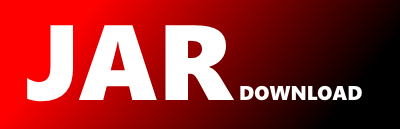
org.efaps.admin.datamodel.Status Maven / Gradle / Ivy
/*
* Copyright 2003 - 2013 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8895 $
* Last Changed: $Date: 2013-02-19 21:48:54 -0500 (Tue, 19 Feb 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.admin.datamodel;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.UUID;
import org.efaps.admin.dbproperty.DBProperties;
import org.efaps.db.Context;
import org.efaps.db.transaction.ConnectionResource;
import org.efaps.db.wrapper.SQLPart;
import org.efaps.db.wrapper.SQLSelect;
import org.efaps.util.EFapsException;
import org.efaps.util.cache.CacheLogListener;
import org.efaps.util.cache.CacheObjectInterface;
import org.efaps.util.cache.CacheReloadException;
import org.efaps.util.cache.InfinispanCache;
import org.infinispan.Cache;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* TODO comment!
*
* @author The eFaps Team
* @version $Id: Status.java 8895 2013-02-20 02:48:54Z [email protected] $
*/
public final class Status
implements CacheObjectInterface
{
/**
* This is the SQL select statement to select a role from the database by
* ID.
*/
private static final String SQL_ID4STATUS = new SQLSelect()
.column("ID")
.column("TYPEID")
.column("KEY")
.column("DESCR")
.from("T_DMSTATUS", 0)
.addPart(SQLPart.WHERE).addColumnPart(0, "ID").addPart(SQLPart.EQUAL).addValuePart("?").toString();
/**
* This is the SQL select statement to select a role from the database by
* Name.
*/
private static final String SQL_NAME4GRP = new SQLSelect()
.column(0, "ID")
.column(0, "TYPEID")
.column("KEY")
.column("DESCR")
.from("T_DMSTATUS", 0)
.innerJoin("T_CMABSTRACT", 1, "ID", 0, "TYPEID")
.addPart(SQLPart.WHERE).addColumnPart(1, "NAME").addPart(SQLPart.EQUAL).addValuePart("?")
.toString();
/**
* This is the SQL select statement to select a role from the database by
* UUID.
*/
private static final String SQL_UUID4GRP = new SQLSelect()
.column(0, "ID")
.column(0, "TYPEID")
.column("KEY")
.column("DESCR")
.from("T_DMSTATUS", 0)
.innerJoin("T_CMABSTRACT", 1, "ID", 0, "TYPEID")
.addPart(SQLPart.WHERE).addColumnPart(1, "UUID").addPart(SQLPart.EQUAL).addValuePart("?")
.toString();
/**
* Name of the Cache by UUID.
*/
private static final String UUIDCACHE4GRP = "StatusGroup4UUID";
/**
* Name of the Cache by ID.
*/
private static final String IDCACHE4STATUS = "Status4ID";
/**
* Name of the Cache by Name.
*/
private static final String NAMECACHE4GRP = "StatusGroup4Name";
/**
* Logging instance used in this class.
*/
private static final Logger LOG = LoggerFactory.getLogger(Status.class);
/**
* Id of this Status.
*/
private final long id;
/**
* Key of this status.
*/
private final String key;
/**
* Description for this status.
*/
private final String desc;
/**
* StatusGroup this Status belongs to.
*/
private final StatusGroup statusGroup;
/**
* @param _statusGroup StatusGroup this Status belongs to
* @param _id Id of this Status
* @param _key Key of this status.
* @param _desc Description for this status
*/
private Status(final StatusGroup _statusGroup,
final long _id,
final String _key,
final String _desc)
{
this.statusGroup = _statusGroup;
this.id = _id;
this.key = _key;
this.desc = _desc;
}
/**
* Getter method for instance variable {@link #id}.
*
* @return value of instance variable {@link #id}
*/
public long getId()
{
return this.id;
}
/**
* {@inheritDoc}
*/
@Override
public String getName()
{
throw new Error();
}
/**
* {@inheritDoc}
*/
@Override
public UUID getUUID()
{
throw new Error();
}
/**
* Getter method for instance variable {@link #key}.
*
* @return value of instance variable {@link #key}
*/
public String getKey()
{
return this.key;
}
/**
* Getter method for instance variable {@link #desc}.
*
* @return value of instance variable {@link #desc}
*/
public String getDescription()
{
return this.desc;
}
/**
* Method to get the key to the label.
*
* @return key to the label
*/
public String getLabelKey()
{
final StringBuilder keyStr = new StringBuilder();
return keyStr.append(this.statusGroup.getName()).append("/Key.Status.").append(this.key).toString();
}
/**
* Method to get the translated label for this Status.
*
* @return translated Label
*/
public String getLabel()
{
return DBProperties.getProperty(getLabelKey());
}
/**
* Getter method for instance variable {@link #statusGroup}.
*
* @return value of instance variable {@link #statusGroup}
*/
public StatusGroup getStatusGroup()
{
return this.statusGroup;
}
/**
* Method to initialize the Cache of this CacheObjectInterface.
*
* @param _class class that called the method
* @throws CacheReloadException on error
*/
public static void initialize(final Class> _class)
throws CacheReloadException
{
if (InfinispanCache.get().exists(Status.UUIDCACHE4GRP)) {
InfinispanCache.get().getCache(Status.UUIDCACHE4GRP).clear();
} else {
InfinispanCache.get().getCache(Status.UUIDCACHE4GRP)
.addListener(new CacheLogListener(Status.LOG));
}
if (InfinispanCache.get().exists(Status.IDCACHE4STATUS)) {
InfinispanCache.get().getCache(Status.IDCACHE4STATUS).clear();
} else {
InfinispanCache.get().getCache(Status.IDCACHE4STATUS)
.addListener(new CacheLogListener(Status.LOG));
}
if (InfinispanCache.get().exists(Status.NAMECACHE4GRP)) {
InfinispanCache.get().getCache(Status.NAMECACHE4GRP).clear();
} else {
InfinispanCache.get().getCache(Status.NAMECACHE4GRP)
.addListener(new CacheLogListener(Status.LOG));
}
}
/**
* Method to initialize the Cache of this CacheObjectInterface.
*
* @throws CacheReloadException on error
*/
public static void initialize()
throws CacheReloadException
{
Status.initialize(Status.class);
}
/**
* Method to get a Status from the cache.
*
* @param _typeName name of the StatusGroup
* @param _key key of the Status
* @return Status
* @throws CacheReloadException on error
*/
public static Status find(final String _typeName,
final String _key)
throws CacheReloadException
{
return Status.get(_typeName).get(_key);
}
/**
* Method to get a Status from the cache.
*
* @param _uuid uuid of the StatusGroup
* @param _key key of the Status
* @return Status
* @throws CacheReloadException on error
*/
public static Status find(final UUID _uuid,
final String _key)
throws CacheReloadException
{
return Status.get(_uuid).get(_key);
}
/**
* Method to get a Status from the cache.
*
* @param _id id of the status wanted.
* @return Status
* @throws CacheReloadException on error
*/
public static Status get(final long _id)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(Status.IDCACHE4STATUS);
if (!cache.containsKey(_id)) {
Status.getStatusFromDB(Status.SQL_ID4STATUS, _id);
}
return cache.get(_id);
}
/**
* Method to get a StatusGroup from the cache.
*
* @param _typeName name of the StatusGroup wanted.
* @return StatusGroup
* @throws CacheReloadException on error
*/
public static StatusGroup get(final String _typeName)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(
Status.NAMECACHE4GRP);
if (!cache.containsKey(_typeName)) {
Status.getStatusGroupFromDB(Status.SQL_NAME4GRP, _typeName);
}
return cache.get(_typeName);
}
/**
* Method to get a StatusGroup from the cache.
*
* @param _uuid UUID of the StatusGroup wanted.
* @return StatusGroup
* @throws CacheReloadException on error
*/
public static StatusGroup get(final UUID _uuid)
throws CacheReloadException
{
final Cache cache = InfinispanCache.get().getCache(Status.UUIDCACHE4GRP);
if (!cache.containsKey(_uuid)) {
Status.getStatusGroupFromDB(Status.SQL_UUID4GRP, String.valueOf(_uuid));
}
return cache.get(_uuid);
}
/**
* Class for a group of stati.
*/
public static class StatusGroup
extends HashMap
implements CacheObjectInterface
{
/**
* Needed for serialization.
*/
private static final long serialVersionUID = 1L;
/**
* Type this StatusGroup represents.
*/
private final Type type;
/**
* @param _type type to set
*/
public StatusGroup(final Type _type)
{
this.type = _type;
}
/**
* {@inheritDoc}
*/
@Override
public long getId()
{
return this.type.getId();
}
/**
* {@inheritDoc}
*/
@Override
public String getName()
{
return this.type.getName();
}
/**
* {@inheritDoc}
*/
@Override
public UUID getUUID()
{
return this.type.getUUID();
}
}
/**
* @param _grp StatusGroup to be cached
*/
private static void cacheStatusGroup(final StatusGroup _grp)
{
final Cache nameCache = InfinispanCache.get().getCache(
Status.NAMECACHE4GRP);
if (!nameCache.containsKey(_grp.getName())) {
nameCache.put(_grp.getName(), _grp);
}
final Cache uuidCache = InfinispanCache.get().getCache(
Status.UUIDCACHE4GRP);
if (!uuidCache.containsKey(_grp.getName())) {
uuidCache.put(_grp.getUUID(), _grp);
}
}
/**
* @param _status Status to be cached
*/
private static void cacheStatus(final Status _status)
{
final Cache idCache = InfinispanCache.get().getCache(Status.IDCACHE4STATUS);
if (!idCache.containsKey(_status.getId())) {
idCache.put(_status.getId(), _status);
}
}
/**
* @param _sql SQL Statement to be executed
* @param _criteria filter criteria
* @return true if successful
* @throws CacheReloadException on error
*/
private static boolean getStatusGroupFromDB(final String _sql,
final Object _criteria)
throws CacheReloadException
{
boolean ret = false;
ConnectionResource con = null;
try {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy