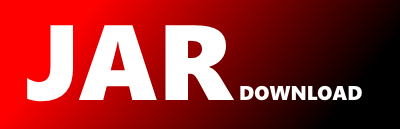
org.eclipse.xtext.parser.antlr.XtextTokenStream Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2008 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package org.eclipse.xtext.parser.antlr;
import java.util.ArrayList;
import java.util.BitSet;
import java.util.HashMap;
import java.util.Map;
import org.antlr.runtime.CharStream;
import org.antlr.runtime.CommonToken;
import org.antlr.runtime.CommonTokenStream;
import org.antlr.runtime.Lexer;
import org.antlr.runtime.Token;
import org.antlr.runtime.TokenSource;
/**
* A token stream that is aware of the current lookahead.
*
* @author Jan K?hnlein - Initial contribution and API
* @author Sebastian Zarnekow - Support for dynamic hidden tokens,
* reworked lookahead algorithm
*/
public class XtextTokenStream extends CommonTokenStream {
private int currentLookAhead;
private Map rulenameToTokenType;
private BitSet hiddenTokens;
public XtextTokenStream() {
super();
tokens = new TokenList(500);
}
public XtextTokenStream(TokenSource tokenSource, int channel) {
super(tokenSource, channel);
tokens = new TokenList(500);
}
public XtextTokenStream(TokenSource tokenSource, ITokenDefProvider tokenDefProvider) {
super(tokenSource);
tokens = new TokenList(500);
rulenameToTokenType = new HashMap(tokenDefProvider.getTokenDefMap().size());
for(Map.Entry entry: tokenDefProvider.getTokenDefMap().entrySet()) {
rulenameToTokenType.put(entry.getValue(), entry.getKey());
}
}
@Override
public String toString(int start, int stop) {
if ( start<0 || stop<0 ) {
return null;
}
if ( p == -1 ) {
fillBuffer();
}
if ( stop>=tokens.size() ) {
stop = tokens.size()-1;
}
if (tokenSource instanceof Lexer) {
Token startToken = (Token) tokens.get(start);
Token stopToken = (Token) tokens.get(stop);
if (startToken instanceof CommonToken && stopToken instanceof CommonToken) {
CommonToken commonStart = (CommonToken) startToken;
CommonToken commonStop = (CommonToken) stopToken;
CharStream charStream = ((Lexer) tokenSource).getCharStream();
String result = charStream.substring(commonStart.getStartIndex(), commonStop.getStopIndex());
return result;
}
}
// fall back to super implementation but use StringBuilder instead of StringBuffer
// and use reasonable initialization size
StringBuilder result = new StringBuilder(Math.max(1024, tokens.size() * 6));
for (int i = start; i <= stop; i++) {
Token t = (Token)tokens.get(i);
result.append(t.getText());
}
return result.toString();
}
@SuppressWarnings({ "serial" })
private final class TokenList extends ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy