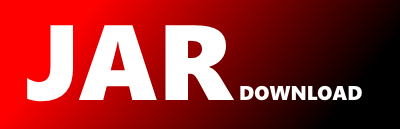
org.eclipse.xtext.xbase.XbasePackage Maven / Gradle / Ivy
/**
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*/
package org.eclipse.xtext.xbase;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
/**
*
* The Package for the model.
* It contains accessors for the meta objects to represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
*
* @since 2.7
* @noextend This interface is not intended to be extended by clients.
* @noimplement This interface is not intended to be implemented by clients.
*
* @see org.eclipse.xtext.xbase.XbaseFactory
* @model kind="package"
* @generated
*/
public interface XbasePackage extends EPackage
{
/**
* The package name.
*
*
* @generated
*/
String eNAME = "xbase";
/**
* The package namespace URI.
*
*
* @generated
*/
String eNS_URI = "http://www.eclipse.org/xtext/xbase/Xbase";
/**
* The package namespace name.
*
*
* @generated
*/
String eNS_PREFIX = "xbase";
/**
* The singleton instance of the package.
*
*
* @generated
*/
XbasePackage eINSTANCE = org.eclipse.xtext.xbase.impl.XbasePackageImpl.init();
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XExpressionImpl XExpression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXExpression()
* @generated
*/
int XEXPRESSION = 0;
/**
* The number of structural features of the 'XExpression' class.
*
*
* @generated
* @ordered
*/
int XEXPRESSION_FEATURE_COUNT = 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XIfExpressionImpl XIf Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XIfExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXIfExpression()
* @generated
*/
int XIF_EXPRESSION = 1;
/**
* The feature id for the 'If' containment reference.
*
*
* @generated
* @ordered
*/
int XIF_EXPRESSION__IF = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Then' containment reference.
*
*
* @generated
* @ordered
*/
int XIF_EXPRESSION__THEN = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Else' containment reference.
*
*
* @generated
* @ordered
*/
int XIF_EXPRESSION__ELSE = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The number of structural features of the 'XIf Expression' class.
*
*
* @generated
* @ordered
*/
int XIF_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XSwitchExpressionImpl XSwitch Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSwitchExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSwitchExpression()
* @generated
*/
int XSWITCH_EXPRESSION = 2;
/**
* The feature id for the 'Switch' containment reference.
*
*
* @generated
* @ordered
*/
int XSWITCH_EXPRESSION__SWITCH = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Cases' containment reference list.
*
*
* @generated
* @ordered
*/
int XSWITCH_EXPRESSION__CASES = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Default' containment reference.
*
*
* @generated
* @ordered
*/
int XSWITCH_EXPRESSION__DEFAULT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Declared Param' containment reference.
*
*
* @generated
* @ordered
*/
int XSWITCH_EXPRESSION__DECLARED_PARAM = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XSwitch Expression' class.
*
*
* @generated
* @ordered
*/
int XSWITCH_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XCasePartImpl XCase Part}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCasePartImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCasePart()
* @generated
*/
int XCASE_PART = 3;
/**
* The feature id for the 'Case' containment reference.
*
*
* @generated
* @ordered
*/
int XCASE_PART__CASE = 0;
/**
* The feature id for the 'Then' containment reference.
*
*
* @generated
* @ordered
*/
int XCASE_PART__THEN = 1;
/**
* The feature id for the 'Type Guard' containment reference.
*
*
* @generated
* @ordered
*/
int XCASE_PART__TYPE_GUARD = 2;
/**
* The feature id for the 'Fall Through' attribute.
*
*
* @generated
* @ordered
*/
int XCASE_PART__FALL_THROUGH = 3;
/**
* The number of structural features of the 'XCase Part' class.
*
*
* @generated
* @ordered
*/
int XCASE_PART_FEATURE_COUNT = 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XBlockExpressionImpl XBlock Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBlockExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBlockExpression()
* @generated
*/
int XBLOCK_EXPRESSION = 4;
/**
* The feature id for the 'Expressions' containment reference list.
*
*
* @generated
* @ordered
*/
int XBLOCK_EXPRESSION__EXPRESSIONS = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XBlock Expression' class.
*
*
* @generated
* @ordered
*/
int XBLOCK_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XVariableDeclarationImpl XVariable Declaration}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XVariableDeclarationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXVariableDeclaration()
* @generated
*/
int XVARIABLE_DECLARATION = 5;
/**
* The feature id for the 'Type' containment reference.
*
*
* @generated
* @ordered
*/
int XVARIABLE_DECLARATION__TYPE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Name' attribute.
*
*
* @generated
* @ordered
*/
int XVARIABLE_DECLARATION__NAME = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Right' containment reference.
*
*
* @generated
* @ordered
*/
int XVARIABLE_DECLARATION__RIGHT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Writeable' attribute.
*
*
* @generated
* @ordered
*/
int XVARIABLE_DECLARATION__WRITEABLE = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XVariable Declaration' class.
*
*
* @generated
* @ordered
*/
int XVARIABLE_DECLARATION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XAbstractFeatureCallImpl XAbstract Feature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAbstractFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAbstractFeatureCall()
* @generated
*/
int XABSTRACT_FEATURE_CALL = 6;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__FEATURE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__VALID_FEATURE = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT = XEXPRESSION_FEATURE_COUNT + 5;
/**
* The number of structural features of the 'XAbstract Feature Call' class.
*
*
* @generated
* @ordered
*/
int XABSTRACT_FEATURE_CALL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 6;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XMemberFeatureCallImpl XMember Feature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XMemberFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXMemberFeatureCall()
* @generated
*/
int XMEMBER_FEATURE_CALL = 7;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Member Call Target' containment reference.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__MEMBER_CALL_TARGET = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The feature id for the 'Member Call Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__MEMBER_CALL_ARGUMENTS = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The feature id for the 'Explicit Operation Call' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__EXPLICIT_OPERATION_CALL = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 2;
/**
* The feature id for the 'Explicit Static' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__EXPLICIT_STATIC = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 3;
/**
* The feature id for the 'Null Safe' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__NULL_SAFE = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 4;
/**
* The feature id for the 'Type Literal' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__TYPE_LITERAL = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 5;
/**
* The feature id for the 'Static With Declaring Type' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__STATIC_WITH_DECLARING_TYPE = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 6;
/**
* The feature id for the 'Package Fragment' attribute.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL__PACKAGE_FRAGMENT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 7;
/**
* The number of structural features of the 'XMember Feature Call' class.
*
*
* @generated
* @ordered
*/
int XMEMBER_FEATURE_CALL_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 8;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XFeatureCallImpl XFeature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXFeatureCall()
* @generated
*/
int XFEATURE_CALL = 8;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Feature Call Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__FEATURE_CALL_ARGUMENTS = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The feature id for the 'Explicit Operation Call' attribute.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__EXPLICIT_OPERATION_CALL = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The feature id for the 'Type Literal' attribute.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__TYPE_LITERAL = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 2;
/**
* The feature id for the 'Package Fragment' attribute.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL__PACKAGE_FRAGMENT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XFeature Call' class.
*
*
* @generated
* @ordered
*/
int XFEATURE_CALL_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XConstructorCallImpl XConstructor Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XConstructorCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXConstructorCall()
* @generated
*/
int XCONSTRUCTOR_CALL = 9;
/**
* The feature id for the 'Constructor' reference.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__CONSTRUCTOR = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__ARGUMENTS = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__TYPE_ARGUMENTS = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__INVALID_FEATURE_ISSUE_CODE = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__VALID_FEATURE = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The feature id for the 'Explicit Constructor Call' attribute.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__EXPLICIT_CONSTRUCTOR_CALL = XEXPRESSION_FEATURE_COUNT + 5;
/**
* The feature id for the 'Anonymous Class Constructor Call' attribute.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL__ANONYMOUS_CLASS_CONSTRUCTOR_CALL = XEXPRESSION_FEATURE_COUNT + 6;
/**
* The number of structural features of the 'XConstructor Call' class.
*
*
* @generated
* @ordered
*/
int XCONSTRUCTOR_CALL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 7;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XBooleanLiteralImpl XBoolean Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBooleanLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBooleanLiteral()
* @generated
*/
int XBOOLEAN_LITERAL = 10;
/**
* The feature id for the 'Is True' attribute.
*
*
* @generated
* @ordered
*/
int XBOOLEAN_LITERAL__IS_TRUE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XBoolean Literal' class.
*
*
* @generated
* @ordered
*/
int XBOOLEAN_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XNullLiteralImpl XNull Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XNullLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXNullLiteral()
* @generated
*/
int XNULL_LITERAL = 11;
/**
* The number of structural features of the 'XNull Literal' class.
*
*
* @generated
* @ordered
*/
int XNULL_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XNumberLiteralImpl XNumber Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XNumberLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXNumberLiteral()
* @generated
*/
int XNUMBER_LITERAL = 12;
/**
* The feature id for the 'Value' attribute.
*
*
* @generated
* @ordered
*/
int XNUMBER_LITERAL__VALUE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XNumber Literal' class.
*
*
* @generated
* @ordered
*/
int XNUMBER_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XStringLiteralImpl XString Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XStringLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXStringLiteral()
* @generated
*/
int XSTRING_LITERAL = 13;
/**
* The feature id for the 'Value' attribute.
*
*
* @generated
* @ordered
*/
int XSTRING_LITERAL__VALUE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XString Literal' class.
*
*
* @generated
* @ordered
*/
int XSTRING_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XCollectionLiteralImpl XCollection Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCollectionLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCollectionLiteral()
* @generated
*/
int XCOLLECTION_LITERAL = 14;
/**
* The feature id for the 'Elements' containment reference list.
*
*
* @generated
* @ordered
*/
int XCOLLECTION_LITERAL__ELEMENTS = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XCollection Literal' class.
*
*
* @generated
* @ordered
*/
int XCOLLECTION_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XListLiteralImpl XList Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XListLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXListLiteral()
* @generated
*/
int XLIST_LITERAL = 15;
/**
* The feature id for the 'Elements' containment reference list.
*
*
* @generated
* @ordered
*/
int XLIST_LITERAL__ELEMENTS = XCOLLECTION_LITERAL__ELEMENTS;
/**
* The number of structural features of the 'XList Literal' class.
*
*
* @generated
* @ordered
*/
int XLIST_LITERAL_FEATURE_COUNT = XCOLLECTION_LITERAL_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XSetLiteralImpl XSet Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSetLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSetLiteral()
* @generated
*/
int XSET_LITERAL = 16;
/**
* The feature id for the 'Elements' containment reference list.
*
*
* @generated
* @ordered
*/
int XSET_LITERAL__ELEMENTS = XCOLLECTION_LITERAL__ELEMENTS;
/**
* The number of structural features of the 'XSet Literal' class.
*
*
* @generated
* @ordered
*/
int XSET_LITERAL_FEATURE_COUNT = XCOLLECTION_LITERAL_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XClosureImpl XClosure}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XClosureImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXClosure()
* @generated
*/
int XCLOSURE = 17;
/**
* The feature id for the 'Declared Formal Parameters' containment reference list.
*
*
* @generated
* @ordered
*/
int XCLOSURE__DECLARED_FORMAL_PARAMETERS = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XCLOSURE__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Explicit Syntax' attribute.
*
*
* @generated
* @ordered
*/
int XCLOSURE__EXPLICIT_SYNTAX = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Implicit Formal Parameters' containment reference list.
*
*
* @generated
* @ordered
*/
int XCLOSURE__IMPLICIT_FORMAL_PARAMETERS = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XClosure' class.
*
*
* @generated
* @ordered
*/
int XCLOSURE_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XCastedExpressionImpl XCasted Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCastedExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCastedExpression()
* @generated
*/
int XCASTED_EXPRESSION = 18;
/**
* The feature id for the 'Type' containment reference.
*
*
* @generated
* @ordered
*/
int XCASTED_EXPRESSION__TYPE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Target' containment reference.
*
*
* @generated
* @ordered
*/
int XCASTED_EXPRESSION__TARGET = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'XCasted Expression' class.
*
*
* @generated
* @ordered
*/
int XCASTED_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XBinaryOperationImpl XBinary Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBinaryOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBinaryOperation()
* @generated
*/
int XBINARY_OPERATION = 19;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Left Operand' containment reference.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__LEFT_OPERAND = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The feature id for the 'Right Operand' containment reference.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__RIGHT_OPERAND = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The feature id for the 'Reassign First Argument' attribute.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION__REASSIGN_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 2;
/**
* The number of structural features of the 'XBinary Operation' class.
*
*
* @generated
* @ordered
*/
int XBINARY_OPERATION_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 3;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XUnaryOperationImpl XUnary Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XUnaryOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXUnaryOperation()
* @generated
*/
int XUNARY_OPERATION = 20;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Operand' containment reference.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION__OPERAND = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XUnary Operation' class.
*
*
* @generated
* @ordered
*/
int XUNARY_OPERATION_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XPostfixOperationImpl XPostfix Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XPostfixOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXPostfixOperation()
* @generated
*/
int XPOSTFIX_OPERATION = 21;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Operand' containment reference.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION__OPERAND = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XPostfix Operation' class.
*
*
* @generated
* @ordered
*/
int XPOSTFIX_OPERATION_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XForLoopExpressionImpl XFor Loop Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XForLoopExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXForLoopExpression()
* @generated
*/
int XFOR_LOOP_EXPRESSION = 22;
/**
* The feature id for the 'For Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XFOR_LOOP_EXPRESSION__FOR_EXPRESSION = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Each Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XFOR_LOOP_EXPRESSION__EACH_EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Declared Param' containment reference.
*
*
* @generated
* @ordered
*/
int XFOR_LOOP_EXPRESSION__DECLARED_PARAM = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The number of structural features of the 'XFor Loop Expression' class.
*
*
* @generated
* @ordered
*/
int XFOR_LOOP_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XBasicForLoopExpressionImpl XBasic For Loop Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBasicForLoopExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBasicForLoopExpression()
* @generated
*/
int XBASIC_FOR_LOOP_EXPRESSION = 23;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XBASIC_FOR_LOOP_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Each Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XBASIC_FOR_LOOP_EXPRESSION__EACH_EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Init Expressions' containment reference list.
*
*
* @generated
* @ordered
*/
int XBASIC_FOR_LOOP_EXPRESSION__INIT_EXPRESSIONS = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The feature id for the 'Update Expressions' containment reference list.
*
*
* @generated
* @ordered
*/
int XBASIC_FOR_LOOP_EXPRESSION__UPDATE_EXPRESSIONS = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XBasic For Loop Expression' class.
*
*
* @generated
* @ordered
*/
int XBASIC_FOR_LOOP_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XAbstractWhileExpressionImpl XAbstract While Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAbstractWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAbstractWhileExpression()
* @generated
*/
int XABSTRACT_WHILE_EXPRESSION = 24;
/**
* The feature id for the 'Predicate' containment reference.
*
*
* @generated
* @ordered
*/
int XABSTRACT_WHILE_EXPRESSION__PREDICATE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Body' containment reference.
*
*
* @generated
* @ordered
*/
int XABSTRACT_WHILE_EXPRESSION__BODY = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'XAbstract While Expression' class.
*
*
* @generated
* @ordered
*/
int XABSTRACT_WHILE_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XDoWhileExpressionImpl XDo While Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XDoWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXDoWhileExpression()
* @generated
*/
int XDO_WHILE_EXPRESSION = 25;
/**
* The feature id for the 'Predicate' containment reference.
*
*
* @generated
* @ordered
*/
int XDO_WHILE_EXPRESSION__PREDICATE = XABSTRACT_WHILE_EXPRESSION__PREDICATE;
/**
* The feature id for the 'Body' containment reference.
*
*
* @generated
* @ordered
*/
int XDO_WHILE_EXPRESSION__BODY = XABSTRACT_WHILE_EXPRESSION__BODY;
/**
* The number of structural features of the 'XDo While Expression' class.
*
*
* @generated
* @ordered
*/
int XDO_WHILE_EXPRESSION_FEATURE_COUNT = XABSTRACT_WHILE_EXPRESSION_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XWhileExpressionImpl XWhile Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXWhileExpression()
* @generated
*/
int XWHILE_EXPRESSION = 26;
/**
* The feature id for the 'Predicate' containment reference.
*
*
* @generated
* @ordered
*/
int XWHILE_EXPRESSION__PREDICATE = XABSTRACT_WHILE_EXPRESSION__PREDICATE;
/**
* The feature id for the 'Body' containment reference.
*
*
* @generated
* @ordered
*/
int XWHILE_EXPRESSION__BODY = XABSTRACT_WHILE_EXPRESSION__BODY;
/**
* The number of structural features of the 'XWhile Expression' class.
*
*
* @generated
* @ordered
*/
int XWHILE_EXPRESSION_FEATURE_COUNT = XABSTRACT_WHILE_EXPRESSION_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XTypeLiteralImpl XType Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XTypeLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXTypeLiteral()
* @generated
*/
int XTYPE_LITERAL = 27;
/**
* The feature id for the 'Type' reference.
*
*
* @generated
* @ordered
*/
int XTYPE_LITERAL__TYPE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Array Dimensions' attribute list.
*
*
* @generated
* @ordered
*/
int XTYPE_LITERAL__ARRAY_DIMENSIONS = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'XType Literal' class.
*
*
* @generated
* @ordered
*/
int XTYPE_LITERAL_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XInstanceOfExpressionImpl XInstance Of Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XInstanceOfExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXInstanceOfExpression()
* @generated
*/
int XINSTANCE_OF_EXPRESSION = 28;
/**
* The feature id for the 'Type' containment reference.
*
*
* @generated
* @ordered
*/
int XINSTANCE_OF_EXPRESSION__TYPE = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XINSTANCE_OF_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'XInstance Of Expression' class.
*
*
* @generated
* @ordered
*/
int XINSTANCE_OF_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XThrowExpressionImpl XThrow Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XThrowExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXThrowExpression()
* @generated
*/
int XTHROW_EXPRESSION = 29;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XTHROW_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XThrow Expression' class.
*
*
* @generated
* @ordered
*/
int XTHROW_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XTryCatchFinallyExpressionImpl XTry Catch Finally Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XTryCatchFinallyExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXTryCatchFinallyExpression()
* @generated
*/
int XTRY_CATCH_FINALLY_EXPRESSION = 30;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XTRY_CATCH_FINALLY_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Finally Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XTRY_CATCH_FINALLY_EXPRESSION__FINALLY_EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The feature id for the 'Catch Clauses' containment reference list.
*
*
* @generated
* @ordered
*/
int XTRY_CATCH_FINALLY_EXPRESSION__CATCH_CLAUSES = XEXPRESSION_FEATURE_COUNT + 2;
/**
* The number of structural features of the 'XTry Catch Finally Expression' class.
*
*
* @generated
* @ordered
*/
int XTRY_CATCH_FINALLY_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 3;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XCatchClauseImpl XCatch Clause}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCatchClauseImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCatchClause()
* @generated
*/
int XCATCH_CLAUSE = 31;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XCATCH_CLAUSE__EXPRESSION = 0;
/**
* The feature id for the 'Declared Param' containment reference.
*
*
* @generated
* @ordered
*/
int XCATCH_CLAUSE__DECLARED_PARAM = 1;
/**
* The number of structural features of the 'XCatch Clause' class.
*
*
* @generated
* @ordered
*/
int XCATCH_CLAUSE_FEATURE_COUNT = 2;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XAssignmentImpl XAssignment}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAssignmentImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAssignment()
* @generated
*/
int XASSIGNMENT = 32;
/**
* The feature id for the 'Feature' reference.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__FEATURE = XABSTRACT_FEATURE_CALL__FEATURE;
/**
* The feature id for the 'Type Arguments' containment reference list.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__TYPE_ARGUMENTS = XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS;
/**
* The feature id for the 'Implicit Receiver' containment reference.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__IMPLICIT_RECEIVER = XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER;
/**
* The feature id for the 'Invalid Feature Issue Code' attribute.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__INVALID_FEATURE_ISSUE_CODE = XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE;
/**
* The feature id for the 'Valid Feature' attribute.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__VALID_FEATURE = XABSTRACT_FEATURE_CALL__VALID_FEATURE;
/**
* The feature id for the 'Implicit First Argument' containment reference.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__IMPLICIT_FIRST_ARGUMENT = XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT;
/**
* The feature id for the 'Assignable' containment reference.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__ASSIGNABLE = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 0;
/**
* The feature id for the 'Value' containment reference.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__VALUE = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 1;
/**
* The feature id for the 'Explicit Static' attribute.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__EXPLICIT_STATIC = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 2;
/**
* The feature id for the 'Static With Declaring Type' attribute.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT__STATIC_WITH_DECLARING_TYPE = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'XAssignment' class.
*
*
* @generated
* @ordered
*/
int XASSIGNMENT_FEATURE_COUNT = XABSTRACT_FEATURE_CALL_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XReturnExpressionImpl XReturn Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XReturnExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXReturnExpression()
* @generated
*/
int XRETURN_EXPRESSION = 33;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XRETURN_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'XReturn Expression' class.
*
*
* @generated
* @ordered
*/
int XRETURN_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link org.eclipse.xtext.xbase.impl.XSynchronizedExpressionImpl XSynchronized Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSynchronizedExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSynchronizedExpression()
* @generated
*/
int XSYNCHRONIZED_EXPRESSION = 34;
/**
* The feature id for the 'Param' containment reference.
*
*
* @generated
* @ordered
*/
int XSYNCHRONIZED_EXPRESSION__PARAM = XEXPRESSION_FEATURE_COUNT + 0;
/**
* The feature id for the 'Expression' containment reference.
*
*
* @generated
* @ordered
*/
int XSYNCHRONIZED_EXPRESSION__EXPRESSION = XEXPRESSION_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'XSynchronized Expression' class.
*
*
* @generated
* @ordered
*/
int XSYNCHRONIZED_EXPRESSION_FEATURE_COUNT = XEXPRESSION_FEATURE_COUNT + 2;
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XExpression XExpression}'.
*
*
* @return the meta object for class 'XExpression'.
* @see org.eclipse.xtext.xbase.XExpression
* @generated
*/
EClass getXExpression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XIfExpression XIf Expression}'.
*
*
* @return the meta object for class 'XIf Expression'.
* @see org.eclipse.xtext.xbase.XIfExpression
* @generated
*/
EClass getXIfExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XIfExpression#getIf If}'.
*
*
* @return the meta object for the containment reference 'If'.
* @see org.eclipse.xtext.xbase.XIfExpression#getIf()
* @see #getXIfExpression()
* @generated
*/
EReference getXIfExpression_If();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XIfExpression#getThen Then}'.
*
*
* @return the meta object for the containment reference 'Then'.
* @see org.eclipse.xtext.xbase.XIfExpression#getThen()
* @see #getXIfExpression()
* @generated
*/
EReference getXIfExpression_Then();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XIfExpression#getElse Else}'.
*
*
* @return the meta object for the containment reference 'Else'.
* @see org.eclipse.xtext.xbase.XIfExpression#getElse()
* @see #getXIfExpression()
* @generated
*/
EReference getXIfExpression_Else();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XSwitchExpression XSwitch Expression}'.
*
*
* @return the meta object for class 'XSwitch Expression'.
* @see org.eclipse.xtext.xbase.XSwitchExpression
* @generated
*/
EClass getXSwitchExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XSwitchExpression#getSwitch Switch}'.
*
*
* @return the meta object for the containment reference 'Switch'.
* @see org.eclipse.xtext.xbase.XSwitchExpression#getSwitch()
* @see #getXSwitchExpression()
* @generated
*/
EReference getXSwitchExpression_Switch();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XSwitchExpression#getCases Cases}'.
*
*
* @return the meta object for the containment reference list 'Cases'.
* @see org.eclipse.xtext.xbase.XSwitchExpression#getCases()
* @see #getXSwitchExpression()
* @generated
*/
EReference getXSwitchExpression_Cases();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XSwitchExpression#getDefault Default}'.
*
*
* @return the meta object for the containment reference 'Default'.
* @see org.eclipse.xtext.xbase.XSwitchExpression#getDefault()
* @see #getXSwitchExpression()
* @generated
*/
EReference getXSwitchExpression_Default();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XSwitchExpression#getDeclaredParam Declared Param}'.
*
*
* @return the meta object for the containment reference 'Declared Param'.
* @see org.eclipse.xtext.xbase.XSwitchExpression#getDeclaredParam()
* @see #getXSwitchExpression()
* @generated
*/
EReference getXSwitchExpression_DeclaredParam();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XCasePart XCase Part}'.
*
*
* @return the meta object for class 'XCase Part'.
* @see org.eclipse.xtext.xbase.XCasePart
* @generated
*/
EClass getXCasePart();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCasePart#getCase Case}'.
*
*
* @return the meta object for the containment reference 'Case'.
* @see org.eclipse.xtext.xbase.XCasePart#getCase()
* @see #getXCasePart()
* @generated
*/
EReference getXCasePart_Case();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCasePart#getThen Then}'.
*
*
* @return the meta object for the containment reference 'Then'.
* @see org.eclipse.xtext.xbase.XCasePart#getThen()
* @see #getXCasePart()
* @generated
*/
EReference getXCasePart_Then();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCasePart#getTypeGuard Type Guard}'.
*
*
* @return the meta object for the containment reference 'Type Guard'.
* @see org.eclipse.xtext.xbase.XCasePart#getTypeGuard()
* @see #getXCasePart()
* @generated
*/
EReference getXCasePart_TypeGuard();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XCasePart#isFallThrough Fall Through}'.
*
*
* @return the meta object for the attribute 'Fall Through'.
* @see org.eclipse.xtext.xbase.XCasePart#isFallThrough()
* @see #getXCasePart()
* @generated
*/
EAttribute getXCasePart_FallThrough();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XBlockExpression XBlock Expression}'.
*
*
* @return the meta object for class 'XBlock Expression'.
* @see org.eclipse.xtext.xbase.XBlockExpression
* @generated
*/
EClass getXBlockExpression();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XBlockExpression#getExpressions Expressions}'.
*
*
* @return the meta object for the containment reference list 'Expressions'.
* @see org.eclipse.xtext.xbase.XBlockExpression#getExpressions()
* @see #getXBlockExpression()
* @generated
*/
EReference getXBlockExpression_Expressions();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XVariableDeclaration XVariable Declaration}'.
*
*
* @return the meta object for class 'XVariable Declaration'.
* @see org.eclipse.xtext.xbase.XVariableDeclaration
* @generated
*/
EClass getXVariableDeclaration();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XVariableDeclaration#getType Type}'.
*
*
* @return the meta object for the containment reference 'Type'.
* @see org.eclipse.xtext.xbase.XVariableDeclaration#getType()
* @see #getXVariableDeclaration()
* @generated
*/
EReference getXVariableDeclaration_Type();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XVariableDeclaration#getName Name}'.
*
*
* @return the meta object for the attribute 'Name'.
* @see org.eclipse.xtext.xbase.XVariableDeclaration#getName()
* @see #getXVariableDeclaration()
* @generated
*/
EAttribute getXVariableDeclaration_Name();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XVariableDeclaration#getRight Right}'.
*
*
* @return the meta object for the containment reference 'Right'.
* @see org.eclipse.xtext.xbase.XVariableDeclaration#getRight()
* @see #getXVariableDeclaration()
* @generated
*/
EReference getXVariableDeclaration_Right();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XVariableDeclaration#isWriteable Writeable}'.
*
*
* @return the meta object for the attribute 'Writeable'.
* @see org.eclipse.xtext.xbase.XVariableDeclaration#isWriteable()
* @see #getXVariableDeclaration()
* @generated
*/
EAttribute getXVariableDeclaration_Writeable();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall XAbstract Feature Call}'.
*
*
* @return the meta object for class 'XAbstract Feature Call'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall
* @generated
*/
EClass getXAbstractFeatureCall();
/**
* Returns the meta object for the reference '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#getFeature Feature}'.
*
*
* @return the meta object for the reference 'Feature'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#getFeature()
* @see #getXAbstractFeatureCall()
* @generated
*/
EReference getXAbstractFeatureCall_Feature();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#getTypeArguments Type Arguments}'.
*
*
* @return the meta object for the containment reference list 'Type Arguments'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#getTypeArguments()
* @see #getXAbstractFeatureCall()
* @generated
*/
EReference getXAbstractFeatureCall_TypeArguments();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#getImplicitReceiver Implicit Receiver}'.
*
*
* @return the meta object for the containment reference 'Implicit Receiver'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#getImplicitReceiver()
* @see #getXAbstractFeatureCall()
* @generated
*/
EReference getXAbstractFeatureCall_ImplicitReceiver();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#getInvalidFeatureIssueCode Invalid Feature Issue Code}'.
*
*
* @return the meta object for the attribute 'Invalid Feature Issue Code'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#getInvalidFeatureIssueCode()
* @see #getXAbstractFeatureCall()
* @generated
*/
EAttribute getXAbstractFeatureCall_InvalidFeatureIssueCode();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#isValidFeature Valid Feature}'.
*
*
* @return the meta object for the attribute 'Valid Feature'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#isValidFeature()
* @see #getXAbstractFeatureCall()
* @generated
*/
EAttribute getXAbstractFeatureCall_ValidFeature();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAbstractFeatureCall#getImplicitFirstArgument Implicit First Argument}'.
*
*
* @return the meta object for the containment reference 'Implicit First Argument'.
* @see org.eclipse.xtext.xbase.XAbstractFeatureCall#getImplicitFirstArgument()
* @see #getXAbstractFeatureCall()
* @generated
*/
EReference getXAbstractFeatureCall_ImplicitFirstArgument();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XMemberFeatureCall XMember Feature Call}'.
*
*
* @return the meta object for class 'XMember Feature Call'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall
* @generated
*/
EClass getXMemberFeatureCall();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#getMemberCallTarget Member Call Target}'.
*
*
* @return the meta object for the containment reference 'Member Call Target'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#getMemberCallTarget()
* @see #getXMemberFeatureCall()
* @generated
*/
EReference getXMemberFeatureCall_MemberCallTarget();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#getMemberCallArguments Member Call Arguments}'.
*
*
* @return the meta object for the containment reference list 'Member Call Arguments'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#getMemberCallArguments()
* @see #getXMemberFeatureCall()
* @generated
*/
EReference getXMemberFeatureCall_MemberCallArguments();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isExplicitOperationCall Explicit Operation Call}'.
*
*
* @return the meta object for the attribute 'Explicit Operation Call'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isExplicitOperationCall()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_ExplicitOperationCall();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isExplicitStatic Explicit Static}'.
*
*
* @return the meta object for the attribute 'Explicit Static'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isExplicitStatic()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_ExplicitStatic();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isNullSafe Null Safe}'.
*
*
* @return the meta object for the attribute 'Null Safe'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isNullSafe()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_NullSafe();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isTypeLiteral Type Literal}'.
*
*
* @return the meta object for the attribute 'Type Literal'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isTypeLiteral()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_TypeLiteral();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isStaticWithDeclaringType Static With Declaring Type}'.
*
*
* @return the meta object for the attribute 'Static With Declaring Type'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isStaticWithDeclaringType()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_StaticWithDeclaringType();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XMemberFeatureCall#isPackageFragment Package Fragment}'.
*
*
* @return the meta object for the attribute 'Package Fragment'.
* @see org.eclipse.xtext.xbase.XMemberFeatureCall#isPackageFragment()
* @see #getXMemberFeatureCall()
* @generated
*/
EAttribute getXMemberFeatureCall_PackageFragment();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XFeatureCall XFeature Call}'.
*
*
* @return the meta object for class 'XFeature Call'.
* @see org.eclipse.xtext.xbase.XFeatureCall
* @generated
*/
EClass getXFeatureCall();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XFeatureCall#getFeatureCallArguments Feature Call Arguments}'.
*
*
* @return the meta object for the containment reference list 'Feature Call Arguments'.
* @see org.eclipse.xtext.xbase.XFeatureCall#getFeatureCallArguments()
* @see #getXFeatureCall()
* @generated
*/
EReference getXFeatureCall_FeatureCallArguments();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XFeatureCall#isExplicitOperationCall Explicit Operation Call}'.
*
*
* @return the meta object for the attribute 'Explicit Operation Call'.
* @see org.eclipse.xtext.xbase.XFeatureCall#isExplicitOperationCall()
* @see #getXFeatureCall()
* @generated
*/
EAttribute getXFeatureCall_ExplicitOperationCall();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XFeatureCall#isTypeLiteral Type Literal}'.
*
*
* @return the meta object for the attribute 'Type Literal'.
* @see org.eclipse.xtext.xbase.XFeatureCall#isTypeLiteral()
* @see #getXFeatureCall()
* @generated
*/
EAttribute getXFeatureCall_TypeLiteral();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XFeatureCall#isPackageFragment Package Fragment}'.
*
*
* @return the meta object for the attribute 'Package Fragment'.
* @see org.eclipse.xtext.xbase.XFeatureCall#isPackageFragment()
* @see #getXFeatureCall()
* @generated
*/
EAttribute getXFeatureCall_PackageFragment();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XConstructorCall XConstructor Call}'.
*
*
* @return the meta object for class 'XConstructor Call'.
* @see org.eclipse.xtext.xbase.XConstructorCall
* @generated
*/
EClass getXConstructorCall();
/**
* Returns the meta object for the reference '{@link org.eclipse.xtext.xbase.XConstructorCall#getConstructor Constructor}'.
*
*
* @return the meta object for the reference 'Constructor'.
* @see org.eclipse.xtext.xbase.XConstructorCall#getConstructor()
* @see #getXConstructorCall()
* @generated
*/
EReference getXConstructorCall_Constructor();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XConstructorCall#getArguments Arguments}'.
*
*
* @return the meta object for the containment reference list 'Arguments'.
* @see org.eclipse.xtext.xbase.XConstructorCall#getArguments()
* @see #getXConstructorCall()
* @generated
*/
EReference getXConstructorCall_Arguments();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XConstructorCall#getTypeArguments Type Arguments}'.
*
*
* @return the meta object for the containment reference list 'Type Arguments'.
* @see org.eclipse.xtext.xbase.XConstructorCall#getTypeArguments()
* @see #getXConstructorCall()
* @generated
*/
EReference getXConstructorCall_TypeArguments();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XConstructorCall#getInvalidFeatureIssueCode Invalid Feature Issue Code}'.
*
*
* @return the meta object for the attribute 'Invalid Feature Issue Code'.
* @see org.eclipse.xtext.xbase.XConstructorCall#getInvalidFeatureIssueCode()
* @see #getXConstructorCall()
* @generated
*/
EAttribute getXConstructorCall_InvalidFeatureIssueCode();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XConstructorCall#isValidFeature Valid Feature}'.
*
*
* @return the meta object for the attribute 'Valid Feature'.
* @see org.eclipse.xtext.xbase.XConstructorCall#isValidFeature()
* @see #getXConstructorCall()
* @generated
*/
EAttribute getXConstructorCall_ValidFeature();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XConstructorCall#isExplicitConstructorCall Explicit Constructor Call}'.
*
*
* @return the meta object for the attribute 'Explicit Constructor Call'.
* @see org.eclipse.xtext.xbase.XConstructorCall#isExplicitConstructorCall()
* @see #getXConstructorCall()
* @generated
*/
EAttribute getXConstructorCall_ExplicitConstructorCall();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XConstructorCall#isAnonymousClassConstructorCall Anonymous Class Constructor Call}'.
*
*
* @return the meta object for the attribute 'Anonymous Class Constructor Call'.
* @see org.eclipse.xtext.xbase.XConstructorCall#isAnonymousClassConstructorCall()
* @see #getXConstructorCall()
* @generated
*/
EAttribute getXConstructorCall_AnonymousClassConstructorCall();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XBooleanLiteral XBoolean Literal}'.
*
*
* @return the meta object for class 'XBoolean Literal'.
* @see org.eclipse.xtext.xbase.XBooleanLiteral
* @generated
*/
EClass getXBooleanLiteral();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XBooleanLiteral#isIsTrue Is True}'.
*
*
* @return the meta object for the attribute 'Is True'.
* @see org.eclipse.xtext.xbase.XBooleanLiteral#isIsTrue()
* @see #getXBooleanLiteral()
* @generated
*/
EAttribute getXBooleanLiteral_IsTrue();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XNullLiteral XNull Literal}'.
*
*
* @return the meta object for class 'XNull Literal'.
* @see org.eclipse.xtext.xbase.XNullLiteral
* @generated
*/
EClass getXNullLiteral();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XNumberLiteral XNumber Literal}'.
*
*
* @return the meta object for class 'XNumber Literal'.
* @see org.eclipse.xtext.xbase.XNumberLiteral
* @generated
*/
EClass getXNumberLiteral();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XNumberLiteral#getValue Value}'.
*
*
* @return the meta object for the attribute 'Value'.
* @see org.eclipse.xtext.xbase.XNumberLiteral#getValue()
* @see #getXNumberLiteral()
* @generated
*/
EAttribute getXNumberLiteral_Value();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XStringLiteral XString Literal}'.
*
*
* @return the meta object for class 'XString Literal'.
* @see org.eclipse.xtext.xbase.XStringLiteral
* @generated
*/
EClass getXStringLiteral();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XStringLiteral#getValue Value}'.
*
*
* @return the meta object for the attribute 'Value'.
* @see org.eclipse.xtext.xbase.XStringLiteral#getValue()
* @see #getXStringLiteral()
* @generated
*/
EAttribute getXStringLiteral_Value();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XCollectionLiteral XCollection Literal}'.
*
*
* @return the meta object for class 'XCollection Literal'.
* @see org.eclipse.xtext.xbase.XCollectionLiteral
* @generated
*/
EClass getXCollectionLiteral();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XCollectionLiteral#getElements Elements}'.
*
*
* @return the meta object for the containment reference list 'Elements'.
* @see org.eclipse.xtext.xbase.XCollectionLiteral#getElements()
* @see #getXCollectionLiteral()
* @generated
*/
EReference getXCollectionLiteral_Elements();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XListLiteral XList Literal}'.
*
*
* @return the meta object for class 'XList Literal'.
* @see org.eclipse.xtext.xbase.XListLiteral
* @generated
*/
EClass getXListLiteral();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XSetLiteral XSet Literal}'.
*
*
* @return the meta object for class 'XSet Literal'.
* @see org.eclipse.xtext.xbase.XSetLiteral
* @generated
*/
EClass getXSetLiteral();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XClosure XClosure}'.
*
*
* @return the meta object for class 'XClosure'.
* @see org.eclipse.xtext.xbase.XClosure
* @generated
*/
EClass getXClosure();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XClosure#getDeclaredFormalParameters Declared Formal Parameters}'.
*
*
* @return the meta object for the containment reference list 'Declared Formal Parameters'.
* @see org.eclipse.xtext.xbase.XClosure#getDeclaredFormalParameters()
* @see #getXClosure()
* @generated
*/
EReference getXClosure_DeclaredFormalParameters();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XClosure#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XClosure#getExpression()
* @see #getXClosure()
* @generated
*/
EReference getXClosure_Expression();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XClosure#isExplicitSyntax Explicit Syntax}'.
*
*
* @return the meta object for the attribute 'Explicit Syntax'.
* @see org.eclipse.xtext.xbase.XClosure#isExplicitSyntax()
* @see #getXClosure()
* @generated
*/
EAttribute getXClosure_ExplicitSyntax();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XClosure#getImplicitFormalParameters Implicit Formal Parameters}'.
*
*
* @return the meta object for the containment reference list 'Implicit Formal Parameters'.
* @see org.eclipse.xtext.xbase.XClosure#getImplicitFormalParameters()
* @see #getXClosure()
* @generated
*/
EReference getXClosure_ImplicitFormalParameters();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XCastedExpression XCasted Expression}'.
*
*
* @return the meta object for class 'XCasted Expression'.
* @see org.eclipse.xtext.xbase.XCastedExpression
* @generated
*/
EClass getXCastedExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCastedExpression#getType Type}'.
*
*
* @return the meta object for the containment reference 'Type'.
* @see org.eclipse.xtext.xbase.XCastedExpression#getType()
* @see #getXCastedExpression()
* @generated
*/
EReference getXCastedExpression_Type();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCastedExpression#getTarget Target}'.
*
*
* @return the meta object for the containment reference 'Target'.
* @see org.eclipse.xtext.xbase.XCastedExpression#getTarget()
* @see #getXCastedExpression()
* @generated
*/
EReference getXCastedExpression_Target();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XBinaryOperation XBinary Operation}'.
*
*
* @return the meta object for class 'XBinary Operation'.
* @see org.eclipse.xtext.xbase.XBinaryOperation
* @generated
*/
EClass getXBinaryOperation();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XBinaryOperation#getLeftOperand Left Operand}'.
*
*
* @return the meta object for the containment reference 'Left Operand'.
* @see org.eclipse.xtext.xbase.XBinaryOperation#getLeftOperand()
* @see #getXBinaryOperation()
* @generated
*/
EReference getXBinaryOperation_LeftOperand();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XBinaryOperation#getRightOperand Right Operand}'.
*
*
* @return the meta object for the containment reference 'Right Operand'.
* @see org.eclipse.xtext.xbase.XBinaryOperation#getRightOperand()
* @see #getXBinaryOperation()
* @generated
*/
EReference getXBinaryOperation_RightOperand();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XBinaryOperation#isReassignFirstArgument Reassign First Argument}'.
*
*
* @return the meta object for the attribute 'Reassign First Argument'.
* @see org.eclipse.xtext.xbase.XBinaryOperation#isReassignFirstArgument()
* @see #getXBinaryOperation()
* @generated
*/
EAttribute getXBinaryOperation_ReassignFirstArgument();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XUnaryOperation XUnary Operation}'.
*
*
* @return the meta object for class 'XUnary Operation'.
* @see org.eclipse.xtext.xbase.XUnaryOperation
* @generated
*/
EClass getXUnaryOperation();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XUnaryOperation#getOperand Operand}'.
*
*
* @return the meta object for the containment reference 'Operand'.
* @see org.eclipse.xtext.xbase.XUnaryOperation#getOperand()
* @see #getXUnaryOperation()
* @generated
*/
EReference getXUnaryOperation_Operand();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XPostfixOperation XPostfix Operation}'.
*
*
* @return the meta object for class 'XPostfix Operation'.
* @see org.eclipse.xtext.xbase.XPostfixOperation
* @generated
*/
EClass getXPostfixOperation();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XPostfixOperation#getOperand Operand}'.
*
*
* @return the meta object for the containment reference 'Operand'.
* @see org.eclipse.xtext.xbase.XPostfixOperation#getOperand()
* @see #getXPostfixOperation()
* @generated
*/
EReference getXPostfixOperation_Operand();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XForLoopExpression XFor Loop Expression}'.
*
*
* @return the meta object for class 'XFor Loop Expression'.
* @see org.eclipse.xtext.xbase.XForLoopExpression
* @generated
*/
EClass getXForLoopExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XForLoopExpression#getForExpression For Expression}'.
*
*
* @return the meta object for the containment reference 'For Expression'.
* @see org.eclipse.xtext.xbase.XForLoopExpression#getForExpression()
* @see #getXForLoopExpression()
* @generated
*/
EReference getXForLoopExpression_ForExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XForLoopExpression#getEachExpression Each Expression}'.
*
*
* @return the meta object for the containment reference 'Each Expression'.
* @see org.eclipse.xtext.xbase.XForLoopExpression#getEachExpression()
* @see #getXForLoopExpression()
* @generated
*/
EReference getXForLoopExpression_EachExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XForLoopExpression#getDeclaredParam Declared Param}'.
*
*
* @return the meta object for the containment reference 'Declared Param'.
* @see org.eclipse.xtext.xbase.XForLoopExpression#getDeclaredParam()
* @see #getXForLoopExpression()
* @generated
*/
EReference getXForLoopExpression_DeclaredParam();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XBasicForLoopExpression XBasic For Loop Expression}'.
*
*
* @return the meta object for class 'XBasic For Loop Expression'.
* @see org.eclipse.xtext.xbase.XBasicForLoopExpression
* @generated
*/
EClass getXBasicForLoopExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XBasicForLoopExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XBasicForLoopExpression#getExpression()
* @see #getXBasicForLoopExpression()
* @generated
*/
EReference getXBasicForLoopExpression_Expression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XBasicForLoopExpression#getEachExpression Each Expression}'.
*
*
* @return the meta object for the containment reference 'Each Expression'.
* @see org.eclipse.xtext.xbase.XBasicForLoopExpression#getEachExpression()
* @see #getXBasicForLoopExpression()
* @generated
*/
EReference getXBasicForLoopExpression_EachExpression();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XBasicForLoopExpression#getInitExpressions Init Expressions}'.
*
*
* @return the meta object for the containment reference list 'Init Expressions'.
* @see org.eclipse.xtext.xbase.XBasicForLoopExpression#getInitExpressions()
* @see #getXBasicForLoopExpression()
* @generated
*/
EReference getXBasicForLoopExpression_InitExpressions();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XBasicForLoopExpression#getUpdateExpressions Update Expressions}'.
*
*
* @return the meta object for the containment reference list 'Update Expressions'.
* @see org.eclipse.xtext.xbase.XBasicForLoopExpression#getUpdateExpressions()
* @see #getXBasicForLoopExpression()
* @generated
*/
EReference getXBasicForLoopExpression_UpdateExpressions();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XAbstractWhileExpression XAbstract While Expression}'.
*
*
* @return the meta object for class 'XAbstract While Expression'.
* @see org.eclipse.xtext.xbase.XAbstractWhileExpression
* @generated
*/
EClass getXAbstractWhileExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAbstractWhileExpression#getPredicate Predicate}'.
*
*
* @return the meta object for the containment reference 'Predicate'.
* @see org.eclipse.xtext.xbase.XAbstractWhileExpression#getPredicate()
* @see #getXAbstractWhileExpression()
* @generated
*/
EReference getXAbstractWhileExpression_Predicate();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAbstractWhileExpression#getBody Body}'.
*
*
* @return the meta object for the containment reference 'Body'.
* @see org.eclipse.xtext.xbase.XAbstractWhileExpression#getBody()
* @see #getXAbstractWhileExpression()
* @generated
*/
EReference getXAbstractWhileExpression_Body();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XDoWhileExpression XDo While Expression}'.
*
*
* @return the meta object for class 'XDo While Expression'.
* @see org.eclipse.xtext.xbase.XDoWhileExpression
* @generated
*/
EClass getXDoWhileExpression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XWhileExpression XWhile Expression}'.
*
*
* @return the meta object for class 'XWhile Expression'.
* @see org.eclipse.xtext.xbase.XWhileExpression
* @generated
*/
EClass getXWhileExpression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XTypeLiteral XType Literal}'.
*
*
* @return the meta object for class 'XType Literal'.
* @see org.eclipse.xtext.xbase.XTypeLiteral
* @generated
*/
EClass getXTypeLiteral();
/**
* Returns the meta object for the reference '{@link org.eclipse.xtext.xbase.XTypeLiteral#getType Type}'.
*
*
* @return the meta object for the reference 'Type'.
* @see org.eclipse.xtext.xbase.XTypeLiteral#getType()
* @see #getXTypeLiteral()
* @generated
*/
EReference getXTypeLiteral_Type();
/**
* Returns the meta object for the attribute list '{@link org.eclipse.xtext.xbase.XTypeLiteral#getArrayDimensions Array Dimensions}'.
*
*
* @return the meta object for the attribute list 'Array Dimensions'.
* @see org.eclipse.xtext.xbase.XTypeLiteral#getArrayDimensions()
* @see #getXTypeLiteral()
* @generated
*/
EAttribute getXTypeLiteral_ArrayDimensions();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XInstanceOfExpression XInstance Of Expression}'.
*
*
* @return the meta object for class 'XInstance Of Expression'.
* @see org.eclipse.xtext.xbase.XInstanceOfExpression
* @generated
*/
EClass getXInstanceOfExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XInstanceOfExpression#getType Type}'.
*
*
* @return the meta object for the containment reference 'Type'.
* @see org.eclipse.xtext.xbase.XInstanceOfExpression#getType()
* @see #getXInstanceOfExpression()
* @generated
*/
EReference getXInstanceOfExpression_Type();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XInstanceOfExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XInstanceOfExpression#getExpression()
* @see #getXInstanceOfExpression()
* @generated
*/
EReference getXInstanceOfExpression_Expression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XThrowExpression XThrow Expression}'.
*
*
* @return the meta object for class 'XThrow Expression'.
* @see org.eclipse.xtext.xbase.XThrowExpression
* @generated
*/
EClass getXThrowExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XThrowExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XThrowExpression#getExpression()
* @see #getXThrowExpression()
* @generated
*/
EReference getXThrowExpression_Expression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XTryCatchFinallyExpression XTry Catch Finally Expression}'.
*
*
* @return the meta object for class 'XTry Catch Finally Expression'.
* @see org.eclipse.xtext.xbase.XTryCatchFinallyExpression
* @generated
*/
EClass getXTryCatchFinallyExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getExpression()
* @see #getXTryCatchFinallyExpression()
* @generated
*/
EReference getXTryCatchFinallyExpression_Expression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getFinallyExpression Finally Expression}'.
*
*
* @return the meta object for the containment reference 'Finally Expression'.
* @see org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getFinallyExpression()
* @see #getXTryCatchFinallyExpression()
* @generated
*/
EReference getXTryCatchFinallyExpression_FinallyExpression();
/**
* Returns the meta object for the containment reference list '{@link org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getCatchClauses Catch Clauses}'.
*
*
* @return the meta object for the containment reference list 'Catch Clauses'.
* @see org.eclipse.xtext.xbase.XTryCatchFinallyExpression#getCatchClauses()
* @see #getXTryCatchFinallyExpression()
* @generated
*/
EReference getXTryCatchFinallyExpression_CatchClauses();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XCatchClause XCatch Clause}'.
*
*
* @return the meta object for class 'XCatch Clause'.
* @see org.eclipse.xtext.xbase.XCatchClause
* @generated
*/
EClass getXCatchClause();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCatchClause#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XCatchClause#getExpression()
* @see #getXCatchClause()
* @generated
*/
EReference getXCatchClause_Expression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XCatchClause#getDeclaredParam Declared Param}'.
*
*
* @return the meta object for the containment reference 'Declared Param'.
* @see org.eclipse.xtext.xbase.XCatchClause#getDeclaredParam()
* @see #getXCatchClause()
* @generated
*/
EReference getXCatchClause_DeclaredParam();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XAssignment XAssignment}'.
*
*
* @return the meta object for class 'XAssignment'.
* @see org.eclipse.xtext.xbase.XAssignment
* @generated
*/
EClass getXAssignment();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAssignment#getAssignable Assignable}'.
*
*
* @return the meta object for the containment reference 'Assignable'.
* @see org.eclipse.xtext.xbase.XAssignment#getAssignable()
* @see #getXAssignment()
* @generated
*/
EReference getXAssignment_Assignable();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XAssignment#getValue Value}'.
*
*
* @return the meta object for the containment reference 'Value'.
* @see org.eclipse.xtext.xbase.XAssignment#getValue()
* @see #getXAssignment()
* @generated
*/
EReference getXAssignment_Value();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XAssignment#isExplicitStatic Explicit Static}'.
*
*
* @return the meta object for the attribute 'Explicit Static'.
* @see org.eclipse.xtext.xbase.XAssignment#isExplicitStatic()
* @see #getXAssignment()
* @generated
*/
EAttribute getXAssignment_ExplicitStatic();
/**
* Returns the meta object for the attribute '{@link org.eclipse.xtext.xbase.XAssignment#isStaticWithDeclaringType Static With Declaring Type}'.
*
*
* @return the meta object for the attribute 'Static With Declaring Type'.
* @see org.eclipse.xtext.xbase.XAssignment#isStaticWithDeclaringType()
* @see #getXAssignment()
* @generated
*/
EAttribute getXAssignment_StaticWithDeclaringType();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XReturnExpression XReturn Expression}'.
*
*
* @return the meta object for class 'XReturn Expression'.
* @see org.eclipse.xtext.xbase.XReturnExpression
* @generated
*/
EClass getXReturnExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XReturnExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XReturnExpression#getExpression()
* @see #getXReturnExpression()
* @generated
*/
EReference getXReturnExpression_Expression();
/**
* Returns the meta object for class '{@link org.eclipse.xtext.xbase.XSynchronizedExpression XSynchronized Expression}'.
*
*
* @return the meta object for class 'XSynchronized Expression'.
* @see org.eclipse.xtext.xbase.XSynchronizedExpression
* @generated
*/
EClass getXSynchronizedExpression();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XSynchronizedExpression#getParam Param}'.
*
*
* @return the meta object for the containment reference 'Param'.
* @see org.eclipse.xtext.xbase.XSynchronizedExpression#getParam()
* @see #getXSynchronizedExpression()
* @generated
*/
EReference getXSynchronizedExpression_Param();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.xtext.xbase.XSynchronizedExpression#getExpression Expression}'.
*
*
* @return the meta object for the containment reference 'Expression'.
* @see org.eclipse.xtext.xbase.XSynchronizedExpression#getExpression()
* @see #getXSynchronizedExpression()
* @generated
*/
EReference getXSynchronizedExpression_Expression();
/**
* Returns the factory that creates the instances of the model.
*
*
* @return the factory that creates the instances of the model.
* @generated
*/
XbaseFactory getXbaseFactory();
/**
*
* Defines literals for the meta objects that represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @generated
*/
interface Literals
{
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XExpressionImpl XExpression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXExpression()
* @generated
*/
EClass XEXPRESSION = eINSTANCE.getXExpression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XIfExpressionImpl XIf Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XIfExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXIfExpression()
* @generated
*/
EClass XIF_EXPRESSION = eINSTANCE.getXIfExpression();
/**
* The meta object literal for the 'If' containment reference feature.
*
*
* @generated
*/
EReference XIF_EXPRESSION__IF = eINSTANCE.getXIfExpression_If();
/**
* The meta object literal for the 'Then' containment reference feature.
*
*
* @generated
*/
EReference XIF_EXPRESSION__THEN = eINSTANCE.getXIfExpression_Then();
/**
* The meta object literal for the 'Else' containment reference feature.
*
*
* @generated
*/
EReference XIF_EXPRESSION__ELSE = eINSTANCE.getXIfExpression_Else();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XSwitchExpressionImpl XSwitch Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSwitchExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSwitchExpression()
* @generated
*/
EClass XSWITCH_EXPRESSION = eINSTANCE.getXSwitchExpression();
/**
* The meta object literal for the 'Switch' containment reference feature.
*
*
* @generated
*/
EReference XSWITCH_EXPRESSION__SWITCH = eINSTANCE.getXSwitchExpression_Switch();
/**
* The meta object literal for the 'Cases' containment reference list feature.
*
*
* @generated
*/
EReference XSWITCH_EXPRESSION__CASES = eINSTANCE.getXSwitchExpression_Cases();
/**
* The meta object literal for the 'Default' containment reference feature.
*
*
* @generated
*/
EReference XSWITCH_EXPRESSION__DEFAULT = eINSTANCE.getXSwitchExpression_Default();
/**
* The meta object literal for the 'Declared Param' containment reference feature.
*
*
* @generated
*/
EReference XSWITCH_EXPRESSION__DECLARED_PARAM = eINSTANCE.getXSwitchExpression_DeclaredParam();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XCasePartImpl XCase Part}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCasePartImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCasePart()
* @generated
*/
EClass XCASE_PART = eINSTANCE.getXCasePart();
/**
* The meta object literal for the 'Case' containment reference feature.
*
*
* @generated
*/
EReference XCASE_PART__CASE = eINSTANCE.getXCasePart_Case();
/**
* The meta object literal for the 'Then' containment reference feature.
*
*
* @generated
*/
EReference XCASE_PART__THEN = eINSTANCE.getXCasePart_Then();
/**
* The meta object literal for the 'Type Guard' containment reference feature.
*
*
* @generated
*/
EReference XCASE_PART__TYPE_GUARD = eINSTANCE.getXCasePart_TypeGuard();
/**
* The meta object literal for the 'Fall Through' attribute feature.
*
*
* @generated
*/
EAttribute XCASE_PART__FALL_THROUGH = eINSTANCE.getXCasePart_FallThrough();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XBlockExpressionImpl XBlock Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBlockExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBlockExpression()
* @generated
*/
EClass XBLOCK_EXPRESSION = eINSTANCE.getXBlockExpression();
/**
* The meta object literal for the 'Expressions' containment reference list feature.
*
*
* @generated
*/
EReference XBLOCK_EXPRESSION__EXPRESSIONS = eINSTANCE.getXBlockExpression_Expressions();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XVariableDeclarationImpl XVariable Declaration}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XVariableDeclarationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXVariableDeclaration()
* @generated
*/
EClass XVARIABLE_DECLARATION = eINSTANCE.getXVariableDeclaration();
/**
* The meta object literal for the 'Type' containment reference feature.
*
*
* @generated
*/
EReference XVARIABLE_DECLARATION__TYPE = eINSTANCE.getXVariableDeclaration_Type();
/**
* The meta object literal for the 'Name' attribute feature.
*
*
* @generated
*/
EAttribute XVARIABLE_DECLARATION__NAME = eINSTANCE.getXVariableDeclaration_Name();
/**
* The meta object literal for the 'Right' containment reference feature.
*
*
* @generated
*/
EReference XVARIABLE_DECLARATION__RIGHT = eINSTANCE.getXVariableDeclaration_Right();
/**
* The meta object literal for the 'Writeable' attribute feature.
*
*
* @generated
*/
EAttribute XVARIABLE_DECLARATION__WRITEABLE = eINSTANCE.getXVariableDeclaration_Writeable();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XAbstractFeatureCallImpl XAbstract Feature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAbstractFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAbstractFeatureCall()
* @generated
*/
EClass XABSTRACT_FEATURE_CALL = eINSTANCE.getXAbstractFeatureCall();
/**
* The meta object literal for the 'Feature' reference feature.
*
*
* @generated
*/
EReference XABSTRACT_FEATURE_CALL__FEATURE = eINSTANCE.getXAbstractFeatureCall_Feature();
/**
* The meta object literal for the 'Type Arguments' containment reference list feature.
*
*
* @generated
*/
EReference XABSTRACT_FEATURE_CALL__TYPE_ARGUMENTS = eINSTANCE.getXAbstractFeatureCall_TypeArguments();
/**
* The meta object literal for the 'Implicit Receiver' containment reference feature.
*
*
* @generated
*/
EReference XABSTRACT_FEATURE_CALL__IMPLICIT_RECEIVER = eINSTANCE.getXAbstractFeatureCall_ImplicitReceiver();
/**
* The meta object literal for the 'Invalid Feature Issue Code' attribute feature.
*
*
* @generated
*/
EAttribute XABSTRACT_FEATURE_CALL__INVALID_FEATURE_ISSUE_CODE = eINSTANCE.getXAbstractFeatureCall_InvalidFeatureIssueCode();
/**
* The meta object literal for the 'Valid Feature' attribute feature.
*
*
* @generated
*/
EAttribute XABSTRACT_FEATURE_CALL__VALID_FEATURE = eINSTANCE.getXAbstractFeatureCall_ValidFeature();
/**
* The meta object literal for the 'Implicit First Argument' containment reference feature.
*
*
* @generated
*/
EReference XABSTRACT_FEATURE_CALL__IMPLICIT_FIRST_ARGUMENT = eINSTANCE.getXAbstractFeatureCall_ImplicitFirstArgument();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XMemberFeatureCallImpl XMember Feature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XMemberFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXMemberFeatureCall()
* @generated
*/
EClass XMEMBER_FEATURE_CALL = eINSTANCE.getXMemberFeatureCall();
/**
* The meta object literal for the 'Member Call Target' containment reference feature.
*
*
* @generated
*/
EReference XMEMBER_FEATURE_CALL__MEMBER_CALL_TARGET = eINSTANCE.getXMemberFeatureCall_MemberCallTarget();
/**
* The meta object literal for the 'Member Call Arguments' containment reference list feature.
*
*
* @generated
*/
EReference XMEMBER_FEATURE_CALL__MEMBER_CALL_ARGUMENTS = eINSTANCE.getXMemberFeatureCall_MemberCallArguments();
/**
* The meta object literal for the 'Explicit Operation Call' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__EXPLICIT_OPERATION_CALL = eINSTANCE.getXMemberFeatureCall_ExplicitOperationCall();
/**
* The meta object literal for the 'Explicit Static' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__EXPLICIT_STATIC = eINSTANCE.getXMemberFeatureCall_ExplicitStatic();
/**
* The meta object literal for the 'Null Safe' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__NULL_SAFE = eINSTANCE.getXMemberFeatureCall_NullSafe();
/**
* The meta object literal for the 'Type Literal' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__TYPE_LITERAL = eINSTANCE.getXMemberFeatureCall_TypeLiteral();
/**
* The meta object literal for the 'Static With Declaring Type' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__STATIC_WITH_DECLARING_TYPE = eINSTANCE.getXMemberFeatureCall_StaticWithDeclaringType();
/**
* The meta object literal for the 'Package Fragment' attribute feature.
*
*
* @generated
*/
EAttribute XMEMBER_FEATURE_CALL__PACKAGE_FRAGMENT = eINSTANCE.getXMemberFeatureCall_PackageFragment();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XFeatureCallImpl XFeature Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XFeatureCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXFeatureCall()
* @generated
*/
EClass XFEATURE_CALL = eINSTANCE.getXFeatureCall();
/**
* The meta object literal for the 'Feature Call Arguments' containment reference list feature.
*
*
* @generated
*/
EReference XFEATURE_CALL__FEATURE_CALL_ARGUMENTS = eINSTANCE.getXFeatureCall_FeatureCallArguments();
/**
* The meta object literal for the 'Explicit Operation Call' attribute feature.
*
*
* @generated
*/
EAttribute XFEATURE_CALL__EXPLICIT_OPERATION_CALL = eINSTANCE.getXFeatureCall_ExplicitOperationCall();
/**
* The meta object literal for the 'Type Literal' attribute feature.
*
*
* @generated
*/
EAttribute XFEATURE_CALL__TYPE_LITERAL = eINSTANCE.getXFeatureCall_TypeLiteral();
/**
* The meta object literal for the 'Package Fragment' attribute feature.
*
*
* @generated
*/
EAttribute XFEATURE_CALL__PACKAGE_FRAGMENT = eINSTANCE.getXFeatureCall_PackageFragment();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XConstructorCallImpl XConstructor Call}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XConstructorCallImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXConstructorCall()
* @generated
*/
EClass XCONSTRUCTOR_CALL = eINSTANCE.getXConstructorCall();
/**
* The meta object literal for the 'Constructor' reference feature.
*
*
* @generated
*/
EReference XCONSTRUCTOR_CALL__CONSTRUCTOR = eINSTANCE.getXConstructorCall_Constructor();
/**
* The meta object literal for the 'Arguments' containment reference list feature.
*
*
* @generated
*/
EReference XCONSTRUCTOR_CALL__ARGUMENTS = eINSTANCE.getXConstructorCall_Arguments();
/**
* The meta object literal for the 'Type Arguments' containment reference list feature.
*
*
* @generated
*/
EReference XCONSTRUCTOR_CALL__TYPE_ARGUMENTS = eINSTANCE.getXConstructorCall_TypeArguments();
/**
* The meta object literal for the 'Invalid Feature Issue Code' attribute feature.
*
*
* @generated
*/
EAttribute XCONSTRUCTOR_CALL__INVALID_FEATURE_ISSUE_CODE = eINSTANCE.getXConstructorCall_InvalidFeatureIssueCode();
/**
* The meta object literal for the 'Valid Feature' attribute feature.
*
*
* @generated
*/
EAttribute XCONSTRUCTOR_CALL__VALID_FEATURE = eINSTANCE.getXConstructorCall_ValidFeature();
/**
* The meta object literal for the 'Explicit Constructor Call' attribute feature.
*
*
* @generated
*/
EAttribute XCONSTRUCTOR_CALL__EXPLICIT_CONSTRUCTOR_CALL = eINSTANCE.getXConstructorCall_ExplicitConstructorCall();
/**
* The meta object literal for the 'Anonymous Class Constructor Call' attribute feature.
*
*
* @generated
*/
EAttribute XCONSTRUCTOR_CALL__ANONYMOUS_CLASS_CONSTRUCTOR_CALL = eINSTANCE.getXConstructorCall_AnonymousClassConstructorCall();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XBooleanLiteralImpl XBoolean Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBooleanLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBooleanLiteral()
* @generated
*/
EClass XBOOLEAN_LITERAL = eINSTANCE.getXBooleanLiteral();
/**
* The meta object literal for the 'Is True' attribute feature.
*
*
* @generated
*/
EAttribute XBOOLEAN_LITERAL__IS_TRUE = eINSTANCE.getXBooleanLiteral_IsTrue();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XNullLiteralImpl XNull Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XNullLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXNullLiteral()
* @generated
*/
EClass XNULL_LITERAL = eINSTANCE.getXNullLiteral();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XNumberLiteralImpl XNumber Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XNumberLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXNumberLiteral()
* @generated
*/
EClass XNUMBER_LITERAL = eINSTANCE.getXNumberLiteral();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
* @generated
*/
EAttribute XNUMBER_LITERAL__VALUE = eINSTANCE.getXNumberLiteral_Value();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XStringLiteralImpl XString Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XStringLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXStringLiteral()
* @generated
*/
EClass XSTRING_LITERAL = eINSTANCE.getXStringLiteral();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
* @generated
*/
EAttribute XSTRING_LITERAL__VALUE = eINSTANCE.getXStringLiteral_Value();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XCollectionLiteralImpl XCollection Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCollectionLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCollectionLiteral()
* @generated
*/
EClass XCOLLECTION_LITERAL = eINSTANCE.getXCollectionLiteral();
/**
* The meta object literal for the 'Elements' containment reference list feature.
*
*
* @generated
*/
EReference XCOLLECTION_LITERAL__ELEMENTS = eINSTANCE.getXCollectionLiteral_Elements();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XListLiteralImpl XList Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XListLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXListLiteral()
* @generated
*/
EClass XLIST_LITERAL = eINSTANCE.getXListLiteral();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XSetLiteralImpl XSet Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSetLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSetLiteral()
* @generated
*/
EClass XSET_LITERAL = eINSTANCE.getXSetLiteral();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XClosureImpl XClosure}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XClosureImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXClosure()
* @generated
*/
EClass XCLOSURE = eINSTANCE.getXClosure();
/**
* The meta object literal for the 'Declared Formal Parameters' containment reference list feature.
*
*
* @generated
*/
EReference XCLOSURE__DECLARED_FORMAL_PARAMETERS = eINSTANCE.getXClosure_DeclaredFormalParameters();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XCLOSURE__EXPRESSION = eINSTANCE.getXClosure_Expression();
/**
* The meta object literal for the 'Explicit Syntax' attribute feature.
*
*
* @generated
*/
EAttribute XCLOSURE__EXPLICIT_SYNTAX = eINSTANCE.getXClosure_ExplicitSyntax();
/**
* The meta object literal for the 'Implicit Formal Parameters' containment reference list feature.
*
*
* @generated
*/
EReference XCLOSURE__IMPLICIT_FORMAL_PARAMETERS = eINSTANCE.getXClosure_ImplicitFormalParameters();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XCastedExpressionImpl XCasted Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCastedExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCastedExpression()
* @generated
*/
EClass XCASTED_EXPRESSION = eINSTANCE.getXCastedExpression();
/**
* The meta object literal for the 'Type' containment reference feature.
*
*
* @generated
*/
EReference XCASTED_EXPRESSION__TYPE = eINSTANCE.getXCastedExpression_Type();
/**
* The meta object literal for the 'Target' containment reference feature.
*
*
* @generated
*/
EReference XCASTED_EXPRESSION__TARGET = eINSTANCE.getXCastedExpression_Target();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XBinaryOperationImpl XBinary Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBinaryOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBinaryOperation()
* @generated
*/
EClass XBINARY_OPERATION = eINSTANCE.getXBinaryOperation();
/**
* The meta object literal for the 'Left Operand' containment reference feature.
*
*
* @generated
*/
EReference XBINARY_OPERATION__LEFT_OPERAND = eINSTANCE.getXBinaryOperation_LeftOperand();
/**
* The meta object literal for the 'Right Operand' containment reference feature.
*
*
* @generated
*/
EReference XBINARY_OPERATION__RIGHT_OPERAND = eINSTANCE.getXBinaryOperation_RightOperand();
/**
* The meta object literal for the 'Reassign First Argument' attribute feature.
*
*
* @generated
*/
EAttribute XBINARY_OPERATION__REASSIGN_FIRST_ARGUMENT = eINSTANCE.getXBinaryOperation_ReassignFirstArgument();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XUnaryOperationImpl XUnary Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XUnaryOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXUnaryOperation()
* @generated
*/
EClass XUNARY_OPERATION = eINSTANCE.getXUnaryOperation();
/**
* The meta object literal for the 'Operand' containment reference feature.
*
*
* @generated
*/
EReference XUNARY_OPERATION__OPERAND = eINSTANCE.getXUnaryOperation_Operand();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XPostfixOperationImpl XPostfix Operation}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XPostfixOperationImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXPostfixOperation()
* @generated
*/
EClass XPOSTFIX_OPERATION = eINSTANCE.getXPostfixOperation();
/**
* The meta object literal for the 'Operand' containment reference feature.
*
*
* @generated
*/
EReference XPOSTFIX_OPERATION__OPERAND = eINSTANCE.getXPostfixOperation_Operand();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XForLoopExpressionImpl XFor Loop Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XForLoopExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXForLoopExpression()
* @generated
*/
EClass XFOR_LOOP_EXPRESSION = eINSTANCE.getXForLoopExpression();
/**
* The meta object literal for the 'For Expression' containment reference feature.
*
*
* @generated
*/
EReference XFOR_LOOP_EXPRESSION__FOR_EXPRESSION = eINSTANCE.getXForLoopExpression_ForExpression();
/**
* The meta object literal for the 'Each Expression' containment reference feature.
*
*
* @generated
*/
EReference XFOR_LOOP_EXPRESSION__EACH_EXPRESSION = eINSTANCE.getXForLoopExpression_EachExpression();
/**
* The meta object literal for the 'Declared Param' containment reference feature.
*
*
* @generated
*/
EReference XFOR_LOOP_EXPRESSION__DECLARED_PARAM = eINSTANCE.getXForLoopExpression_DeclaredParam();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XBasicForLoopExpressionImpl XBasic For Loop Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XBasicForLoopExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXBasicForLoopExpression()
* @generated
*/
EClass XBASIC_FOR_LOOP_EXPRESSION = eINSTANCE.getXBasicForLoopExpression();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XBASIC_FOR_LOOP_EXPRESSION__EXPRESSION = eINSTANCE.getXBasicForLoopExpression_Expression();
/**
* The meta object literal for the 'Each Expression' containment reference feature.
*
*
* @generated
*/
EReference XBASIC_FOR_LOOP_EXPRESSION__EACH_EXPRESSION = eINSTANCE.getXBasicForLoopExpression_EachExpression();
/**
* The meta object literal for the 'Init Expressions' containment reference list feature.
*
*
* @generated
*/
EReference XBASIC_FOR_LOOP_EXPRESSION__INIT_EXPRESSIONS = eINSTANCE.getXBasicForLoopExpression_InitExpressions();
/**
* The meta object literal for the 'Update Expressions' containment reference list feature.
*
*
* @generated
*/
EReference XBASIC_FOR_LOOP_EXPRESSION__UPDATE_EXPRESSIONS = eINSTANCE.getXBasicForLoopExpression_UpdateExpressions();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XAbstractWhileExpressionImpl XAbstract While Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAbstractWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAbstractWhileExpression()
* @generated
*/
EClass XABSTRACT_WHILE_EXPRESSION = eINSTANCE.getXAbstractWhileExpression();
/**
* The meta object literal for the 'Predicate' containment reference feature.
*
*
* @generated
*/
EReference XABSTRACT_WHILE_EXPRESSION__PREDICATE = eINSTANCE.getXAbstractWhileExpression_Predicate();
/**
* The meta object literal for the 'Body' containment reference feature.
*
*
* @generated
*/
EReference XABSTRACT_WHILE_EXPRESSION__BODY = eINSTANCE.getXAbstractWhileExpression_Body();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XDoWhileExpressionImpl XDo While Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XDoWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXDoWhileExpression()
* @generated
*/
EClass XDO_WHILE_EXPRESSION = eINSTANCE.getXDoWhileExpression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XWhileExpressionImpl XWhile Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XWhileExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXWhileExpression()
* @generated
*/
EClass XWHILE_EXPRESSION = eINSTANCE.getXWhileExpression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XTypeLiteralImpl XType Literal}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XTypeLiteralImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXTypeLiteral()
* @generated
*/
EClass XTYPE_LITERAL = eINSTANCE.getXTypeLiteral();
/**
* The meta object literal for the 'Type' reference feature.
*
*
* @generated
*/
EReference XTYPE_LITERAL__TYPE = eINSTANCE.getXTypeLiteral_Type();
/**
* The meta object literal for the 'Array Dimensions' attribute list feature.
*
*
* @generated
*/
EAttribute XTYPE_LITERAL__ARRAY_DIMENSIONS = eINSTANCE.getXTypeLiteral_ArrayDimensions();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XInstanceOfExpressionImpl XInstance Of Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XInstanceOfExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXInstanceOfExpression()
* @generated
*/
EClass XINSTANCE_OF_EXPRESSION = eINSTANCE.getXInstanceOfExpression();
/**
* The meta object literal for the 'Type' containment reference feature.
*
*
* @generated
*/
EReference XINSTANCE_OF_EXPRESSION__TYPE = eINSTANCE.getXInstanceOfExpression_Type();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XINSTANCE_OF_EXPRESSION__EXPRESSION = eINSTANCE.getXInstanceOfExpression_Expression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XThrowExpressionImpl XThrow Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XThrowExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXThrowExpression()
* @generated
*/
EClass XTHROW_EXPRESSION = eINSTANCE.getXThrowExpression();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XTHROW_EXPRESSION__EXPRESSION = eINSTANCE.getXThrowExpression_Expression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XTryCatchFinallyExpressionImpl XTry Catch Finally Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XTryCatchFinallyExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXTryCatchFinallyExpression()
* @generated
*/
EClass XTRY_CATCH_FINALLY_EXPRESSION = eINSTANCE.getXTryCatchFinallyExpression();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XTRY_CATCH_FINALLY_EXPRESSION__EXPRESSION = eINSTANCE.getXTryCatchFinallyExpression_Expression();
/**
* The meta object literal for the 'Finally Expression' containment reference feature.
*
*
* @generated
*/
EReference XTRY_CATCH_FINALLY_EXPRESSION__FINALLY_EXPRESSION = eINSTANCE.getXTryCatchFinallyExpression_FinallyExpression();
/**
* The meta object literal for the 'Catch Clauses' containment reference list feature.
*
*
* @generated
*/
EReference XTRY_CATCH_FINALLY_EXPRESSION__CATCH_CLAUSES = eINSTANCE.getXTryCatchFinallyExpression_CatchClauses();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XCatchClauseImpl XCatch Clause}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XCatchClauseImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXCatchClause()
* @generated
*/
EClass XCATCH_CLAUSE = eINSTANCE.getXCatchClause();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XCATCH_CLAUSE__EXPRESSION = eINSTANCE.getXCatchClause_Expression();
/**
* The meta object literal for the 'Declared Param' containment reference feature.
*
*
* @generated
*/
EReference XCATCH_CLAUSE__DECLARED_PARAM = eINSTANCE.getXCatchClause_DeclaredParam();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XAssignmentImpl XAssignment}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XAssignmentImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXAssignment()
* @generated
*/
EClass XASSIGNMENT = eINSTANCE.getXAssignment();
/**
* The meta object literal for the 'Assignable' containment reference feature.
*
*
* @generated
*/
EReference XASSIGNMENT__ASSIGNABLE = eINSTANCE.getXAssignment_Assignable();
/**
* The meta object literal for the 'Value' containment reference feature.
*
*
* @generated
*/
EReference XASSIGNMENT__VALUE = eINSTANCE.getXAssignment_Value();
/**
* The meta object literal for the 'Explicit Static' attribute feature.
*
*
* @generated
*/
EAttribute XASSIGNMENT__EXPLICIT_STATIC = eINSTANCE.getXAssignment_ExplicitStatic();
/**
* The meta object literal for the 'Static With Declaring Type' attribute feature.
*
*
* @generated
*/
EAttribute XASSIGNMENT__STATIC_WITH_DECLARING_TYPE = eINSTANCE.getXAssignment_StaticWithDeclaringType();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XReturnExpressionImpl XReturn Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XReturnExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXReturnExpression()
* @generated
*/
EClass XRETURN_EXPRESSION = eINSTANCE.getXReturnExpression();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XRETURN_EXPRESSION__EXPRESSION = eINSTANCE.getXReturnExpression_Expression();
/**
* The meta object literal for the '{@link org.eclipse.xtext.xbase.impl.XSynchronizedExpressionImpl XSynchronized Expression}' class.
*
*
* @see org.eclipse.xtext.xbase.impl.XSynchronizedExpressionImpl
* @see org.eclipse.xtext.xbase.impl.XbasePackageImpl#getXSynchronizedExpression()
* @generated
*/
EClass XSYNCHRONIZED_EXPRESSION = eINSTANCE.getXSynchronizedExpression();
/**
* The meta object literal for the 'Param' containment reference feature.
*
*
* @generated
*/
EReference XSYNCHRONIZED_EXPRESSION__PARAM = eINSTANCE.getXSynchronizedExpression_Param();
/**
* The meta object literal for the 'Expression' containment reference feature.
*
*
* @generated
*/
EReference XSYNCHRONIZED_EXPRESSION__EXPRESSION = eINSTANCE.getXSynchronizedExpression_Expression();
}
} //XbasePackage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy