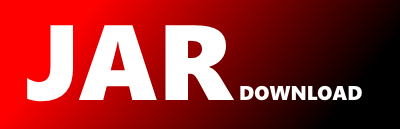
org.eclipse.xtext.validation.ValidationMessageAcceptor Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.validation;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EStructuralFeature;
/**
* A validation message acceptor provides the minimal necessary API to create
* error and warning messages that are associated with a feature value or with
* a range in the input source.
*
* Partial implementations will usually inherit from {@link AbstractValidationMessageAcceptor}.
*
* @author Sebastian Zarnekow - initial contribution and API
*/
public interface ValidationMessageAcceptor {
int INSIGNIFICANT_INDEX = -1;
/**
* Annotate an instance, a feature value, or all feature values with an error.
* @param message the error message. May not be null
.
* @param object the object or the feature holder. May not be null
.
* @param feature the feature or null
if the complete instance should be annotated.
* @param index the index of the erroneous value or -1
if all values are considered to be invalid. The index is ignored if
* the feature is null or the feature is a single value feature.
* @param code the optional issue code.
* @param issueData the optional issue data.
*/
void acceptError(String message, EObject object, EStructuralFeature feature, int index, String code, String...issueData);
/**
* Annotate a range of the resource with an error.
* @param message the error message. May not be null
.
* @param offset the absolute offset in the resource.
* @param length the length of the erroneous range.
* @param code the optional issue code.
* @param issueData the optional issue data.
* @throws IndexOutOfBoundsException if the offset or the length is invalid.
*/
void acceptError(String message, EObject object, int offset, int length, String code, String...issueData);
/**
* Annotate an instance, a feature value, or all feature values with a warning.
* @param message the warning message. May not be null
.
* @param object the object or the feature holder. May not be null
.
* @param feature the feature or null
if the complete instance should be annotated.
* @param index the index of the relevant value or -1
if all values are considered to be affected. The index is ignored if
* the feature is null or the feature is a single value feature.
* @param code the optional issue code.
* @param issueData the optional issue data.
*/
void acceptWarning(String message, EObject object, EStructuralFeature feature, int index, String code, String... issueData);
/**
* Annotate a range of the resource with a warning.
* @param message the warning message. May not be null
.
* @param offset the absolute offset in the resource.
* @param length the length of the invalid range.
* @param code the optional issue code.
* @param issueData the optional issue data.
* @throws IndexOutOfBoundsException if the offset or the length is invalid.
*/
void acceptWarning(String message, EObject object, int offset, int length, String code, String... issueData);
/**
* Annotate an instance, a feature value, or all feature values with an info message.
* @param message the info message. May not be null
.
* @param object the object or the feature holder. May not be null
.
* @param feature the feature or null
if the complete instance should be annotated.
* @param index the index of the interesting value or -1
if all values are considered to be interesting. The index is ignored if
* the feature is null or the feature is a single value feature.
* @param code the optional issue code.
* @param issueData the optional issue data.
*/
void acceptInfo(String message, EObject object, EStructuralFeature feature, int index, String code, String... issueData);
/**
* Annotate a range of the resource with an info message..
* @param message the info message. May not be null
.
* @param offset the absolute offset in the resource.
* @param length the length of the annotated range.
* @param code the optional issue code.
* @param issueData the optional issue data.
* @throws IndexOutOfBoundsException if the offset or the length is invalid.
*/
void acceptInfo(String message, EObject object, int offset, int length, String code, String... issueData);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy