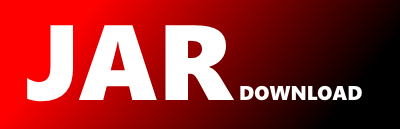
org.eclipse.xtext.xbase.lib.DoubleExtensions Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.lib;
import com.google.common.annotations.GwtCompatible;
/**
* This is an extension library for {@link Double floating point numbers}, e.g. double
or Double
.
*
* @author Jan Koehnlein - Initial contribution and API
*/
@GwtCompatible public class DoubleExtensions {
/**
* The unary minus
operator. This is the equivalent to the unary java -
operator.
*
* @param a
* a double. May not be null
.
* @return -a
* @throws NullPointerException
* if {@code a} is null
.
*/
@Pure
public static double operator_minus(Double a) {
return -a;
}
/**
* The binary plus
operator. This is the equivalent to the java +
operator.
*
* @param a
* a double. May not be null
.
* @param b
* a number. May not be null
.
* @return a+b
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Pure
public static double operator_plus(Double a, Number b) {
return a + b.doubleValue();
}
/**
* The binary minus
operator. This is the equivalent to the java -
operator.
*
* @param a
* a double. May not be null
.
* @param b
* a number. May not be null
.
* @return a-b
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Pure
public static double operator_minus(Double a, Number b) {
return a - b.doubleValue();
}
/**
* The power
operator.
*
* @param a
* a double. May not be null
.
* @param b
* a number. May not be null
.
* @return a ** b
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Pure
public static double operator_power(Double a, Number b) {
return Math.pow(a, b.doubleValue());
}
/**
* The binary times
operator. This is the equivalent to the java *
operator.
*
* @param a
* a double. May not be null
.
* @param b
* a number. May not be null
.
* @return a*b
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
public static double operator_multiply(Double a, Number b) {
return a * b.doubleValue();
}
/**
* The binary divide
operator. This is the equivalent to the java /
operator. That is, the
* result will be the integral result of the division, e.g. operator_divide(1, 2)
yields 0
* .
*
* @param a
* a double. May not be null
.
* @param b
* a number. May not be null
.
* @return a/b
* @throws NullPointerException
* if {@code a} or {@code b} is null
.
*/
@Pure
public static double operator_divide(Double a, Number b) {
return a / b.doubleValue();
}
// BEGIN generated code
/**
* The unary minus
operator. This is the equivalent to the Java's -
function.
*
* @param a a double.
* @return -a
* @since 2.3
*/
@Pure
@Inline("(-$1)")
public static double operator_minus(double a) {
return -a;
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a double.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, double b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a double.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, double b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a double.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, double b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a double.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, double b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a double.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, double b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a double.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, double b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a double.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, double b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a double.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, double b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a double.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, double b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a double.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, double b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a double.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, double b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a double.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, double b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a float.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, float b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a float.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, float b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a float.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, float b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a float.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, float b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a float.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, float b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a float.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, float b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a float.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, float b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a float.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, float b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a float.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, float b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a float.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, float b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a float.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, float b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a float.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, float b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a long.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, long b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a long.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, long b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a long.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, long b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a long.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, long b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a long.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, long b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a long.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, long b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a long.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, long b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a long.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, long b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a long.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, long b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a long.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, long b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a long.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, long b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a long.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, long b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b an integer.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, int b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b an integer.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, int b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b an integer.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, int b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b an integer.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, int b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b an integer.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, int b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b an integer.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, int b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b an integer.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, int b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b an integer.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, int b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b an integer.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, int b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b an integer.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, int b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b an integer.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, int b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b an integer.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, int b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a character.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, char b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a character.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, char b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a character.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, char b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a character.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, char b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a character.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, char b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a character.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, char b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a character.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, char b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a character.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, char b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a character.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, char b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a character.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, char b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a character.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, char b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a character.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, char b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a short.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, short b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a short.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, short b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a short.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, short b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a short.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, short b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a short.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, short b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a short.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, short b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a short.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, short b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a short.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, short b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a short.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, short b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a short.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, short b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a short.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, short b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a short.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, short b) {
return Math.pow(a, b);
}
/**
* The binary plus
operator. This is the equivalent to the Java +
operator.
*
* @param a a double.
* @param b a byte.
* @return a+b
* @since 2.3
*/
@Pure
@Inline("($1 + $2)")
public static double operator_plus(double a, byte b) {
return a + b;
}
/**
* The binary minus
operator. This is the equivalent to the Java -
operator.
*
* @param a a double.
* @param b a byte.
* @return a-b
* @since 2.3
*/
@Pure
@Inline("($1 - $2)")
public static double operator_minus(double a, byte b) {
return a - b;
}
/**
* The binary multiply
operator. This is the equivalent to the Java *
operator.
*
* @param a a double.
* @param b a byte.
* @return a*b
* @since 2.3
*/
@Pure
@Inline("($1 * $2)")
public static double operator_multiply(double a, byte b) {
return a * b;
}
/**
* The binary divide
operator. This is the equivalent to the Java /
operator.
*
* @param a a double.
* @param b a byte.
* @return a/b
* @since 2.3
*/
@Pure
@Inline("($1 / $2)")
public static double operator_divide(double a, byte b) {
return a / b;
}
/**
* The binary modulo
operator. This is the equivalent to the Java %
operator.
*
* @param a a double.
* @param b a byte.
* @return a%b
* @since 2.3
*/
@Pure
@Inline("($1 % $2)")
public static double operator_modulo(double a, byte b) {
return a % b;
}
/**
* The binary lessThan
operator. This is the equivalent to the Java <
operator.
*
* @param a a double.
* @param b a byte.
* @return a<b
* @since 2.3
*/
@Pure
@Inline("($1 < $2)")
public static boolean operator_lessThan(double a, byte b) {
return a < b;
}
/**
* The binary lessEqualsThan
operator. This is the equivalent to the Java <=
operator.
*
* @param a a double.
* @param b a byte.
* @return a<=b
* @since 2.3
*/
@Pure
@Inline("($1 <= $2)")
public static boolean operator_lessEqualsThan(double a, byte b) {
return a <= b;
}
/**
* The binary greaterThan
operator. This is the equivalent to the Java >
operator.
*
* @param a a double.
* @param b a byte.
* @return a>b
* @since 2.3
*/
@Pure
@Inline("($1 > $2)")
public static boolean operator_greaterThan(double a, byte b) {
return a > b;
}
/**
* The binary greaterEqualsThan
operator. This is the equivalent to the Java >=
operator.
*
* @param a a double.
* @param b a byte.
* @return a>=b
* @since 2.3
*/
@Pure
@Inline("($1 >= $2)")
public static boolean operator_greaterEqualsThan(double a, byte b) {
return a >= b;
}
/**
* The binary equals
operator. This is the equivalent to the Java ==
operator.
*
* @param a a double.
* @param b a byte.
* @return a==b
* @since 2.3
*/
@Pure
@Inline("($1 == $2)")
public static boolean operator_equals(double a, byte b) {
return a == b;
}
/**
* The binary notEquals
operator. This is the equivalent to the Java !=
operator.
*
* @param a a double.
* @param b a byte.
* @return a!=b
* @since 2.3
*/
@Pure
@Inline("($1 != $2)")
public static boolean operator_notEquals(double a, byte b) {
return a != b;
}
/**
* The binary power
operator. This is the equivalent to the Java's Math.pow()
function.
*
* @param a a double.
* @param b a byte.
* @return Math.pow(a, b)
* @since 2.3
*/
@Pure
@Inline(value="$3.pow($1, $2)", imported=Math.class)
public static double operator_power(double a, byte b) {
return Math.pow(a, b);
}
// END generated code
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy