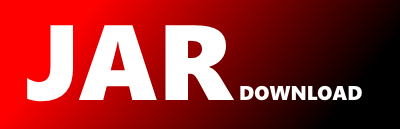
org.eclipse.xtext.parsetree.reconstr.Serializer Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.parsetree.reconstr;
import java.io.IOException;
import java.io.Writer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.eclipse.emf.common.util.Diagnostic;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.formatting.IFormatter;
import org.eclipse.xtext.formatting.IFormatterExtension;
import org.eclipse.xtext.parsetree.reconstr.IParseTreeConstructor.TreeConstructionReport;
import org.eclipse.xtext.parsetree.reconstr.impl.TokenStringBuffer;
import org.eclipse.xtext.parsetree.reconstr.impl.WriterTokenStream;
import org.eclipse.xtext.resource.SaveOptions;
import org.eclipse.xtext.serializer.ISerializer;
import org.eclipse.xtext.util.ReplaceRegion;
import org.eclipse.xtext.validation.IConcreteSyntaxValidator;
import com.google.inject.Inject;
/**
* @author Moritz Eysholdt - Initial contribution and API
* @author Jan Koehnlein
*/
public class Serializer implements ISerializer {
private IParseTreeConstructor parseTreeReconstructor;
private IFormatter formatter;
private IConcreteSyntaxValidator validator;
@Inject
public Serializer(IParseTreeConstructor ptc, IFormatter fmt, IConcreteSyntaxValidator val) {
this.parseTreeReconstructor = ptc;
this.formatter = fmt;
this.validator = val;
}
public TreeConstructionReport serialize(EObject obj, ITokenStream tokenStream, SaveOptions options)
throws IOException {
if (options.isValidating()) {
List diagnostics = new ArrayList();
validator.validateRecursive(obj, new IConcreteSyntaxValidator.DiagnosticListAcceptor(diagnostics),
new HashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy