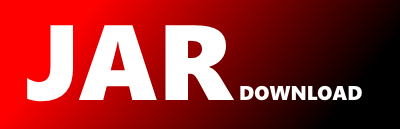
org.eclipse.xtext.util.XtextAdapterFactory Maven / Gradle / Ivy
/**
*
*
*
* $Id: XtextAdapterFactory.java,v 1.19 2010/04/06 14:10:25 sefftinge Exp $
*/
package org.eclipse.xtext.util;
import org.eclipse.emf.common.notify.Adapter;
import org.eclipse.emf.common.notify.Notifier;
import org.eclipse.emf.common.notify.impl.AdapterFactoryImpl;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.*;
/**
*
* The Adapter Factory for the model.
* It provides an adapter createXXX
method for each class of the model.
*
* @see org.eclipse.xtext.XtextPackage
* @generated
*/
public class XtextAdapterFactory extends AdapterFactoryImpl {
/**
* The cached model package.
*
*
* @generated
*/
protected static XtextPackage modelPackage;
/**
* Creates an instance of the adapter factory.
*
*
* @generated
*/
public XtextAdapterFactory() {
if (modelPackage == null) {
modelPackage = XtextPackage.eINSTANCE;
}
}
/**
* Returns whether this factory is applicable for the type of the object.
*
* This implementation returns true
if the object is either the model's package or is an instance object of the model.
*
* @return whether this factory is applicable for the type of the object.
* @generated
*/
@Override
public boolean isFactoryForType(Object object) {
if (object == modelPackage) {
return true;
}
if (object instanceof EObject) {
return ((EObject)object).eClass().getEPackage() == modelPackage;
}
return false;
}
/**
* The switch that delegates to the createXXX
methods.
*
*
* @generated
*/
protected XtextSwitch modelSwitch =
new XtextSwitch() {
@Override
public Adapter caseGrammar(Grammar object) {
return createGrammarAdapter();
}
@Override
public Adapter caseAbstractRule(AbstractRule object) {
return createAbstractRuleAdapter();
}
@Override
public Adapter caseAbstractMetamodelDeclaration(AbstractMetamodelDeclaration object) {
return createAbstractMetamodelDeclarationAdapter();
}
@Override
public Adapter caseGeneratedMetamodel(GeneratedMetamodel object) {
return createGeneratedMetamodelAdapter();
}
@Override
public Adapter caseReferencedMetamodel(ReferencedMetamodel object) {
return createReferencedMetamodelAdapter();
}
@Override
public Adapter caseParserRule(ParserRule object) {
return createParserRuleAdapter();
}
@Override
public Adapter caseTypeRef(TypeRef object) {
return createTypeRefAdapter();
}
@Override
public Adapter caseAbstractElement(AbstractElement object) {
return createAbstractElementAdapter();
}
@Override
public Adapter caseAction(Action object) {
return createActionAdapter();
}
@Override
public Adapter caseKeyword(Keyword object) {
return createKeywordAdapter();
}
@Override
public Adapter caseRuleCall(RuleCall object) {
return createRuleCallAdapter();
}
@Override
public Adapter caseAssignment(Assignment object) {
return createAssignmentAdapter();
}
@Override
public Adapter caseCrossReference(CrossReference object) {
return createCrossReferenceAdapter();
}
@Override
public Adapter caseTerminalRule(TerminalRule object) {
return createTerminalRuleAdapter();
}
@Override
public Adapter caseAbstractNegatedToken(AbstractNegatedToken object) {
return createAbstractNegatedTokenAdapter();
}
@Override
public Adapter caseNegatedToken(NegatedToken object) {
return createNegatedTokenAdapter();
}
@Override
public Adapter caseUntilToken(UntilToken object) {
return createUntilTokenAdapter();
}
@Override
public Adapter caseWildcard(Wildcard object) {
return createWildcardAdapter();
}
@Override
public Adapter caseEnumRule(EnumRule object) {
return createEnumRuleAdapter();
}
@Override
public Adapter caseEnumLiteralDeclaration(EnumLiteralDeclaration object) {
return createEnumLiteralDeclarationAdapter();
}
@Override
public Adapter caseAlternatives(Alternatives object) {
return createAlternativesAdapter();
}
@Override
public Adapter caseUnorderedGroup(UnorderedGroup object) {
return createUnorderedGroupAdapter();
}
@Override
public Adapter caseGroup(Group object) {
return createGroupAdapter();
}
@Override
public Adapter caseCharacterRange(CharacterRange object) {
return createCharacterRangeAdapter();
}
@Override
public Adapter caseCompoundElement(CompoundElement object) {
return createCompoundElementAdapter();
}
@Override
public Adapter caseEOF(EOF object) {
return createEOFAdapter();
}
@Override
public Adapter defaultCase(EObject object) {
return createEObjectAdapter();
}
};
/**
* Creates an adapter for the target
.
*
*
* @param target the object to adapt.
* @return the adapter for the target
.
* @generated
*/
@Override
public Adapter createAdapter(Notifier target) {
return modelSwitch.doSwitch((EObject)target);
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Grammar Grammar}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Grammar
* @generated
*/
public Adapter createGrammarAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.AbstractRule Abstract Rule}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.AbstractRule
* @generated
*/
public Adapter createAbstractRuleAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.AbstractMetamodelDeclaration Abstract Metamodel Declaration}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.AbstractMetamodelDeclaration
* @generated
*/
public Adapter createAbstractMetamodelDeclarationAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.GeneratedMetamodel Generated Metamodel}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.GeneratedMetamodel
* @generated
*/
public Adapter createGeneratedMetamodelAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.ReferencedMetamodel Referenced Metamodel}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.ReferencedMetamodel
* @generated
*/
public Adapter createReferencedMetamodelAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.ParserRule Parser Rule}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.ParserRule
* @generated
*/
public Adapter createParserRuleAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.TypeRef Type Ref}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.TypeRef
* @generated
*/
public Adapter createTypeRefAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.AbstractElement Abstract Element}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.AbstractElement
* @generated
*/
public Adapter createAbstractElementAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Action Action}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Action
* @generated
*/
public Adapter createActionAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Keyword Keyword}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Keyword
* @generated
*/
public Adapter createKeywordAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.RuleCall Rule Call}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.RuleCall
* @generated
*/
public Adapter createRuleCallAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Assignment Assignment}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Assignment
* @generated
*/
public Adapter createAssignmentAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.CrossReference Cross Reference}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.CrossReference
* @generated
*/
public Adapter createCrossReferenceAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.TerminalRule Terminal Rule}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.TerminalRule
* @generated
*/
public Adapter createTerminalRuleAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.AbstractNegatedToken Abstract Negated Token}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.AbstractNegatedToken
* @generated
*/
public Adapter createAbstractNegatedTokenAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.NegatedToken Negated Token}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.NegatedToken
* @generated
*/
public Adapter createNegatedTokenAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.UntilToken Until Token}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.UntilToken
* @generated
*/
public Adapter createUntilTokenAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Wildcard Wildcard}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Wildcard
* @generated
*/
public Adapter createWildcardAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.EOF EOF}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
* @since 2.0
*
* @return the new adapter.
* @see org.eclipse.xtext.EOF
* @generated
*/
public Adapter createEOFAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.EnumRule Enum Rule}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.EnumRule
* @generated
*/
public Adapter createEnumRuleAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.EnumLiteralDeclaration Enum Literal Declaration}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.EnumLiteralDeclaration
* @generated
*/
public Adapter createEnumLiteralDeclarationAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Alternatives Alternatives}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Alternatives
* @generated
*/
public Adapter createAlternativesAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.UnorderedGroup Unordered Group}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.UnorderedGroup
* @generated
*/
public Adapter createUnorderedGroupAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.Group Group}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.Group
* @generated
*/
public Adapter createGroupAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.CharacterRange Character Range}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.CharacterRange
* @generated
*/
public Adapter createCharacterRangeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link org.eclipse.xtext.CompoundElement Compound Element}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see org.eclipse.xtext.CompoundElement
* @generated
*/
public Adapter createCompoundElementAdapter() {
return null;
}
/**
* Creates a new adapter for the default case.
*
* This default implementation returns null.
*
* @return the new adapter.
* @generated
*/
public Adapter createEObjectAdapter() {
return null;
}
} //XtextAdapterFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy