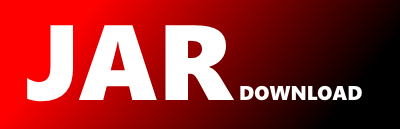
org.eclipse.xtext.xbase.XbaseFactory Maven / Gradle / Ivy
/**
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*/
package org.eclipse.xtext.xbase;
import org.eclipse.emf.ecore.EFactory;
/**
*
* The Factory for the model.
* It provides a create method for each non-abstract class of the model.
*
* @see org.eclipse.xtext.xbase.XbasePackage
* @generated
*/
public interface XbaseFactory extends EFactory
{
/**
* The singleton instance of the factory.
*
*
* @generated
*/
XbaseFactory eINSTANCE = org.eclipse.xtext.xbase.impl.XbaseFactoryImpl.init();
/**
* Returns a new object of class 'XIf Expression'.
*
*
* @return a new object of class 'XIf Expression'.
* @generated
*/
XIfExpression createXIfExpression();
/**
* Returns a new object of class 'XSwitch Expression'.
*
*
* @return a new object of class 'XSwitch Expression'.
* @generated
*/
XSwitchExpression createXSwitchExpression();
/**
* Returns a new object of class 'XCase Part'.
*
*
* @return a new object of class 'XCase Part'.
* @generated
*/
XCasePart createXCasePart();
/**
* Returns a new object of class 'XBlock Expression'.
*
*
* @return a new object of class 'XBlock Expression'.
* @generated
*/
XBlockExpression createXBlockExpression();
/**
* Returns a new object of class 'XVariable Declaration'.
*
*
* @return a new object of class 'XVariable Declaration'.
* @generated
*/
XVariableDeclaration createXVariableDeclaration();
/**
* Returns a new object of class 'XMember Feature Call'.
*
*
* @return a new object of class 'XMember Feature Call'.
* @generated
*/
XMemberFeatureCall createXMemberFeatureCall();
/**
* Returns a new object of class 'XFeature Call'.
*
*
* @return a new object of class 'XFeature Call'.
* @generated
*/
XFeatureCall createXFeatureCall();
/**
* Returns a new object of class 'XConstructor Call'.
*
*
* @return a new object of class 'XConstructor Call'.
* @generated
*/
XConstructorCall createXConstructorCall();
/**
* Returns a new object of class 'XBoolean Literal'.
*
*
* @return a new object of class 'XBoolean Literal'.
* @generated
*/
XBooleanLiteral createXBooleanLiteral();
/**
* Returns a new object of class 'XNull Literal'.
*
*
* @return a new object of class 'XNull Literal'.
* @generated
*/
XNullLiteral createXNullLiteral();
/**
* Returns a new object of class 'XNumber Literal'.
*
*
* @return a new object of class 'XNumber Literal'.
* @generated
*/
XNumberLiteral createXNumberLiteral();
/**
* Returns a new object of class 'XString Literal'.
*
*
* @return a new object of class 'XString Literal'.
* @generated
*/
XStringLiteral createXStringLiteral();
/**
* Returns a new object of class 'XList Literal'.
*
*
* @return a new object of class 'XList Literal'.
* @generated
*/
XListLiteral createXListLiteral();
/**
* Returns a new object of class 'XSet Literal'.
*
*
* @return a new object of class 'XSet Literal'.
* @generated
*/
XSetLiteral createXSetLiteral();
/**
* Returns a new object of class 'XClosure'.
*
*
* @return a new object of class 'XClosure'.
* @generated
*/
XClosure createXClosure();
/**
* Returns a new object of class 'XCasted Expression'.
*
*
* @return a new object of class 'XCasted Expression'.
* @generated
*/
XCastedExpression createXCastedExpression();
/**
* Returns a new object of class 'XBinary Operation'.
*
*
* @return a new object of class 'XBinary Operation'.
* @generated
*/
XBinaryOperation createXBinaryOperation();
/**
* Returns a new object of class 'XUnary Operation'.
*
*
* @return a new object of class 'XUnary Operation'.
* @generated
*/
XUnaryOperation createXUnaryOperation();
/**
* Returns a new object of class 'XFor Loop Expression'.
*
*
* @return a new object of class 'XFor Loop Expression'.
* @generated
*/
XForLoopExpression createXForLoopExpression();
/**
* Returns a new object of class 'XDo While Expression'.
*
*
* @return a new object of class 'XDo While Expression'.
* @generated
*/
XDoWhileExpression createXDoWhileExpression();
/**
* Returns a new object of class 'XWhile Expression'.
*
*
* @return a new object of class 'XWhile Expression'.
* @generated
*/
XWhileExpression createXWhileExpression();
/**
* Returns a new object of class 'XType Literal'.
*
*
* @return a new object of class 'XType Literal'.
* @generated
*/
XTypeLiteral createXTypeLiteral();
/**
* Returns a new object of class 'XInstance Of Expression'.
*
*
* @return a new object of class 'XInstance Of Expression'.
* @generated
*/
XInstanceOfExpression createXInstanceOfExpression();
/**
* Returns a new object of class 'XThrow Expression'.
*
*
* @return a new object of class 'XThrow Expression'.
* @generated
*/
XThrowExpression createXThrowExpression();
/**
* Returns a new object of class 'XTry Catch Finally Expression'.
*
*
* @return a new object of class 'XTry Catch Finally Expression'.
* @generated
*/
XTryCatchFinallyExpression createXTryCatchFinallyExpression();
/**
* Returns a new object of class 'XCatch Clause'.
*
*
* @return a new object of class 'XCatch Clause'.
* @generated
*/
XCatchClause createXCatchClause();
/**
* Returns a new object of class 'XAssignment'.
*
*
* @return a new object of class 'XAssignment'.
* @generated
*/
XAssignment createXAssignment();
/**
* Returns a new object of class 'XReturn Expression'.
*
*
* @return a new object of class 'XReturn Expression'.
* @generated
*/
XReturnExpression createXReturnExpression();
/**
* Returns the package supported by this factory.
*
*
* @return the package supported by this factory.
* @generated
*/
XbasePackage getXbasePackage();
} //XbaseFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy