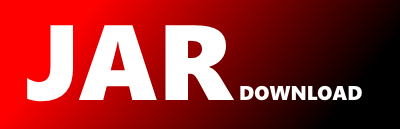
org.eclipse.virgo.util.common.GraphNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.eclipse.virgo.util.common Show documentation
Show all versions of org.eclipse.virgo.util.common Show documentation
Virgo from EclipseRT is a completely module-based Java application server
The newest version!
/*******************************************************************************
* Copyright (c) 2011 VMware Inc. and others
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* VMware Inc. - initial contribution (Tree.java)
* EclipseSource - reworked from generic tree to DAG (Bug 358697)
*******************************************************************************/
package org.eclipse.virgo.util.common;
import java.util.List;
/**
* {@link GraphNode} is a node in a {@link DirectedAcyclicGraph}. Each node has a value.
*
*
* Concurrent Semantics
*
* Implementations of this interface may or may not be thread safe.
*
* @param type of values in the graph nodes.
*/
public interface GraphNode {
/**
* {@link DirectedAcyclicGraphVisitor} is used to visit a node and, at the option of the visitor, its subgraphs
* recursively. Note: Shared nodes are only visited once.
*
*
* Concurrent Semantics
*
* Implementations of this interface should be thread safe when used with a thread safe
* DirectedAcyclicGraph
implementation.
*
* @param type of values in graph nodes
*/
interface DirectedAcyclicGraphVisitor {
/**
* Visits the given {@link GraphNode}. The return value determines whether or not any subgraphs of the given
* node are visited.
*
* @param node the GraphNode
to be visited
* @return true
if and only if the subgraphs of the given node should be visited.
*/
boolean visit(GraphNode node);
}
/**
* An ExceptionThrowingDirectedAcyclicGraphVisitor
is used to visit a node and, at the option of the
* visitor, its subgraphs recursively if the {@link #visit(GraphNode)} implementation needs to be able to throw a
* checked {@link Exception}. Note: Shared nodes are only visited once.
*
*
* Concurrent Semantics
*
* Implementations of this interface should be thread safe when used with a thread safe
* DirectedAcyclicGraph
implementation.
*
* @param type of values in graph nodes
* @param type of exceptions possibly thrown
*/
interface ExceptionThrowingDirectedAcyclicGraphVisitor {
/**
* Visits the given {@link GraphNode}. The return value determines whether or not any subgraphs of the given
* node are visited.
*
* @param node the GraphNode
to be visited
* @throws E if an error occurs when visiting the given node
* @return true
if and only if the subgraphs of the given node should be visited.
*/
boolean visit(GraphNode node) throws E;
}
/**
* Returns the node's value. If there is no value associated with this node, returns null
.
*
* @return the value, which may be null
*/
V getValue();
/**
* Returns a list of this node's children (not copies of the children). If the node has no children, returns an
* empty list. Never returns null
.
*
* @return this node's children
*/
List> getChildren();
/**
* Adds the given node as child to this node. The child node is not copied.
*
* @param child the node to add
* @throws IllegalArgumentException if the given node does not belong to the same {@link DirectedAcyclicGraph}.
* @throws IllegalArgumentException if the given node is already a child of this node.
*/
void addChild(GraphNode child);
/**
* Removes the occurrence of the given node from this node's children. Returns true
if the child was
* found and removed, otherwise false
.
*
* @param child the node to remove
* @throws IllegalArgumentException if the given node does not belong to the same {@link DirectedAcyclicGraph}.
* @return true
if the node was removed successfully, otherwise false
.
* @see java.util.List#remove
*/
boolean removeChild(GraphNode child);
/**
* Returns a list of this node's parents (not copies of the parents). If this node does not have any parents,
* returns an empty list. Never returns null
.
*
* @return this node's parents
*/
List> getParents();
/**
* Traverse this {@link GraphNode} in preorder (see below) and call the visit method of the given
* {@link DirectedAcyclicGraphVisitor} at each node. The visitor determines whether the subgraphs of each visited
* node should also be visited. Note: Shared nodes are only visited once.
*
* Preorder traversal visits the node and then visits, in preorder, each subgraph of the node.
*
* @param visitor a {@link DirectedAcyclicGraphVisitor}
*/
void visit(DirectedAcyclicGraphVisitor visitor);
/**
* Traverse this {@link GraphNode} in preorder (see below) and call the visit method of the given
* {@link ExceptionThrowingDirectedAcyclicGraphVisitor} at each node. The visitor determines whether the subgraph of
* each visited node should also be visited. Note: Shared nodes are only visited once.
*
* Preorder traversal visits the node and then visits, in preorder, each subgraph of the node.
*
* @param type of exception possibly thrown
* @param visitor a {@link ExceptionThrowingDirectedAcyclicGraphVisitor}
* @throws E if an error occurs when visiting the node
*/
void visit(ExceptionThrowingDirectedAcyclicGraphVisitor visitor) throws E;
/**
* Returns the number of nodes in the subgraph of this node. The subgraph includes the node itself as the only root
* node.
*
*
* Note: Nodes may be shared and shared nodes are counted only once.
*
*
* If there are more than Integer.MAX_VALUE nodes, the return value is undefined and the user should seek
* professional help.
*
* @return the number of nodes in the subgraph
*/
int size();
/**
* Check if this node is a root node (a node without parents).
*
* @return true
if the node has no parents. Otherwise false
.
*/
boolean isRootNode();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy