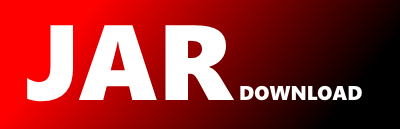
org.eclipse.xtext.service.ProviderModule Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.service;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import com.google.inject.Provider;
import com.google.inject.binder.LinkedBindingBuilder;
import com.google.inject.internal.MoreTypes;
public class ProviderModule extends MethodBasedModule {
public ProviderModule(Method method, Object owner) {
super(method, owner);
}
@SuppressWarnings("unchecked")
@Override
protected void bindToInstance(LinkedBindingBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy