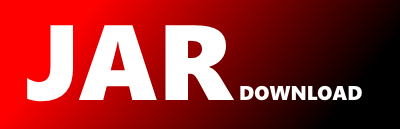
org.efaps.admin.datamodel.ui.BooleanUI Maven / Gradle / Ivy
/*
* Copyright 2003 - 2012 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 7700 $
* Last Changed: $Date: 2012-06-19 15:09:02 -0500 (Tue, 19 Jun 2012) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.admin.datamodel.ui;
import java.util.Map;
import java.util.TreeMap;
import org.efaps.admin.datamodel.Attribute;
import org.efaps.admin.dbproperty.DBProperties;
import org.efaps.admin.ui.AbstractUserInterfaceObject.TargetMode;
import org.efaps.admin.ui.field.Field;
import org.efaps.util.EFapsException;
/**
* A boolean value is shown in create mode with radio boxen which are only
* preselected if a defaultvalue for the attribute was defined. In edit mode,
* the user could select a value. The value is received from the DBProperties
* using the AttributeName and a parameter.
* e.g.
* Key = TypeName/AttributeName.false
* Value = inactive
*
* @author The eFaps Team
* @version $Id: BooleanUI.java 7700 2012-06-19 20:09:02Z [email protected] $
*/
public class BooleanUI
extends AbstractUI
{
/**
* Needed for serialization.
*/
private static final long serialVersionUID = 1L;
/**
* {@inheritDoc}
*/
@Override
public String getEditHtml(final FieldValue _fieldValue)
{
final StringBuilder ret = new StringBuilder();
final Field field = _fieldValue.getField();
final Attribute attribute = _fieldValue.getAttribute();
final Boolean bool;
if (_fieldValue.getValue() instanceof Boolean) {
bool = (Boolean) _fieldValue.getValue();
} else if (_fieldValue.getValue() instanceof String && ((String) _fieldValue.getValue()).length() > 0) {
if (((String) _fieldValue.getValue()).equalsIgnoreCase("TRUE")) {
bool = true;
} else {
bool = false;
}
} else {
bool = null;
}
if (_fieldValue.getTargetMode().equals(TargetMode.SEARCH)) {
ret.append("")
.append("")
.append(getTrue(attribute)).append("
")
.append("").append(getFalse(attribute));
} else {
ret.append("").append(getTrue(attribute)).append("
")
.append("").append(getFalse(attribute));
}
return ret.toString();
}
/**
* {@inheritDoc}
*/
@Override
public String getReadOnlyHtml(final FieldValue _fieldValue)
{
String ret = null;
final Attribute attribute = _fieldValue.getAttribute();
if (_fieldValue.getValue() instanceof Boolean) {
final boolean bool = (Boolean) _fieldValue.getValue();
if (bool) {
ret = getTrue(attribute);
} else {
ret = getFalse(attribute);
}
}
return ret;
}
/**
* {@inheritDoc}
*/
@Override
public Object getObject4Compare(final FieldValue _fieldValue)
{
Object ret = null;
if (_fieldValue.getValue() instanceof Boolean) {
final boolean bool = (Boolean) _fieldValue.getValue();
if (bool) {
ret = getTrue(_fieldValue.getAttribute());
} else {
ret = getFalse(_fieldValue.getAttribute());
}
}
return ret;
}
/**
* {@inheritDoc}
*/
@Override
public int compare(final FieldValue _fieldValue,
final FieldValue _fieldValue2)
{
String value = null;
String value2 = null;
// in case we have a boolean
if (_fieldValue.getValue() instanceof Boolean && _fieldValue2.getValue() instanceof Boolean) {
if ((Boolean) _fieldValue.getValue()) {
value = getTrue(_fieldValue.getAttribute());
} else {
value = getFalse(_fieldValue.getAttribute());
}
if ((Boolean) _fieldValue2.getValue()) {
value2 = getTrue(_fieldValue.getAttribute());
} else {
value2 = getFalse(_fieldValue.getAttribute());
}
}
// in case we have allready a string
if (_fieldValue.getValue() instanceof String && _fieldValue2.getValue() instanceof String) {
value = (String) _fieldValue.getValue();
value2 = (String) _fieldValue2.getValue();
}
return value.compareTo(value2);
}
/**
* Method to evaluate a String representation for the boolean.
*
* @param _attribute Attribute the String representation is wanted for
* @return String representation, default "FALSE"
*/
private String getFalse(final Attribute _attribute)
{
String ret;
if (DBProperties.hasProperty(_attribute.getParent().getName() + "/" + _attribute.getName() + ".false")) {
ret = DBProperties.getProperty(_attribute.getParent().getName() + "/" + _attribute.getName() + ".false");
} else {
ret = "FALSE";
}
return ret;
}
/**
* Method to evaluate a String representation for the boolean.
*
* @param _attribute Attribute the String representation is wanted for
* @return String representation, default "TRUE"
*/
private String getTrue(final Attribute _attribute)
{
String ret;
if (DBProperties.hasProperty(_attribute.getParent().getName() + "/" + _attribute.getName() + ".true")) {
ret = DBProperties.getProperty(_attribute.getParent().getName() + "/" + _attribute.getName() + ".true");
} else {
ret = "TRUE";
}
return ret;
}
@Override
public Object getValue(final UIValue _uiValue)
throws EFapsException
{
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy