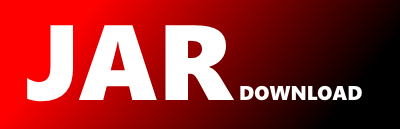
org.eclipse.xtext.resource.SaveOptions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2010 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.resource;
import java.util.Map;
import com.google.common.collect.Maps;
/**
* Immutable SaveOptions can be used to read and write options into the
* map that is passed into {@link org.eclipse.emf.ecore.resource.Resource#save(Map)}.
* Clients should create options by means of SaveOptions.newBuilder()
and
* subsequent calls to configure the result or SaveOptions.defaultOptions()
.
*
* The options map may be populated via {@link #toOptionsMap()} or {@link #addTo(Map)}.
*
* Clients are free to extend this interface and the respective builder implementation.
* @author Sebastian Zarnekow - Initial contribution and API
*/
public class SaveOptions {
protected static final String KEY = SaveOptions.class.getName();
private final boolean formatting;
private final boolean validating;
protected SaveOptions(boolean formatting, boolean validating) {
this.formatting = formatting;
this.validating = validating;
}
/**
* Transparently handles the deprecated options that could be passed as
* map-entries to {@link org.eclipse.emf.ecore.resource.Resource#save(Map)}
* and converts them to semantically equal {@link SaveOptions}.
*
* @param saveOptions the options-map or null
if none.
* @return the options to use. Will never return null
.
*/
@SuppressWarnings("deprecation")
public static SaveOptions getOptions(Map, ?> saveOptions) {
if (saveOptions == null || saveOptions.isEmpty())
return defaultOptions();
if (saveOptions.containsKey(KEY))
return (SaveOptions) saveOptions.get(KEY);
if (saveOptions.containsKey(XtextResource.OPTION_SERIALIZATION_OPTIONS))
return ((org.eclipse.xtext.parsetree.reconstr.SerializerOptions)
saveOptions.get(XtextResource.OPTION_SERIALIZATION_OPTIONS)).toSaveOptions();
if (Boolean.TRUE.equals(saveOptions.get(XtextResource.OPTION_FORMAT))) {
return newBuilder().format().getOptions();
}
return defaultOptions();
}
/**
* Configures the save options into the given map. The method modifies the
* given parameter so it may not be an ImmutableMap or a read-only Map.
* @param saveOptions the options to be modified. May not be null
.
*/
public void addTo(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy