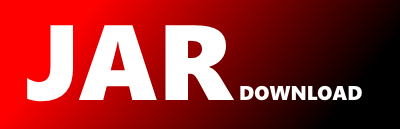
org.eclipse.xtext.xbase.conversion.XbaseValueConverterService Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2011 itemis AG (http://www.itemis.eu) and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*******************************************************************************/
package org.eclipse.xtext.xbase.conversion;
import java.util.Set;
import org.eclipse.xtext.common.services.DefaultTerminalConverters;
import org.eclipse.xtext.conversion.IValueConverter;
import org.eclipse.xtext.conversion.ValueConverter;
import org.eclipse.xtext.conversion.ValueConverterException;
import org.eclipse.xtext.conversion.impl.AbstractValueConverter;
import org.eclipse.xtext.conversion.impl.INTValueConverter;
import org.eclipse.xtext.conversion.impl.KeywordAlternativeConverter;
import org.eclipse.xtext.conversion.impl.KeywordBasedValueConverter;
import org.eclipse.xtext.conversion.impl.QualifiedNameValueConverter;
import org.eclipse.xtext.nodemodel.INode;
import org.eclipse.xtext.util.Strings;
import com.google.common.collect.ImmutableSet;
import com.google.inject.Inject;
import com.google.inject.Provider;
import com.google.inject.Singleton;
/**
* The value converter service for Xbase. It registers
* the {@link QualifiedNameValueConverter} and a {@link KeywordBasedValueConverter}
* for each operator.
* Clients, who extend Xbase should inherit from this value converter service.
*
* @author Sebastian Zarnekow - Initial contribution and API
*/
@Singleton
public class XbaseValueConverterService extends DefaultTerminalConverters {
public static class OtherOperatorsValueConverter extends AbstractValueConverter {
private final static Set operators = ImmutableSet.of(
"->",
"..",
"..<",
">..",
"=>",
">>",
">>>",
"<<",
"<<<",
"<>",
"?:",
"<=>");
@Override
public String toValue(String string, INode node) throws ValueConverterException {
return string;
}
@Override
public String toString(String value) throws ValueConverterException {
if (!operators.contains(value))
throw new ValueConverterException("'" + value + "' is not a valid operator.", null, null);
return value;
}
}
public static class CompareOperatorsValueConverter extends AbstractValueConverter {
private final static Set operators = ImmutableSet.of(
">=",
"<=",
">",
"<");
@Override
public String toValue(String string, INode node) throws ValueConverterException {
return string;
}
@Override
public String toString(String value) throws ValueConverterException {
if (!operators.contains(value))
throw new ValueConverterException("'" + value + "' is not a valid operator.", null, null);
return value;
}
}
public static class MultiAssignOperatorsValueConverter extends AbstractValueConverter {
private final static Set operators = ImmutableSet.of(
"+=",
"-=",
"*=",
"/=",
"&=",
"|=",
"^=",
"%=",
"<<=",
">>=",
">>>=");
@Override
public String toValue(String string, INode node) throws ValueConverterException {
return string;
}
@Override
public String toString(String value) throws ValueConverterException {
if (!operators.contains(value))
throw new ValueConverterException("'" + value + "' is not a valid operator.", null, null);
return value;
}
}
@Inject
private XbaseQualifiedNameValueConverter qualifiedNameValueConverter;
@Inject
private XbaseQualifiedNameInStaticImportValueConverter qualifiedNameInStaticImportValueConverter;
@Inject
private Provider keywordBasedConverterProvider;
@Inject
private OtherOperatorsValueConverter otherOperatorsValueConverter;
@Inject
private CompareOperatorsValueConverter compareOperatorsValueConverter;
@Inject
private MultiAssignOperatorsValueConverter multiAssignOperatorsValueConverter;
@Inject
private KeywordAlternativeConverter validIDConverter;
@Inject
private KeywordAlternativeConverter featureCallIDConverter;
@Inject
private KeywordAlternativeConverter idOrSuperConverter;
@ValueConverter(rule = "IdOrSuper")
public IValueConverter getIdOrSuperValueConverter() {
return idOrSuperConverter;
}
@ValueConverter(rule = "ValidID")
public IValueConverter getValidIDConverter() {
return validIDConverter;
}
@ValueConverter(rule = "FeatureCallID")
public IValueConverter getFeatureCallIDValueConverter() {
return featureCallIDConverter;
}
@ValueConverter(rule = "QualifiedName")
public IValueConverter getQualifiedNameValueConverter() {
return qualifiedNameValueConverter;
}
@ValueConverter(rule = "QualifiedNameWithWildcard")
public IValueConverter getQualifiedNameWithWildCardValueConverter() {
return getQualifiedNameValueConverter();
}
@ValueConverter(rule = "QualifiedNameInStaticImport")
public IValueConverter getQualifiedNameInStaticImportValueConverter() {
return qualifiedNameInStaticImportValueConverter;
}
@ValueConverter(rule = "OpSingleAssign")
public IValueConverter getOpSingleAssignConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpMultiAssign")
public IValueConverter getOpMultiAssignConverter() {
return multiAssignOperatorsValueConverter;
}
@ValueConverter(rule = "OpOr")
public IValueConverter getOpOrConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpAnd")
public IValueConverter getOpAndConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpEquality")
public IValueConverter getOpEqualityConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpCompare")
public IValueConverter getOpCompareConverter() {
return compareOperatorsValueConverter;
}
@ValueConverter(rule = "OpOther")
public IValueConverter getOpOtherConverter() {
return otherOperatorsValueConverter;
}
@ValueConverter(rule = "OpAdd")
public IValueConverter getOpAddConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpMulti")
public IValueConverter getOpMultiConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpUnary")
public IValueConverter getOpUnaryConverter() {
return keywordBasedConverterProvider.get();
}
@ValueConverter(rule = "OpPostfix")
public IValueConverter getOpPostfixConverter() {
return keywordBasedConverterProvider.get();
}
@Inject
private IntUnderscoreValueConverter intUnderscoreValueConverter;
@Override
@ValueConverter(rule = "INT")
public IValueConverter INT() {
return intUnderscoreValueConverter;
}
public static class IntUnderscoreValueConverter extends INTValueConverter {
@Override
public Integer toValue(String string, INode node) {
if (Strings.isEmpty(string))
throw new ValueConverterException("Couldn't convert empty string to an int value.", node, null);
String withoutUnderscore = string.replace("_", "");
if (Strings.isEmpty(withoutUnderscore))
throw new ValueConverterException("Couldn't convert input '" + string + "' to an int value.", node, null);
return super.toValue(withoutUnderscore, node);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy