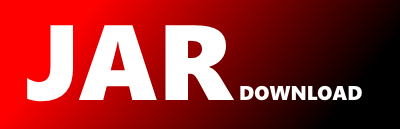
org.efaps.db.wrapper.AbstractSQLInsertUpdate Maven / Gradle / Ivy
/*
* Copyright 2003 - 2013 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8848 $
* Last Changed: $Date: 2013-02-19 10:49:59 -0500 (Tue, 19 Feb 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.db.wrapper;
import java.math.BigDecimal;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.sql.Types;
import java.util.ArrayList;
import java.util.List;
import org.efaps.db.Context;
/**
*
* @param original SQL statement class
* @author The eFaps Team
* @version $Id: AbstractSQLInsertUpdate.java 8848 2013-02-19 15:49:59Z [email protected] $
*/
public abstract class AbstractSQLInsertUpdate>
{
/**
* SQL table name to insert or update.
*
* @see #AbstractSQLInsertUpdate(String, String)
*/
private final String tableName;
/**
* Name of the id column.
*
* @see #AbstractSQLInsertUpdate(String, String)
*/
private final String idColumn;
/**
* Columns to insert or update.
*
* @see #columnWithSQLValue(String, String)
*/
private final List> columnWithValues = new ArrayList>();
/**
* Columns to insert or update.
*
* @see #columnWithSQLValue(String, String)
*/
private final List columnWithSQLValues = new ArrayList();
/**
* Initializes the {@link #tableName table name} to update.
*
* @param _tableName name of the table to update
* @param _idColumn name of the id column
*/
public AbstractSQLInsertUpdate(final String _tableName,
final String _idColumn)
{
this.tableName = _tableName;
this.idColumn = _idColumn;
}
/**
* Returns the {@link #tableName table name} to insert / update.
*
* @return table name to insert / update
* @see #tableName
*/
protected final String getTableName()
{
return this.tableName;
}
/**
* Returns the name of the {@link #idColumn id column}.
*
* @return name of the id column
* @see #idColumn
*/
protected final String getIdColumn()
{
return this.idColumn;
}
/**
* Returns the {@link #columnWithValues column with values}.
*
* @return columns with values
* @see #columnWithValues
*/
protected final List> getColumnWithValues()
{
return this.columnWithValues;
}
/**
* Returns the {@link #columnWithSQLValues column with SQL values}.
*
* @return columns with SQL values
* @see #columnWithSQLValues
*/
protected final List getColumnWithSQLValues()
{
return this.columnWithSQLValues;
}
/**
* Adds a new column which will have the current time stamp to append.
*
* @param _columnName name of column to append for which current time
* stamp must be set
* @return this SQL update instance
*/
@SuppressWarnings("unchecked")
public STMT columnWithCurrentTimestamp(final String _columnName)
{
this.columnWithSQLValues.add(
new AbstractSQLInsertUpdate.ColumnWithSQLValue(_columnName,
Context.getDbType().getCurrentTimeStamp()));
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link BigDecimal}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final BigDecimal _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.DECIMAL);
} else {
_stmt.setBigDecimal(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link Double}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final Double _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.DECIMAL);
} else {
_stmt.setDouble(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link String}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final String _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.VARCHAR);
} else {
_stmt.setString(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link Timestamp}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final Timestamp _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.TIMESTAMP);
} else {
_stmt.setTimestamp(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link Boolean}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final boolean _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.BOOLEAN);
} else {
_stmt.setBoolean(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link Long}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final Long _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.BIGINT);
} else {
_stmt.setLong(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Defines a new column _columnName
with {@link Long}
* _value
within this SQL insert / update statement.
*
* @param _columnName name of the column
* @param _value value of the column
* @return this SQL statement
*/
@SuppressWarnings("unchecked")
public STMT column(final String _columnName,
final Integer _value)
{
this.columnWithValues.add(new AbstractSQLInsertUpdate.AbstractColumnWithValue(_columnName, _value) {
@Override
public void set(final int _index, final PreparedStatement _stmt)
throws SQLException
{
if (getValue() == null) {
_stmt.setNull(_index, Types.BIGINT);
} else {
_stmt.setInt(_index, getValue());
}
}
});
return (STMT) this;
}
/**
* Abstract definition of a column.
*/
protected abstract static class AbstractColumn
{
/**
* Name of the SQL table column.
*/
private final String columnName;
/**
* Initializes the {@link #columnName column name}.
*
* @param _columnName name of the column
*/
private AbstractColumn(final String _columnName)
{
this.columnName = _columnName;
}
/**
* Returns the {@link #columnName column name}.
*
* @return column name
* @see #columnName
*/
public final String getColumnName()
{
return this.columnName;
}
}
/**
* Column with SQL value.
*/
protected static final class ColumnWithSQLValue
extends AbstractSQLInsertUpdate.AbstractColumn
{
/**
* SQL value to set for the column.
*/
private final String sqlValue;
/**
*
* @param _columnName column name
* @param _sqlValue SQL value
*/
private ColumnWithSQLValue(final String _columnName,
final String _sqlValue)
{
super(_columnName);
this.sqlValue = _sqlValue;
}
/**
* Returns related {@link #sqlValue SQL value}.
*
* @return SQL value
* @see #sqlValue
*/
public String getSqlValue()
{
return this.sqlValue;
}
}
/**
* Class holding the values depending on a column.
*
* @param class of the value
*/
protected abstract static class AbstractColumnWithValue
extends AbstractSQLInsertUpdate.AbstractColumn
{
/**
* SQL value to set for the column.
*/
private final VALUE value;
/**
* Initializes this column depending on the _columnName
* with _value
.
*
* @param _columnName column name
* @param _value value
*/
private AbstractColumnWithValue(final String _columnName,
final VALUE _value)
{
super(_columnName);
this.value = _value;
}
/**
* Returns the {@link #value} of this column.
*
* @return value of the column
*/
public VALUE getValue()
{
return this.value;
}
/**
*
*
* @param _index index in the prepared statement
* @param _stmt prepared statement
* @throws SQLException if value could not be set within the prepared
* statement _stmt
*/
public abstract void set(final int _index,
final PreparedStatement _stmt)
throws SQLException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy