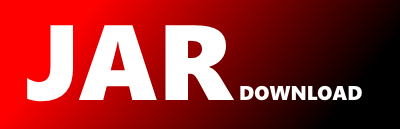
org.eclipse.ocl.expressions.impl.UnspecifiedValueExpImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ocl Show documentation
Show all versions of ocl Show documentation
OCL (Object Constraint Language) Parser and Interpreter
The newest version!
/*******************************************************************************
* Copyright (c) 2006, 2009 IBM Corporation, Zeligsoft Inc., and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM - Initial API and implementation
* Zeligsoft - Bug 207365
*******************************************************************************/
package org.eclipse.ocl.expressions.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.ocl.expressions.ExpressionsPackage;
import org.eclipse.ocl.expressions.UnspecifiedValueExp;
import org.eclipse.ocl.utilities.TypedASTNode;
import org.eclipse.ocl.utilities.UtilitiesPackage;
import org.eclipse.ocl.utilities.Visitor;
/**
*
* An implementation of the model object 'Unspecified Value Exp'.
*
*
* The following features are implemented:
*
* - {@link org.eclipse.ocl.expressions.impl.UnspecifiedValueExpImpl#getTypeStartPosition Type Start Position}
* - {@link org.eclipse.ocl.expressions.impl.UnspecifiedValueExpImpl#getTypeEndPosition Type End Position}
*
*
*
* @generated
*/
public class UnspecifiedValueExpImpl
extends OCLExpressionImpl
implements UnspecifiedValueExp {
/**
* The default value of the '{@link #getTypeStartPosition() Type Start Position}' attribute.
*
*
* @see #getTypeStartPosition()
* @generated
* @ordered
*/
protected static final int TYPE_START_POSITION_EDEFAULT = -1;
/**
* The cached value of the '{@link #getTypeStartPosition() Type Start Position}' attribute.
*
*
* @see #getTypeStartPosition()
* @generated
* @ordered
*/
protected int typeStartPosition = TYPE_START_POSITION_EDEFAULT;
/**
* The default value of the '{@link #getTypeEndPosition() Type End Position}' attribute.
*
*
* @see #getTypeEndPosition()
* @generated
* @ordered
*/
protected static final int TYPE_END_POSITION_EDEFAULT = -1;
/**
* The cached value of the '{@link #getTypeEndPosition() Type End Position}' attribute.
*
*
* @see #getTypeEndPosition()
* @generated
* @ordered
*/
protected int typeEndPosition = TYPE_END_POSITION_EDEFAULT;
/**
*
*
* @generated
*/
protected UnspecifiedValueExpImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return ExpressionsPackage.Literals.UNSPECIFIED_VALUE_EXP;
}
/**
*
*
* @generated
*/
public int getTypeStartPosition() {
return typeStartPosition;
}
/**
*
*
* @generated
*/
public void setTypeStartPosition(int newTypeStartPosition) {
int oldTypeStartPosition = typeStartPosition;
typeStartPosition = newTypeStartPosition;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET,
ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION,
oldTypeStartPosition, typeStartPosition));
}
/**
*
*
* @generated
*/
public int getTypeEndPosition() {
return typeEndPosition;
}
/**
*
*
* @generated
*/
public void setTypeEndPosition(int newTypeEndPosition) {
int oldTypeEndPosition = typeEndPosition;
typeEndPosition = newTypeEndPosition;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET,
ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION,
oldTypeEndPosition, typeEndPosition));
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION :
return getTypeStartPosition();
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION :
return getTypeEndPosition();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION :
setTypeStartPosition((Integer) newValue);
return;
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION :
setTypeEndPosition((Integer) newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION :
setTypeStartPosition(TYPE_START_POSITION_EDEFAULT);
return;
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION :
setTypeEndPosition(TYPE_END_POSITION_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION :
return typeStartPosition != TYPE_START_POSITION_EDEFAULT;
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION :
return typeEndPosition != TYPE_END_POSITION_EDEFAULT;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public int eBaseStructuralFeatureID(int derivedFeatureID, Class> baseClass) {
if (baseClass == TypedASTNode.class) {
switch (derivedFeatureID) {
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION :
return UtilitiesPackage.TYPED_AST_NODE__TYPE_START_POSITION;
case ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION :
return UtilitiesPackage.TYPED_AST_NODE__TYPE_END_POSITION;
default :
return -1;
}
}
return super.eBaseStructuralFeatureID(derivedFeatureID, baseClass);
}
/**
*
*
* @generated
*/
@Override
public int eDerivedStructuralFeatureID(int baseFeatureID, Class> baseClass) {
if (baseClass == TypedASTNode.class) {
switch (baseFeatureID) {
case UtilitiesPackage.TYPED_AST_NODE__TYPE_START_POSITION :
return ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_START_POSITION;
case UtilitiesPackage.TYPED_AST_NODE__TYPE_END_POSITION :
return ExpressionsPackage.UNSPECIFIED_VALUE_EXP__TYPE_END_POSITION;
default :
return -1;
}
}
return super.eDerivedStructuralFeatureID(baseFeatureID, baseClass);
}
/**
*
*
* @generated
*/
@Override
public String toString() {
if (eIsProxy())
return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (typeStartPosition: "); //$NON-NLS-1$
result.append(typeStartPosition);
result.append(", typeEndPosition: "); //$NON-NLS-1$
result.append(typeEndPosition);
result.append(')');
return result.toString();
}
/**
* @generated NOT
*/
@Override
@SuppressWarnings("unchecked")
public > T accept(U v) {
return ((Visitor) v)
.visitUnspecifiedValueExp(this);
}
} //UnspecifiedValueExpImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy