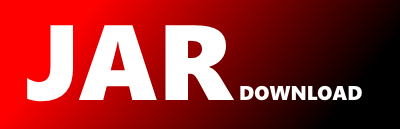
org.eclipse.xtext.scoping.impl.SimpleLocalScopeProvider Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2008 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.scoping.impl;
import java.util.Iterator;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.xtext.naming.IQualifiedNameProvider;
import org.eclipse.xtext.resource.IEObjectDescription;
import org.eclipse.xtext.resource.ISelectable;
import org.eclipse.xtext.scoping.IScope;
import org.eclipse.xtext.scoping.Scopes;
import org.eclipse.xtext.util.IResourceScopeCache;
import org.eclipse.xtext.util.Tuples;
import com.google.inject.Inject;
import com.google.inject.Provider;
/**
* A local scope that uses the qualified names of all elements of an {@link org.eclipse.emf.ecore.resource.Resource} and
* delegates to a global scope.
*
* @author Sven Efftinge - Initial contribution and API
*/
public class SimpleLocalScopeProvider extends AbstractGlobalScopeDelegatingScopeProvider {
@Inject
private IQualifiedNameProvider qualifiedNameProvider;
@Inject
private IResourceScopeCache cache;
public void setCache(IResourceScopeCache cache) {
this.cache = cache;
}
public void setNameProvider(IQualifiedNameProvider nameProvider) {
this.qualifiedNameProvider = nameProvider;
}
protected IQualifiedNameProvider getNameProvider() {
return qualifiedNameProvider;
}
public IScope getScope(final EObject context, final EReference reference) {
ISelectable resourceContent = cache.get(Tuples.pair(SimpleLocalScopeProvider.class.getName(), reference),
context.eResource(), new Provider() {
public ISelectable get() {
return getAllDescriptions(context.eResource());
}
});
IScope globalScope = getGlobalScope(context.eResource(), reference);
return createScope(globalScope, resourceContent, reference.getEReferenceType(), isIgnoreCase(reference));
}
protected IScope createScope(IScope parent, ISelectable resourceContent, EClass type, boolean ignoreCase) {
return SelectableBasedScope.createScope(parent, resourceContent, type, ignoreCase);
}
protected ISelectable getAllDescriptions(final Resource resource) {
Iterable allContents = new Iterable(){
public Iterator iterator() {
return resource.getAllContents();
}
};
Iterable allDescriptions = Scopes.scopedElementsFor(allContents, qualifiedNameProvider);
return new MultimapBasedSelectable(allDescriptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy