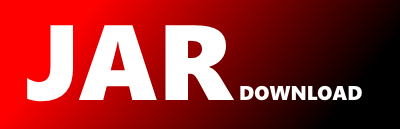
org.eclipse.xtext.xbase.compiler.LoopExtensions Maven / Gradle / Ivy
/**
* Copyright (c) 2012 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.eclipse.xtext.xbase.compiler;
import org.eclipse.xtext.xbase.compiler.LoopParams;
import org.eclipse.xtext.xbase.compiler.output.ITreeAppendable;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ObjectExtensions;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
/**
* @author Jan Koehnlein
*/
@SuppressWarnings("all")
public class LoopExtensions {
/**
* Iterates elements and execute the procedure.
* A prefix, a separator and a suffix can be initialized with the loopInitializer lambda.
*/
public void forEach(final ITreeAppendable appendable, final Iterable elements, final Procedure1 super LoopParams> loopInitializer, final Procedure1 super T> procedure) {
boolean _isEmpty = IterableExtensions.isEmpty(elements);
if (_isEmpty) {
return;
}
LoopParams _loopParams = new LoopParams();
final LoopParams params = ObjectExtensions.operator_doubleArrow(_loopParams, loopInitializer);
params.appendPrefix(appendable);
T _head = IterableExtensions.head(elements);
ObjectExtensions.operator_doubleArrow(_head, procedure);
Iterable _tail = IterableExtensions.tail(elements);
final Procedure1 _function = new Procedure1() {
public void apply(final T it) {
params.appendSeparator(appendable);
ObjectExtensions.operator_doubleArrow(it, procedure);
}
};
IterableExtensions.forEach(_tail, _function);
params.appendSuffix(appendable);
}
/**
* Uses curly braces and comma as delimiters. Doesn't use them for single valued iterables.
*/
public void forEachWithShortcut(final ITreeAppendable appendable, final Iterable elements, final Procedure1 super T> procedure) {
int _size = IterableExtensions.size(elements);
boolean _equals = (_size == 1);
if (_equals) {
T _head = IterableExtensions.head(elements);
ObjectExtensions.operator_doubleArrow(_head, procedure);
} else {
final Procedure1 _function = new Procedure1() {
public void apply(final LoopParams it) {
it.setPrefix("{ ");
it.setSeparator(", ");
it.setSuffix(" }");
}
};
this.forEach(appendable, elements, _function, procedure);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy