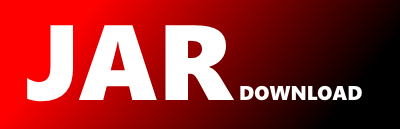
org.eclipse.xtext.xbase.lib.Pair Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.lib;
import com.google.common.annotations.GwtCompatible;
import com.google.common.base.Objects;
/**
* An immutable pair of {@link #getKey() key} and {@link #getValue() value}. A pair is considered to be
* {@link #equals(Object) equal} to another pair if both the key and the value are equal.
*
* @param
* the key-type of the pair.
* @param
* the value-type of the pair.
* @author Sebastian Zarnekow - Initial contribution and API
*/
@GwtCompatible public final class Pair {
private final K k;
private final V v;
/**
* Creates a new instance with the given key and value.
* May be used instead of the constructor for convenience reasons.
*
* @param k
* the key. May be null
.
* @param v
* the value. May be null
.
* @return a newly created pair. Never null
.
* @since 2.3
*/
@Pure
public static Pair of(K k, V v) {
return new Pair(k, v);
}
/**
* Creates a new instance with the given key and value.
*
* @param k
* the key. May be null
.
* @param v
* the value. May be null
.
*
*/
@Pure
public Pair(K k, V v) {
this.k = k;
this.v = v;
}
/**
* Returns the key.
*
* @return the key.
*/
@Pure
public K getKey() {
return k;
}
/**
* Returns the value.
*
* @return the value.
*/
@Pure
public V getValue() {
return v;
}
@Override
public boolean equals(Object o) {
if (o == this)
return true;
if (!(o instanceof Pair))
return false;
Pair, ?> e = (Pair, ?>) o;
return Objects.equal(k, e.getKey()) && Objects.equal(v, e.getValue());
}
@Override
public int hashCode() {
return ((k == null) ? 0 : k.hashCode()) ^ ((v == null) ? 0 : v.hashCode());
}
@Override
public String toString() {
return k + "->" + v;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy