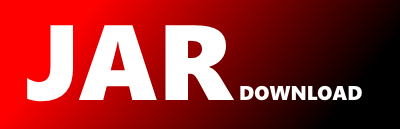
org.eclipse.xtext.xbase.lib.BigIntegerExtensions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.lib;
import java.math.BigInteger;
import com.google.common.annotations.GwtCompatible;
/**
* This is an extension library for {@link BigInteger big integral numbers}.
*
* @author Jan Koehnlein - Initial contribution and API
*/
@GwtCompatible public class BigIntegerExtensions {
/**
* The unary minus
operator.
*
* @param a
* a BigInteger. May not be null
.
* @return -a
* @throws NullPointerException
* if {@code a} is null
.
*/
@Inline(value="$1.negate()")
@Pure
public static BigInteger operator_minus(BigInteger a) {
return a.negate();
}
/**
* The binary plus
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param b
* a BigInteger. May not be null
.
* @return a.add(b)
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Inline(value="$1.add($2)")
@Pure
public static BigInteger operator_plus(BigInteger a, BigInteger b) {
return a.add(b);
}
/**
* The binary minus
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param b
* a BigInteger. May not be null
.
* @return a.subtract(b)
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Inline(value="$1.subtract($2)")
@Pure
public static BigInteger operator_minus(BigInteger a, BigInteger b) {
return a.subtract(b);
}
/**
* The power
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param exponent
* the exponent.
* @return a.pow(b)
* @throws NullPointerException
* if {@code a} null
.
*/
@Inline(value="$1.pow($2)")
@Pure
public static BigInteger operator_power(BigInteger a, int exponent) {
return a.pow(exponent);
}
/**
* The binary times
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param b
* a BigInteger. May not be null
.
* @return a.multiply(b)
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Inline(value="$1.multiply($2)")
@Pure
public static BigInteger operator_multiply(BigInteger a, BigInteger b) {
return a.multiply(b);
}
/**
* The binary divide
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param b
* a BigInteger. May not be null
.
* @return a.divide(b)
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Inline(value="$1.divide($2)")
@Pure
public static BigInteger operator_divide(BigInteger a, BigInteger b) {
return a.divide(b);
}
/**
* The binary modulo
operator.
*
* @param a
* a BigInteger. May not be null
.
* @param b
* a BigInteger. May not be null
.
* @return a.mod(b)
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Inline(value="$1.mod($2)")
@Pure
public static BigInteger operator_modulo(BigInteger a, BigInteger b) {
return a.mod(b);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy