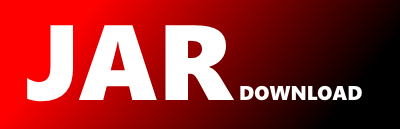
org.eclipse.xtext.service.MethodBasedModule Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.service;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.WildcardType;
import org.apache.log4j.Logger;
import com.google.inject.Binder;
import com.google.inject.Key;
import com.google.inject.Module;
import com.google.inject.Scopes;
import com.google.inject.binder.LinkedBindingBuilder;
/**
* @author Sebastian Zarnekow - Initial contribution and API
* @author Sven Efftinge
*/
public abstract class MethodBasedModule implements Module {
private static Logger LOGGER = Logger.getLogger(BindModule.class);
private final Method method;
private final Object owner;
protected MethodBasedModule(Method method, Object owner) {
this.method = method;
this.owner = owner;
}
public Method getMethod() {
return method;
}
public Object getOwner() {
return owner;
}
@SuppressWarnings("unchecked")
public void configure(Binder binder) {
Type key = getKeyType();
if (isClassBinding()) {
Class> value = (Class>) invokeMethod();
if (LOGGER.isTraceEnabled())
LOGGER.trace("Adding binding from " + key + " to " + value.getName()
+ ". Declaring Method was '" + getMethod().toGenericString() + "' in Module "
+ this.getClass().getName());
if (value != null && !Void.class.equals(value)) {
LinkedBindingBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy