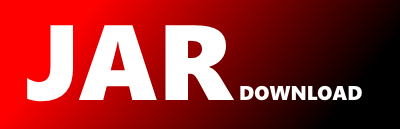
org.eclipse.xtext.xbase.lib.ComparableExtensions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.lib;
import com.google.common.annotations.GwtCompatible;
/**
* This is an extension library for {@link Comparable comparables}.
*
* @author Sven Efftinge - Initial contribution and API
*/
@GwtCompatible public class ComparableExtensions {
/**
* The comparison operator less than
.
*
* @param left
* a comparable
* @param right
* the value to compare with
* @return left.compareTo(right) < 0
*/
@Inline("($1.compareTo($2) < 0)")
public static boolean operator_lessThan(Comparable super C> left, C right) {
return left.compareTo(right) < 0;
}
/**
* The comparison operator greater than
.
*
* @param left
* a comparable
* @param right
* the value to compare with
* @return left.compareTo(right) > 0
*/
@Inline("($1.compareTo($2) > 0)")
public static boolean operator_greaterThan(Comparable super C> left, C right) {
return left.compareTo(right) > 0;
}
/**
* The comparison operator less than or equals
.
*
* @param left
* a comparable
* @param right
* the value to compare with
* @return left.compareTo(right) <= 0
*/
@Inline("($1.compareTo($2) <= 0)")
public static boolean operator_lessEqualsThan(Comparable super C> left, C right) {
return left.compareTo(right) <= 0;
}
/**
* The comparison operator greater than or equals
.
*
* @param left
* a comparable
* @param right
* the value to compare with
* @return left.compareTo(right) >= 0
*/
@Inline("($1.compareTo($2) >= 0)")
public static boolean operator_greaterEqualsThan(Comparable super C> left, C right) {
return left.compareTo(right) >= 0;
}
/**
* The spaceship operator <=>
.
*
* @param left
* a comparable
* @param right
* the value to compare with
* @return left.compareTo(right)
* @since 2.4
*/
@Inline("($1.compareTo($2))")
public static int operator_spaceship(Comparable super C> left, C right) {
return left.compareTo(right);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy