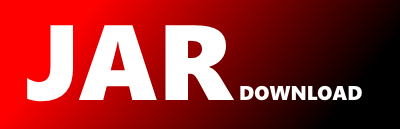
org.efaps.admin.ui.Form Maven / Gradle / Ivy
/*
* Copyright 2003 - 2013 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8896 $
* Last Changed: $Date: 2013-02-19 22:03:59 -0500 (Tue, 19 Feb 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.admin.ui;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import org.efaps.admin.datamodel.Type;
import org.efaps.ci.CIAdminUserInterface;
import org.efaps.util.EFapsException;
import org.efaps.util.cache.CacheReloadException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @author The eFaps TEam
* @version $Id: Form.java 8896 2013-02-20 03:03:59Z [email protected] $ TODO:
* description
*/
public class Form
extends AbstractCollection
{
/**
* Logging instance used in this class.
*/
protected static final Logger LOG = LoggerFactory.getLogger(Form.class);
/**
* Stores the mapping from type to tree menu.
*/
private static final Map TYPE2FORMS = new HashMap();
/**
* @param _id id
* @param _uuid UUID
* @param _name name
*/
public Form(final Long _id,
final String _uuid,
final String _name)
{
super(_id, _uuid, _name);
}
/**
* Sets the link properties for this object.
*
* @param _linkType type of the link property
* @param _toId to id
* @param _toType to type
* @param _toName to name
* @throws EFapsException on error
*/
@Override
protected void setLinkProperty(final Type _linkType,
final long _toId,
final Type _toType,
final String _toName)
throws EFapsException
{
if (_linkType.isKindOf(CIAdminUserInterface.LinkIsTypeFormFor.getType())) {
final Type type = Type.get(_toId);
if (type == null) {
Form.LOG.error("Form '" + getName() + "' could not defined as type form for type '" + _toName
+ "'! Type does not " + "exists!");
} else {
Form.TYPE2FORMS.put(type, this);
}
} else {
super.setLinkProperty(_linkType, _toId, _toType, _toName);
}
}
/**
* Returns for given parameter _id the instance of class {@link Form}
* .
*
* @param _id id to search in the cache
* @return instance of class {@link Form}
* @throws CacheReloadException on error
*/
public static Form get(final long _id)
throws CacheReloadException
{
return AbstractUserInterfaceObject.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy