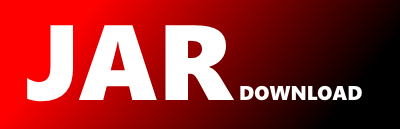
org.eclipse.osgi.internal.resolver.BaseDescriptionImpl Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2004, 2011 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
* Rob Harrop - SpringSource Inc. (bug 247522)
*******************************************************************************/
package org.eclipse.osgi.internal.resolver;
import java.util.*;
import java.util.Map.Entry;
import org.eclipse.osgi.service.resolver.BaseDescription;
import org.eclipse.osgi.service.resolver.extras.DescriptionReference;
import org.osgi.framework.Version;
import org.osgi.framework.wiring.BundleCapability;
import org.osgi.framework.wiring.BundleRevision;
public abstract class BaseDescriptionImpl implements BaseDescription {
protected final Object monitor = new Object();
private volatile String name;
private volatile Version version;
private volatile Object userObject;
public String getName() {
return name;
}
public Version getVersion() {
synchronized (this.monitor) {
if (version == null)
return Version.emptyVersion;
return version;
}
}
protected void setName(String name) {
this.name = name;
}
protected void setVersion(Version version) {
this.version = version;
}
static String toString(Map map, boolean directives) {
if (map.size() == 0)
return ""; //$NON-NLS-1$
String assignment = directives ? ":=" : "="; //$NON-NLS-1$//$NON-NLS-2$
Set> set = map.entrySet();
StringBuffer sb = new StringBuffer();
for (Entry entry : set) {
sb.append("; "); //$NON-NLS-1$
String key = entry.getKey();
Object value = entry.getValue();
if (value instanceof List) {
@SuppressWarnings("unchecked")
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy