.
*
*
* Assert.notEmpty(array, "The array must have elements");
*
*
* @param array the array to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the object array is null
or has no elements
*/
public static void notEmpty(Object[] array, String message, Object... inserts) {
if (array == null || array.length == 0) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that a collection has elements; that is, it must not be null
and must have at least one
* element.
*
*
* Assert.notEmpty(collection, "Collection must have elements");
*
* @param the type of the elements of the collection
*
* @param collection the collection to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the collection is null
or has no elements
*/
public static void notEmpty(Collection collection, String message, Object... inserts) {
if (collection == null || collection.isEmpty()) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that a Map has entries; that is, it must not be null
and must have at least one entry.
*
*
* Assert.notEmpty(map, "Map must have entries");
*
* @param type of Key (domain)
* @param type of Value (range)
*
* @param map the map to check
* @param message the exception message to use if the assertion fails
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the map is null
or has no entries
*/
public static void notEmpty(Map map, String message, Object... inserts) {
if (map == null || map.isEmpty()) {
throw new IllegalArgumentException(String.format(message, inserts));
}
}
/**
* Assert that the provided object is a non-null instance of the provided class.
*
*
* Assert.instanceOf(Foo.class, foo);
*
* @param the type to check this for
*
* @param type the type to check against
* @param obj the object to check
* @param message a message which will be prepended to the message produced by the function itself, and which may be
* used to provide context. It should normally end in a ": " or ". " so that the function generate message
* looks ok when appended to it.
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the object is not an instance of clazz
* @see Class#isInstance
*/
public static void isInstanceOf(Class type, Object obj, String message, Object... inserts) {
notNull(type, "The type to check against must not be null");
if (!type.isInstance(obj)) {
throw new IllegalArgumentException(String.format(message, inserts) + "Object of class [" + (obj != null ? obj.getClass().getName() : "null")
+ "] must be an instance of " + type);
}
}
/**
* Assert that superType.isAssignableFrom(subType)
is true
.
*
*
* Assert.isAssignable(Number.class, myClass);
*
* @param the supertype
* @param the subtype
*
* @param superType the super type to check against
* @param subType the sub type to check
* @param message a message which will be prepended to the message produced by the function itself, and which may be
* used to provide context. It should normally end in a ": " or ". " so that the function generate message
* looks ok when appended to it.
* @param inserts any inserts to include if the message is a format string.
* @throws IllegalArgumentException if the classes are not assignable
*/
public static void isAssignable(Class superType, Class subType, String message, Object... inserts) {
notNull(superType, "Type to check against must not be null");
if (subType == null || !superType.isAssignableFrom(subType)) {
throw new IllegalArgumentException(String.format(message, inserts) + subType + " is not assignable to " + superType);
}
}
/*
* Prevent instantiation - Java does not allow final abstract classes.
*/
private Assert() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy