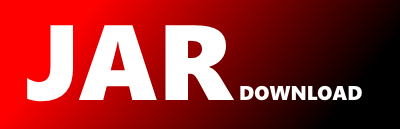
org.efaps.ui.wicket.components.tree.TreeCellPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of efaps-webapp Show documentation
Show all versions of efaps-webapp Show documentation
eFaps WebApp provides a web interface as the User Interface for eFaps
which can be easily expanded and altered.
/*
* Copyright 2003 - 2011 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev:1510 $
* Last Changed: $Date:2007-10-18 09:35:40 -0500 (Thu, 18 Oct 2007) $
* Last Changed By: $Author:jmox $
*/
package org.efaps.ui.wicket.components.tree;
import java.util.Iterator;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.TreeNode;
import org.apache.wicket.Component;
import org.apache.wicket.behavior.AttributeAppender;
import org.apache.wicket.behavior.SimpleAttributeModifier;
import org.apache.wicket.datetime.markup.html.form.DateTextField;
import org.apache.wicket.markup.ComponentTag;
import org.apache.wicket.markup.html.WebMarkupContainer;
import org.apache.wicket.markup.html.link.PopupSettings;
import org.apache.wicket.markup.html.panel.Panel;
import org.apache.wicket.model.IModel;
import org.apache.wicket.model.Model;
import org.efaps.admin.datamodel.ui.DateTimeUI;
import org.efaps.admin.datamodel.ui.DateUI;
import org.efaps.admin.ui.AbstractCommand.Target;
import org.efaps.admin.ui.field.Field.Display;
import org.efaps.ui.wicket.behaviors.AjaxFieldUpdateBehavior;
import org.efaps.ui.wicket.behaviors.ExpandTextareaBehavior;
import org.efaps.ui.wicket.behaviors.SetSelectedRowBehavior;
import org.efaps.ui.wicket.components.LabelComponent;
import org.efaps.ui.wicket.components.autocomplete.AutoCompleteField;
import org.efaps.ui.wicket.components.date.DateTimePanel;
import org.efaps.ui.wicket.components.date.UnnestedDatePickers;
import org.efaps.ui.wicket.components.efapscontent.StaticImageComponent;
import org.efaps.ui.wicket.components.picker.AjaxPickerLink;
import org.efaps.ui.wicket.components.table.cell.AjaxLinkContainer;
import org.efaps.ui.wicket.components.table.cell.AjaxLoadInOpenerLink;
import org.efaps.ui.wicket.components.table.cell.CheckOutLink;
import org.efaps.ui.wicket.components.table.cell.ContentContainerLink;
import org.efaps.ui.wicket.models.UIModel;
import org.efaps.ui.wicket.models.cell.UIStructurBrowserTableCell;
import org.efaps.ui.wicket.models.objects.UIStructurBrowser;
import org.efaps.util.EFapsException;
/**
* Class is used to render a cell inside a table.
*
* @author The eFaps Team
* @version $Id:CellPanel.java 1510 2007-10-18 14:35:40Z jmox $
*/
public class TreeCellPanel
extends Panel
{
/**
* Needed for serialization.
*/
private static final long serialVersionUID = 1L;
/**
* @param _wicketId wicketID for this Component
* @param _node treeNode the cell belongs to
* @param _index index of the column
* @param _updateListMenu must the list menu be update by a link
* @param _datePickers unnested DatePickers
* @throws EFapsException on error
*/
public TreeCellPanel(final String _wicketId,
final TreeNode _node,
final int _index,
final boolean _updateListMenu,
final UnnestedDatePickers _datePickers)
throws EFapsException
{
super(_wicketId);
final UIStructurBrowser uiStru = (UIStructurBrowser) ((DefaultMutableTreeNode) _node).getUserObject();
final UIStructurBrowserTableCell uiCell = uiStru.getColumnValue(_index);
final IModel cellModel = new UIModel(uiCell);
// set the title of the cell
add(new SimpleAttributeModifier("title", uiCell.getCellTitle()));
add(new AttributeAppender("style", true, new Model("text-align:" + uiCell.getAlign()), ";"));
if (uiCell.isHide()) {
add(new AttributeAppender("style", true, new Model("display:none"), ";"));
}
final WebMarkupContainer link;
if (uiCell.getReference() == null
|| (uiStru.isSearchMode() && uiCell.getTarget() != Target.POPUP && uiStru.isSubmit())
|| uiStru.isCreateMode() || uiStru.isEditMode()) {
link = new WebMarkupContainer("link")
{
private static final long serialVersionUID = 1L;
@Override
protected void onComponentTag(final ComponentTag _tag)
{
_tag.setName("span");
super.onComponentTag(_tag);
}
};
} else {
if (_updateListMenu && uiCell.getTarget() != Target.POPUP) {
link = new AjaxLinkContainer("link", cellModel);
} else {
if (uiCell.isCheckOut()) {
link = new CheckOutLink("link", cellModel);
} else {
if (uiStru.isSearchMode() && uiCell.getTarget() != Target.POPUP) {
link = new AjaxLoadInOpenerLink("link", cellModel);
} else {
link = new ContentContainerLink("link", cellModel);
if (uiCell.getTarget() == Target.POPUP) {
final PopupSettings popup = new PopupSettings("popup");
((ContentContainerLink>) link).setPopupSettings(popup);
}
}
}
}
}
add(link);
Component label;
if (uiCell.isAutoComplete() && uiCell.getDisplay().equals(Display.EDITABLE)) {
label = new AutoCompleteField("label", cellModel, false);
} else {
label = new LabelComponent("label", cellModel);
}
if (uiCell.getDisplay().equals(Display.EDITABLE)) {
label.add(new SetSelectedRowBehavior(uiCell.getName()));
if (uiCell.getUiClass() instanceof DateUI || uiCell.getUiClass() instanceof DateTimeUI) {
label = new DateTimePanel("label", uiCell.getCompareValue(), uiCell.getName(),
uiCell.getUiClass() instanceof DateTimeUI,
uiCell.getField().getCols());
if (uiCell.isFieldUpdate()) {
// the update behavior must be added to the inner text field
final Iterator extends Component> iter = ((WebMarkupContainer) label).iterator();
while (iter.hasNext()) {
final Component comp = iter.next();
if (comp instanceof DateTextField) {
comp.add(new AjaxFieldUpdateBehavior(uiCell.getFieldUpdateEvent(), cellModel));
break;
}
}
}
((DateTimePanel) label).getDatePicker().setUnNestedComponent(_datePickers);
} else {
if (uiCell.isFieldUpdate()) {
label.add(new AjaxFieldUpdateBehavior(uiCell.getFieldUpdateEvent(), cellModel));
}
if (uiCell.isMultiRows()) {
label.add(new ExpandTextareaBehavior());
}
}
link.add(new WebMarkupContainer("icon").setVisible(false));
} else {
if (uiCell.getIcon() != null && !uiCell.getIcon().isEmpty()) {
link.add(new StaticImageComponent("icon", uiCell.getIcon()));
} else {
link.add(new WebMarkupContainer("icon").setVisible(false));
}
}
link.add(label);
if (uiCell.isValuePicker() && uiCell.getDisplay().equals(Display.EDITABLE)) {
this.add(new AjaxPickerLink("valuePicker", cellModel, label));
} else {
add(new WebMarkupContainer("valuePicker").setVisible(false));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy