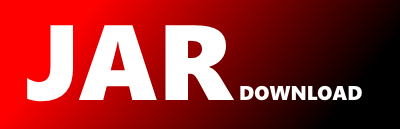
org.eclipse.xtext.resource.IResourceDescription Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.resource;
import java.util.Collection;
import org.eclipse.emf.common.util.URI;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.xtext.naming.QualifiedName;
import org.eclipse.xtext.resource.impl.DefaultResourceDescriptionManager;
import com.google.common.collect.ImmutableList;
import com.google.inject.ImplementedBy;
/**
* A representation of a resource's contents as an {@link ISelectable}.
* The exported objects of a {@link Resource} describe its public interface.
* A {@link IResourceDescription description} holds information about the
* {@link #getImportedNames() imported names} which can be used to compute the
* transitive closure when a resource is modified. Information about the actually
* established {@link #getReferenceDescriptions() cross references} is available, too.
*
* @author Sebastian Zarnekow - Initial contribution and API
* @author Sven Efftinge
* @author Jan Koehnlein
*/
public interface IResourceDescription extends ISelectable {
/**
* @return descriptions of all EObjects provided by the given Resource. The result is expected to return any
* combination of name
and eObjectOrProxy
only once as an
* {@link IEObjectDescription}. The order of the exported objects matters.
*/
Iterable getExportedObjects();
/**
* @return the list of names the described resource depends depends on.
*/
Iterable getImportedNames();
/**
* @return the list of all references contained in the underlying resource.
*/
Iterable getReferenceDescriptions();
/**
* @return the uri of the described resource. Will not return null
.
*/
URI getURI();
@ImplementedBy(DefaultResourceDescriptionManager.class)
interface Manager {
/**
* @return a resource description for the given resource. The result represents the current state of the given
* resource.
*/
IResourceDescription getResourceDescription(Resource resource);
/**
* @return a delta for both given descriptions.
*/
IResourceDescription.Delta createDelta(IResourceDescription oldDescription, IResourceDescription newDescription);
/**
* @return whether the candidate is affected by the change in the delta.
* @throws IllegalArgumentException
* if this manager is not responsible for the given candidate.
*/
boolean isAffected(IResourceDescription.Delta delta, IResourceDescription candidate)
throws IllegalArgumentException;
/**
* Batch operation to check whether a description is affected by any given delta in
* the given context. Implementations may perform any optimizations to return false
whenever
* possible, e.g. check the deltas against the visible containers.
* @param deltas List of deltas to check. May not be null
.
* @param candidate The description to check. May not be null
.
* @param context The current context of the batch operation. May not be null
.
* @return whether the condidate is affected by any of the given changes.
* @throws IllegalArgumentException
* if this manager is not responsible for the given candidate.
*/
boolean isAffected(Collection deltas,
IResourceDescription candidate,
IResourceDescriptions context)
throws IllegalArgumentException;
}
/**
* A delta describing the differences between two versions of the same {@link IResourceDescription}. Instances have
* to follow the rule :
*
* getNew()==null || getOld()==null || getOld().getURI().equals(getNew().getURI())
*
* and
*
* getNew()!=getOld()
*
*
*/
interface Delta {
/**
*@return the uri for the resource description delta.
*/
URI getUri();
/**
* @return the old resource description, or null if the change is an addition
*/
IResourceDescription getOld();
/**
* @return the new resource description, or null if the change is a deletion
*/
IResourceDescription getNew();
/**
* @return whether there are differences between the old and the new resource description.
*/
boolean haveEObjectDescriptionsChanged();
}
interface Event {
/**
* @return the list of changes. It is never null
but may be empty.
*/
ImmutableList getDeltas();
/**
* @return the sender of this event. Is never null
.
*/
Source getSender();
interface Source {
/**
* Add a listener to the event source. Listeners will not be added twice. Subsequent calls to
* {@link #addListener(Listener)} will not affect the number of events that the listener receives.
* {@link #removeListener(Listener)} will remove the listener immediately independently from the number of
* invocations of {@link #addListener(Listener)} for the given listener.
*
* @param listener
* the listener to be registered. May not be null
.
*/
void addListener(Listener listener);
/**
* Immediately removes a registered listener from the source. However if {@link #removeListener(Listener)}
* is called during a notification, the removed listener will still receive the event. If the listener has
* not been registered before, the {@link #removeListener(Listener)} does nothing.
*
* @param listener
* the listener to be removed. May not be null
.
*/
void removeListener(Listener listener);
}
/**
* A listener for events raised by a {@link Event.Source}.
*/
interface Listener {
/**
*
* The source will invoce this method to announce changed resource. The event will never be
* null
. However, it may contain an empty list of deltas.
*
*
* Listeners are free to remove themselves from the sender of the event or add other listeners. However
* added listeners will not be informed about the current change.
*
*
* This event may be fired asynchronously. It is ensured that the changed resources will provide the content
* as it was when the change has been announced to the sender of the event.
*
*
* @param event
* the fired event. Will never be null
.
*/
void descriptionsChanged(Event event);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy