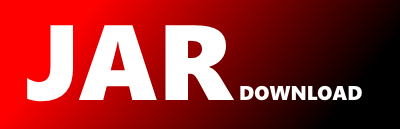
org.eclipse.xtext.util.XtextSwitch Maven / Gradle / Ivy
/**
*
*
*
* $Id: XtextSwitch.java,v 1.19 2010/04/06 14:10:25 sefftinge Exp $
*/
package org.eclipse.xtext.util;
import java.util.List;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.*;
/**
*
* The Switch for the model's inheritance hierarchy.
* It supports the call {@link #doSwitch(EObject) doSwitch(object)}
* to invoke the caseXXX
method for each class of the model,
* starting with the actual class of the object
* and proceeding up the inheritance hierarchy
* until a non-null result is returned,
* which is the result of the switch.
*
* @see org.eclipse.xtext.XtextPackage
* @generated
*/
public class XtextSwitch {
/**
* The cached model package
*
*
* @generated
*/
protected static XtextPackage modelPackage;
/**
* Creates an instance of the switch.
*
*
* @generated
*/
public XtextSwitch() {
if (modelPackage == null) {
modelPackage = XtextPackage.eINSTANCE;
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
public T doSwitch(EObject theEObject) {
return doSwitch(theEObject.eClass(), theEObject);
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(EClass theEClass, EObject theEObject) {
if (theEClass.eContainer() == modelPackage) {
return doSwitch(theEClass.getClassifierID(), theEObject);
}
else {
List eSuperTypes = theEClass.getESuperTypes();
return
eSuperTypes.isEmpty() ?
defaultCase(theEObject) :
doSwitch(eSuperTypes.get(0), theEObject);
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(int classifierID, EObject theEObject) {
switch (classifierID) {
case XtextPackage.GRAMMAR: {
Grammar grammar = (Grammar)theEObject;
T result = caseGrammar(grammar);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ABSTRACT_RULE: {
AbstractRule abstractRule = (AbstractRule)theEObject;
T result = caseAbstractRule(abstractRule);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ABSTRACT_METAMODEL_DECLARATION: {
AbstractMetamodelDeclaration abstractMetamodelDeclaration = (AbstractMetamodelDeclaration)theEObject;
T result = caseAbstractMetamodelDeclaration(abstractMetamodelDeclaration);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.GENERATED_METAMODEL: {
GeneratedMetamodel generatedMetamodel = (GeneratedMetamodel)theEObject;
T result = caseGeneratedMetamodel(generatedMetamodel);
if (result == null) result = caseAbstractMetamodelDeclaration(generatedMetamodel);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.REFERENCED_METAMODEL: {
ReferencedMetamodel referencedMetamodel = (ReferencedMetamodel)theEObject;
T result = caseReferencedMetamodel(referencedMetamodel);
if (result == null) result = caseAbstractMetamodelDeclaration(referencedMetamodel);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.PARSER_RULE: {
ParserRule parserRule = (ParserRule)theEObject;
T result = caseParserRule(parserRule);
if (result == null) result = caseAbstractRule(parserRule);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.TYPE_REF: {
TypeRef typeRef = (TypeRef)theEObject;
T result = caseTypeRef(typeRef);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ABSTRACT_ELEMENT: {
AbstractElement abstractElement = (AbstractElement)theEObject;
T result = caseAbstractElement(abstractElement);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ACTION: {
Action action = (Action)theEObject;
T result = caseAction(action);
if (result == null) result = caseAbstractElement(action);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.KEYWORD: {
Keyword keyword = (Keyword)theEObject;
T result = caseKeyword(keyword);
if (result == null) result = caseAbstractElement(keyword);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.RULE_CALL: {
RuleCall ruleCall = (RuleCall)theEObject;
T result = caseRuleCall(ruleCall);
if (result == null) result = caseAbstractElement(ruleCall);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ASSIGNMENT: {
Assignment assignment = (Assignment)theEObject;
T result = caseAssignment(assignment);
if (result == null) result = caseAbstractElement(assignment);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.CROSS_REFERENCE: {
CrossReference crossReference = (CrossReference)theEObject;
T result = caseCrossReference(crossReference);
if (result == null) result = caseAbstractElement(crossReference);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.TERMINAL_RULE: {
TerminalRule terminalRule = (TerminalRule)theEObject;
T result = caseTerminalRule(terminalRule);
if (result == null) result = caseAbstractRule(terminalRule);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ABSTRACT_NEGATED_TOKEN: {
AbstractNegatedToken abstractNegatedToken = (AbstractNegatedToken)theEObject;
T result = caseAbstractNegatedToken(abstractNegatedToken);
if (result == null) result = caseAbstractElement(abstractNegatedToken);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.NEGATED_TOKEN: {
NegatedToken negatedToken = (NegatedToken)theEObject;
T result = caseNegatedToken(negatedToken);
if (result == null) result = caseAbstractNegatedToken(negatedToken);
if (result == null) result = caseAbstractElement(negatedToken);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.UNTIL_TOKEN: {
UntilToken untilToken = (UntilToken)theEObject;
T result = caseUntilToken(untilToken);
if (result == null) result = caseAbstractNegatedToken(untilToken);
if (result == null) result = caseAbstractElement(untilToken);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.WILDCARD: {
Wildcard wildcard = (Wildcard)theEObject;
T result = caseWildcard(wildcard);
if (result == null) result = caseAbstractElement(wildcard);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ENUM_RULE: {
EnumRule enumRule = (EnumRule)theEObject;
T result = caseEnumRule(enumRule);
if (result == null) result = caseAbstractRule(enumRule);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ENUM_LITERAL_DECLARATION: {
EnumLiteralDeclaration enumLiteralDeclaration = (EnumLiteralDeclaration)theEObject;
T result = caseEnumLiteralDeclaration(enumLiteralDeclaration);
if (result == null) result = caseAbstractElement(enumLiteralDeclaration);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.ALTERNATIVES: {
Alternatives alternatives = (Alternatives)theEObject;
T result = caseAlternatives(alternatives);
if (result == null) result = caseCompoundElement(alternatives);
if (result == null) result = caseAbstractElement(alternatives);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.UNORDERED_GROUP: {
UnorderedGroup unorderedGroup = (UnorderedGroup)theEObject;
T result = caseUnorderedGroup(unorderedGroup);
if (result == null) result = caseCompoundElement(unorderedGroup);
if (result == null) result = caseAbstractElement(unorderedGroup);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.GROUP: {
Group group = (Group)theEObject;
T result = caseGroup(group);
if (result == null) result = caseCompoundElement(group);
if (result == null) result = caseAbstractElement(group);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.CHARACTER_RANGE: {
CharacterRange characterRange = (CharacterRange)theEObject;
T result = caseCharacterRange(characterRange);
if (result == null) result = caseAbstractElement(characterRange);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.COMPOUND_ELEMENT: {
CompoundElement compoundElement = (CompoundElement)theEObject;
T result = caseCompoundElement(compoundElement);
if (result == null) result = caseAbstractElement(compoundElement);
if (result == null) result = defaultCase(theEObject);
return result;
}
case XtextPackage.EOF: {
EOF eof = (EOF)theEObject;
T result = caseEOF(eof);
if (result == null) result = caseAbstractElement(eof);
if (result == null) result = defaultCase(theEObject);
return result;
}
default: return defaultCase(theEObject);
}
}
/**
* Returns the result of interpreting the object as an instance of 'Grammar'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Grammar'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGrammar(Grammar object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Abstract Rule'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Abstract Rule'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAbstractRule(AbstractRule object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Abstract Metamodel Declaration'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Abstract Metamodel Declaration'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAbstractMetamodelDeclaration(AbstractMetamodelDeclaration object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generated Metamodel'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generated Metamodel'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGeneratedMetamodel(GeneratedMetamodel object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Referenced Metamodel'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Referenced Metamodel'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseReferencedMetamodel(ReferencedMetamodel object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Parser Rule'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Parser Rule'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseParserRule(ParserRule object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Type Ref'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Type Ref'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseTypeRef(TypeRef object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Abstract Element'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Abstract Element'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAbstractElement(AbstractElement object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Action'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Action'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAction(Action object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Keyword'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Keyword'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseKeyword(Keyword object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Rule Call'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Rule Call'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRuleCall(RuleCall object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Assignment'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Assignment'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssignment(Assignment object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Cross Reference'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Cross Reference'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseCrossReference(CrossReference object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Terminal Rule'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Terminal Rule'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseTerminalRule(TerminalRule object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Abstract Negated Token'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Abstract Negated Token'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAbstractNegatedToken(AbstractNegatedToken object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Negated Token'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Negated Token'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseNegatedToken(NegatedToken object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Until Token'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Until Token'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseUntilToken(UntilToken object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Wildcard'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Wildcard'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseWildcard(Wildcard object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EOF'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
* @since 2.0
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'EOF'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEOF(EOF object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Enum Rule'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Enum Rule'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEnumRule(EnumRule object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Enum Literal Declaration'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Enum Literal Declaration'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEnumLiteralDeclaration(EnumLiteralDeclaration object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Alternatives'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Alternatives'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAlternatives(Alternatives object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Unordered Group'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Unordered Group'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseUnorderedGroup(UnorderedGroup object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Group'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Group'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGroup(Group object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Character Range'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Character Range'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseCharacterRange(CharacterRange object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Compound Element'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Compound Element'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseCompoundElement(CompoundElement object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EObject'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch, but this is the last case anyway.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'EObject'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject)
* @generated
*/
public T defaultCase(EObject object) {
return null;
}
} //XtextSwitch
© 2015 - 2025 Weber Informatics LLC | Privacy Policy