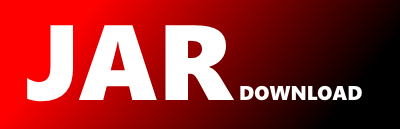
org.efaps.importer.ForeignObject Maven / Gradle / Ivy
/*
* Copyright 2003 - 2012 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 7483 $
* Last Changed: $Date: 2012-05-11 11:57:38 -0500 (Fri, 11 May 2012) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.importer;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.efaps.admin.datamodel.Type;
import org.efaps.db.MultiPrintQuery;
import org.efaps.db.QueryBuilder;
import org.efaps.util.EFapsException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This class presents an Object which is connected to an
* InsertObject
through an id.
*
* @author The eFaps Team
* @version $Id: ForeignObject.java 7483 2012-05-11 16:57:38Z [email protected] $
*/
public class ForeignObject
{
/**
* Logger for this class.
*/
private static final Logger LOG = LoggerFactory.getLogger(ForeignObject.class);
/**
* Contains the name of the attribute which links to an insert object.
*/
private String linkattribute = null;
/**
* Contains the type of this insert object.
*/
private String type = null;
/**
* Contains the attributes and the values used for the query.
*/
private final Map attributes = new HashMap();
/**
* The attribute to be selected. Default is "ID".
*
* @see #setLinkAttribute(String, String, String)
*/
private String attribute;
/**
* Adds an attribute, which will be used to construct the query.
*
* @param _name Name of the attribute
* @param _value Value of the attribute
*/
public void addAttribute(final String _name,
final String _value)
{
this.attributes.put(_name, _value.trim());
}
/**
* Sets the link attribute and the type of the foreign object.
*
* @param _name name of the link attribute
* @param _type type of the foreign object
* @param _select name of the field to be selected, default "ID"
*/
public void setLinkAttribute(final String _name,
final String _type,
final String _select)
{
this.linkattribute = _name;
this.type = _type;
this.attribute = _select != null ? _select : "ID";
}
/**
* Returns the {@link #linkattribute link attribute} of this foreign
* object.
*
* @return String containing the Name of the LinkAttribute
*/
public String getLinkAttribute()
{
return this.linkattribute;
}
/**
* Fetches the id of this foreign object from eFaps.
* To get the id a query is build. If the query returns
* null
, it will be checked if a default is defined for this
* foreign object. If is so the default is returned, otherwise
* null
.
*
* @return string with the id of the foreign object; null
if
* not found and no default is defined.
*/
public String dbGetValue()
{
String value = null;
try {
final QueryBuilder query = new QueryBuilder(Type.get(this.type));
for (final Entry element : this.attributes.entrySet()) {
query.addWhereAttrEqValue(element.getKey().toString(), element.getValue().toString());
}
final MultiPrintQuery multi = query.getPrint();
multi.addAttribute(this.attribute);
multi.executeWithoutAccessCheck();
if (multi.next()) {
value = multi.getAttribute(this.attribute).toString();
} else {
value = DefaultObject.getDefault(this.type, this.linkattribute);
if (value != null) {
ForeignObject.LOG.debug("Query did not return a Value; set Value to Defaultvalue: " + value);
} else {
ForeignObject.LOG.error("the Search for a ForeignObject did return no Result!: - " + toString());
}
}
} catch (final EFapsException e) {
ForeignObject.LOG.error("getID()", e);
value = null;
}
return value;
}
/**
* Returns the link representation for this foreign object including the
* {@link #type}, {@link #linkattribute link attribute} and all
* {@link #attributes}.
*
* @return string representation of this class
*/
@Override
public String toString()
{
return new ToStringBuilder(this)
.appendSuper(super.toString())
.append("type", this.type)
.append("link attribute", this.linkattribute)
.append("attributes", this.attributes.toString())
.toString();
}
}