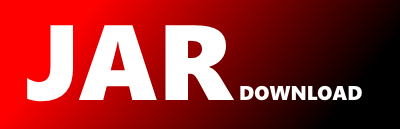
org.eclipse.virgo.util.common.Tree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.eclipse.virgo.util.common Show documentation
Show all versions of org.eclipse.virgo.util.common Show documentation
Virgo from EclipseRT is a completely module-based Java application server
The newest version!
/*******************************************************************************
* Copyright (c) 2008, 2010 VMware Inc.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* VMware Inc. - initial contribution
*******************************************************************************/
package org.eclipse.virgo.util.common;
import java.util.List;
/**
* {@link Tree} is a value with an ordered collection of subtrees of the same type as the main tree.
*
*
* Concurrent Semantics
*
* Implementations of this interface may or may not be thread safe.
*
* @param type of values in tree nodes
*/
public interface Tree {
/**
* {@link Tree.TreeVisitor} is an interface used to visit a tree and, at the option of the visitor, its children and so
* on recursively.
*
*
* Concurrent Semantics
*
* Implementations of this interface should be thread safe when used with a thread safe Tree
* implementation.
* @param type of values in tree nodes
*/
public interface TreeVisitor {
/**
* Visits the given {@link Tree}. The return value determines whether or not any children of the given tree are
* visited.
*
* @param tree a Tree
* @return true
if and only if the children of the given tree should be visited.
*/
boolean visit(Tree tree);
}
/**
* An ExceptionThrowingTreeVisitor
is used to visit a tree when the {@link #visit(Tree)} implementation
* needs to be able to throw a checked {@link Exception}.
*
*
* Concurrent Semantics
*
* Implementations of this interface should be thread safe when used with a thread safe Tree
* implementation.
* @param type of values in tree nodes
* @param type of exceptions possibly thrown
*/
public interface ExceptionThrowingTreeVisitor {
/**
* Visits the given {@link Tree}. The return value determines whether or not any children of the given tree are
* visited.
*
* @param tree a Tree
* @throws E if an error occurs when visiting the tree
* @return true
if and only if the children of the given tree should be visited.
*/
boolean visit(Tree tree) throws E;
}
/**
* Returns the tree's value. If there is no value associated with this tree, returns null
.
*
* @return the value, which may be null
*/
V getValue();
/**
* Returns a list of this tree's children (not copies of the children). If the tree has no children, returns an
* empty list. Never returns null
.
*
* @return this tree's children
*/
List> getChildren();
/**
* Adds a new child tree to this node's children. The child tree is copied, although its values are not.
*
* @param child the child tree to add
* @return the copy of the child tree
*/
Tree addChild(Tree child);
/**
* Removes the first occurrence of the given child tree from this node's children. Returns true
if the
* child was found and removed, otherwise false
.
*
* @param child the child tree to remove
* @return true
if the child tree was removed successfully, otherwise false
.
* @see java.util.List#remove
*/
boolean removeChild(Tree child);
/**
* Returns this tree's parent. If this tree does not have a parent, returns null
.
*
* @return this tree's parent
*/
Tree getParent();
/**
* Traverse this {@link Tree} in preorder (see below) and call the visit method of the given {@link TreeVisitor} at
* each node. The visitor determines whether the children of each visited tree should also be visited.
*
* Preorder traversal visits the tree and then visits, in preorder, each child of the tree.
*
* @param visitor a {@link TreeVisitor}
*/
void visit(TreeVisitor visitor);
/**
* Traverse this {@link Tree} in preorder (see below) and call the visit method of the given
* {@link ExceptionThrowingTreeVisitor} at each node. The visitor determines whether the children of each visited
* tree should also be visited.
*
* Preorder traversal visits the tree and then visits, in preorder, each child of the tree.
* @param type of exception possibly thrown
*
* @param visitor the tree's visitor
* @throws E if an error occurs when visiting the tree
*/
void visit(ExceptionThrowingTreeVisitor visitor) throws E;
/**
* Returns the number of nodes in the tree. This is one plus the sum of the number of nodes in each of the children.
*
* If there are more than Integer.MAX_VALUE node, the return value is undefined and the user should seek
* professional help.
*
* @return the number of non-null
nodes in the tree
*/
int size();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy