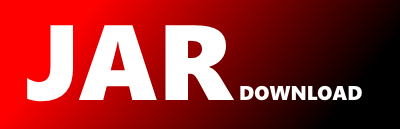
org.eclipse.xtext.xbase.lib.BooleanExtensions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.lib;
import com.google.common.annotations.GwtCompatible;
import com.google.common.primitives.Booleans;
/**
* This is an extension library for {@link Boolean booleans}.
*
* @author Sven Efftinge - Initial contribution and API
*/
@GwtCompatible public class BooleanExtensions {
/**
* The logical and
(conjunction). This is the equivalent to the java &&
operator.
*
* @param a
* a boolean value.
* @param b
* another boolean value.
* @return a && b
*/
@Pure
@Inline("($1 && $2)")
public static boolean operator_and(boolean a, boolean b) {
return a && b;
}
/**
* A logical or
(disjunction). This is the equivalent to the java ||
operator.
*
* @param a
* a boolean value.
* @param b
* another boolean value.
* @return a || b
*/
@Pure
@Inline("($1 || $2)")
public static boolean operator_or(boolean a, boolean b) {
return a || b;
}
/**
* The logical not
(negation). This is the equivalent to the java !
operator.
*
* @param b
* a boolean value.
* @return !b
*/
@Pure
@Inline("(!$1)")
public static boolean operator_not(boolean b) {
return !b;
}
/**
* The binary equals
operator. This is the equivalent to the java ==
operator.
*
* @param a
* an boolean.
* @param b
* an boolean.
* @return a==b
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(boolean a, boolean b) {
return a == b;
}
/**
* The binary not equals
operator. This is the equivalent to the java !=
operator.
*
* @param a
* an boolean.
* @param b
* an boolean.
* @return a!=b
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(boolean a, boolean b) {
return a != b;
}
/**
* A logical xor
. This is the equivalent to the java ^
operator.
*
* @param a
* a boolean value.
* @param b
* another boolean value.
* @return a ^ b
*/
@Pure
@Inline("($1 ^ $2)")
public static boolean xor(boolean a, boolean b) {
return a ^ b;
}
/**
* The binary lessThan
operator for boolean values.
* {@code false} is considered less than {@code true}.
*
* @see Boolean#compareTo(Boolean)
* @see Booleans#compare(boolean, boolean)
* @param a a boolean.
* @param b another boolean.
* @return Booleans.compare(a, b)<0
* @since 2.4
*/
@Pure
@Inline(value = "($3.compare($1, $2) < 0)", imported = Booleans.class)
public static boolean operator_lessThan(boolean a, boolean b) {
return Booleans.compare(a, b) < 0;
}
/**
* The binary lessEqualsThan
operator for boolean values.
* {@code false} is considered less than {@code true}.
*
* @see Boolean#compareTo(Boolean)
* @see Booleans#compare(boolean, boolean)
* @param a a boolean.
* @param b another boolean.
* @return Booleans.compare(a, b)<=0
* @since 2.4
*/
@Pure
@Inline(value = "($3.compare($1, $2) <= 0)", imported = Booleans.class)
public static boolean operator_lessEqualsThan(boolean a, boolean b) {
return Booleans.compare(a, b) <= 0;
}
/**
* The binary greaterThan
operator for boolean values.
* {@code false} is considered less than {@code true}.
*
* @see Boolean#compareTo(Boolean)
* @see Booleans#compare(boolean, boolean)
* @param a a boolean.
* @param b another boolean.
* @return Booleans.compare(a, b)>0
* @since 2.4
*/
@Pure
@Inline(value = "($3.compare($1, $2) > 0)", imported = Booleans.class)
public static boolean operator_greaterThan(boolean a, boolean b) {
return Booleans.compare(a, b) > 0;
}
/**
* The binary greaterEqualsThan
operator for boolean values.
* {@code false} is considered less than {@code true}.
*
* @see Boolean#compareTo(Boolean)
* @see Booleans#compare(boolean, boolean)
* @param a a boolean.
* @param b another boolean.
* @return Booleans.compare(a, b)>=0
* @since 2.4
*/
@Pure
@Inline(value = "($3.compare($1, $2) >= 0)", imported = Booleans.class)
public static boolean operator_greaterEqualsThan(boolean a, boolean b) {
return Booleans.compare(a, b) >= 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy