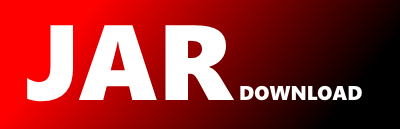
org.efaps.admin.common.SystemConfiguration Maven / Gradle / Ivy
/*
* Copyright 2003 - 2013 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8848 $
* Last Changed: $Date: 2013-02-19 10:49:59 -0500 (Tue, 19 Feb 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.admin.common;
import java.io.IOException;
import java.io.StringReader;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.UUID;
import org.efaps.admin.datamodel.Type;
import org.efaps.admin.user.Company;
import org.efaps.ci.CIAdminCommon;
import org.efaps.db.Context;
import org.efaps.db.Instance;
import org.efaps.db.transaction.ConnectionResource;
import org.efaps.db.wrapper.SQLPart;
import org.efaps.db.wrapper.SQLSelect;
import org.efaps.util.EFapsException;
import org.efaps.util.cache.CacheLogListener;
import org.efaps.util.cache.CacheObjectInterface;
import org.efaps.util.cache.CacheReloadException;
import org.efaps.util.cache.InfinispanCache;
import org.infinispan.Cache;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The class handles the caching for system configurations with their attributes
* and links.
*
* @author The eFaps Team
* @version $Id: SystemConfiguration.java 7483 2012-05-11 16:57:38Z
* [email protected] $
*/
public final class SystemConfiguration
implements CacheObjectInterface
{
/**
* This is the SQL select statement to select the configs from the database
* by ID.
*/
private static final String SQL_CONFIG = new SQLSelect()
.column(0, "TYPEID")
.column(0, "KEY")
.column(0, "VALUE")
.column(0, "COMPANYID")
.from("T_CMSYSCONF", 0)
.addPart(SQLPart.WHERE).addColumnPart(0, "ABSTRACTID").addPart(SQLPart.EQUAL).addValuePart("?")
.toString();
/**
* This is the SQL select statement to select a role from the database by
* ID.
*/
private static final String SQL_ID = new SQLSelect()
.column(0, "ID")
.column(0, "NAME")
.column(0, "UUID")
.from("T_CMABSTRACT", 0)
.addPart(SQLPart.WHERE).addColumnPart(0, "ID").addPart(SQLPart.EQUAL).addValuePart("?").toString();
/**
* This is the SQL select statement to select a role from the database by
* Name.
*/
private static final String SQL_NAME = new SQLSelect()
.column(0, "ID")
.column(0, "NAME")
.column(0, "UUID")
.from("T_CMABSTRACT", 0)
.addPart(SQLPart.WHERE).addColumnPart(0, "NAME").addPart(SQLPart.EQUAL).addValuePart("?")
.toString();
/**
* This is the SQL select statement to select a role from the database by
* UUID.
*/
private static final String SQL_UUID = new SQLSelect()
.column(0, "ID")
.column(0, "NAME")
.column(0, "UUID")
.from("T_CMABSTRACT", 0)
.addPart(SQLPart.WHERE).addColumnPart(0, "UUID").addPart(SQLPart.EQUAL).addValuePart("?")
.toString();
/**
* Name of the Cache by UUID.
*/
private static final String UUIDCACHE = "SystemConfiguration4UUID";
/**
* Name of the Cache by ID.
*/
private static final String IDCACHE = "SystemConfiguration4ID";
/**
* Name of the Cache by Name.
*/
private static final String NAMECACHE = "SystemConfiguration4Name";
/**
* Logging instance used in this class.
*/
private static final Logger LOG = LoggerFactory.getLogger(SystemConfiguration.class);
/**
* The instance variable stores the id of this SystemAttribute.
*
* @see #getId()
*/
private final long id;
/**
* The instance variable stores the UUID of this SystemAttribute.
*
* @see #getUUID()
*/
private final UUID uuid;
/**
* The instance variable stores the Name of this SystemAttribute.
*
* @see #getName()
*/
private final String name;
/**
* Map with all attributes for this system configuration.
*/
private final Map> attributes = new HashMap>();
/**
* Map with all links for this system configuration.
*/
private final Map> links = new HashMap>();
/**
* Map with all object attributes for this system configuration.
*/
private final Map> objectAttributes = new HashMap>();
/**
* Constructor setting instance variables.
*
* @param _id id of the SystemConfiguration
* @param _uuid uuid of the SystemConfiguration
* @param _name name of the SystemConfiguration
*/
private SystemConfiguration(final long _id,
final String _name,
final String _uuid)
{
this.id = _id;
this.uuid = UUID.fromString(_uuid);
this.name = _name;
this.attributes.put(new Long(0), new HashMap());
this.links.put(new Long(0), new HashMap());
this.objectAttributes.put(new Long(0), new HashMap());
}
/**
* Returns for given parameter _id the instance of class
* {@link SystemConfiguration}.
*
* @param _id id of the system configuration
* @return instance of class {@link SystemConfiguration}
* @throws CacheReloadException on error
*/
public static SystemConfiguration get(final long _id)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(
SystemConfiguration.IDCACHE);
if (!cache.containsKey(_id)) {
SystemConfiguration.getSystemConfigurationFromDB(SystemConfiguration.SQL_ID, _id);
}
return cache.get(_id);
}
/**
* Returns for given parameter _name the instance of class
* {@link SystemConfiguration}.
*
* @param _name name of the system configuration
* @return instance of class {@link SystemConfiguration}
* @throws CacheReloadException on error
*/
public static SystemConfiguration get(final String _name)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(
SystemConfiguration.NAMECACHE);
if (!cache.containsKey(_name)) {
SystemConfiguration.getSystemConfigurationFromDB(SystemConfiguration.SQL_NAME, _name);
}
return cache.get(_name);
}
/**
* Returns for given parameter _uuid the instance of class
* {@link SystemConfiguration}.
*
* @param _uuid uuid of the system configuration
* @return instance of class {@link SystemConfiguration}
* @throws CacheReloadException on error
*/
public static SystemConfiguration get(final UUID _uuid)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(
SystemConfiguration.UUIDCACHE);
if (!cache.containsKey(_uuid)) {
SystemConfiguration.getSystemConfigurationFromDB(SystemConfiguration.SQL_UUID, String.valueOf(_uuid));
}
return cache.get(_uuid);
}
/**
* This is the getter method for the instance variable {@link #id}.
*
* @return value of instance variable {@link #id}
*/
public long getId()
{
return this.id;
}
/**
* This is the getter method for the instance variable {@link #uuid}.
*
* @return value of instance variable {@link #uuid}
*/
public UUID getUUID()
{
return this.uuid;
}
/**
* This is the getter method for the instance variable {@link #name}.
*
* @return value of instance variable {@link #name}
*/
public String getName()
{
return this.name;
}
/**
* Get a value from the maps. The following logic applies:
*
* - Check if a Context exists
* - If a Context exist check if a company is given
* - If a company is given, check for a company specific map
* - If a company specific map is given search for the key in this map
* - If any of the earlier point fails the value from the default map is
* returned
*
*
* @param _key key the value is wanted for
* @param _map map the key will be search in for
* @return String value
* @throws EFapsException on error
*/
private String getValue(final String _key,
final Map> _map)
throws EFapsException
{
Company company = null;
if (Context.isThreadActive()) {
company = Context.getThreadContext().getCompany();
}
final long companyId = company == null ? 0 : company.getId();
Map innerMap;
if (_map.containsKey(companyId)) {
innerMap = _map.get(companyId);
if (!innerMap.containsKey(_key)) {
innerMap = _map.get(new Long(0));
}
} else {
innerMap = _map.get(new Long(0));
}
return innerMap.get(_key);
}
/**
* Returns for given _key
the related link. If no link is found
* null
is returned.
*
* @param _key key of searched link
* @return found link; if not found null
* @throws EFapsException on error
* @see #links
*/
public Instance getLink(final String _key)
throws EFapsException
{
return Instance.get(getValue(_key, this.links));
}
/**
* Returns for given Instance
the related attribute value. If
* no attribute value is found null
is returned.
*
* @param _instance Instance of searched objectattribute
* @return found attribute value; if not found null
* @throws EFapsException on error
* @see #objectAttributes
*/
public String getObjectAttributeValue(final Instance _instance)
throws EFapsException
{
return getObjectAttributeValue(_instance.getOid());
}
/**
* Returns for given OID
the related attribute value. If no
* attribute value is found null
is returned.
*
* @param _oid OID of searched objectattribute
* @return found attribute value; if not found null
* @throws EFapsException on error
* @see #objectAttributes
*/
public String getObjectAttributeValue(final String _oid)
throws EFapsException
{
return getValue(_oid, this.objectAttributes);
}
/**
* Returns for given Instance
the related value as Properties.
* If no attribute is found an empty Properties is returned.
*
* @param _instance Instance of searched attribute
* @return Properties
* @throws EFapsException on error
* @see #objectAttributes
*/
public Properties getObjectAttributeValueAsProperties(final Instance _instance)
throws EFapsException
{
return getObjectAttributeValueAsProperties(_instance.getOid());
}
/**
* Returns for given OID
the related value as Properties. If no
* attribute is found an empty Properties is returned.
*
* @param _key key of searched attribute
* @return Properties
* @throws EFapsException on error
* @see #objectAttributes
*/
public Properties getObjectAttributeValueAsProperties(final String _key)
throws EFapsException
{
final Properties ret = new Properties();
final String value = getValue(_key, this.objectAttributes);
if (value != null) {
try {
ret.load(new StringReader(value));
} catch (final IOException e) {
throw new EFapsException(SystemConfiguration.class, "getObjectAttributeValueAsProperties", e);
}
}
return ret;
}
/**
* Returns for given _key
the related attribute value. If no
* attribute value is found null
is returned.
*
* @param _key key of searched attribute
* @return found attribute value; if not found null
* @throws EFapsException on error
* @see #attributes
*/
public String getAttributeValue(final String _key)
throws EFapsException
{
return getValue(_key, this.attributes);
}
/**
* Returns for given _key
the related boolean attribute value.
* If no attribute value is found false is returned.
*
* @param _key key of searched attribute
* @return found boolean attribute value; if not found false
* @throws EFapsException on error
* @see #attributes
*/
public boolean getAttributeValueAsBoolean(final String _key)
throws EFapsException
{
final String value = getAttributeValue(_key);
return value == null ? false : Boolean.parseBoolean(value);
}
/**
* Returns for given _key
the related integer attribute value.
* If no attribute is found 0
is returned.
*
* @param _key key of searched attribute
* @return found integer attribute value; if not found 0
* @throws EFapsException on error
* @see #attributes
*/
public int getAttributeValueAsInteger(final String _key)
throws EFapsException
{
final String value = getAttributeValue(_key);
return value == null ? 0 : Integer.parseInt(value);
}
/**
* Returns for given _key
the related value as Properties. If
* no attribute is found an empty Properties is returned.
*
* @param _key key of searched attribute
* @return Properties
* @throws EFapsException on error
* @see #attributes
*/
public Properties getAttributeValueAsProperties(final String _key)
throws EFapsException
{
final Properties ret = new Properties();
final String value = getAttributeValue(_key);
if (value != null) {
try {
ret.load(new StringReader(value));
} catch (final IOException e) {
throw new EFapsException(SystemConfiguration.class, "getAttributeValueAsProperties", e);
}
}
return ret;
}
/**
* Read the config.
* @throws CacheReloadException on error
*/
private void readConfig()
throws CacheReloadException
{
ConnectionResource con = null;
try {
boolean closeContext = false;
if (!Context.isThreadActive()) {
Context.begin();
closeContext = true;
}
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy