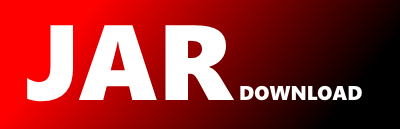
org.efaps.ui.wicket.components.classification.ClassificationPath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of efaps-webapp Show documentation
Show all versions of efaps-webapp Show documentation
eFaps WebApp provides a web interface as the User Interface for eFaps
which can be easily expanded and altered.
/*
* Copyright 2003 - 2012 The eFaps Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Revision: $Rev: 8900 $
* Last Changed: $Date: 2013-02-20 13:50:19 -0500 (Wed, 20 Feb 2013) $
* Last Changed By: $Author: [email protected] $
*/
package org.efaps.ui.wicket.components.classification;
import java.util.ArrayList;
import java.util.List;
import org.apache.wicket.markup.ComponentTag;
import org.apache.wicket.markup.MarkupStream;
import org.apache.wicket.markup.html.WebComponent;
import org.apache.wicket.model.IModel;
import org.efaps.ui.wicket.models.objects.UIClassification;
import org.efaps.util.cache.CacheReloadException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* TODO comment!
*
* @author The eFaps Team
* @version $Id: ClassificationPath.java 8900 2013-02-20 18:50:19Z [email protected] $
*/
public class ClassificationPath
extends WebComponent
{
/**
* Logging instance used in this class.
*/
private static final Logger LOG = LoggerFactory.getLogger(ClassificationPath.class);
/**
* Needed for serialization.
*/
private static final long serialVersionUID = 1L;
/**
* @param _wicketId wicket id of this component
* @param _model model for this component
*/
public ClassificationPath(final String _wicketId,
final IModel _model)
{
super(_wicketId, _model);
}
/**
* @see org.apache.wicket.Component#onComponentTagBody(org.apache.wicket.markup.MarkupStream,
* org.apache.wicket.markup.ComponentTag)
* @param _markupStream MarkupStream
* @param _openTag open tag
*/
@Override
public void onComponentTagBody(final MarkupStream _markupStream,
final ComponentTag _openTag)
{
super.onComponentTagBody(_markupStream, _openTag);
final StringBuilder html = new StringBuilder();
final UIClassification uiclass = (UIClassification) getDefaultModelObject();
if (!uiclass.isInitialized()) {
try {
uiclass.execute();
} catch (final CacheReloadException e) {
ClassificationPath.LOG.error("Error for execute", e);
}
}
final List leafs = new ArrayList();
if (uiclass.isSelected()) {
findSelectedLeafs(leafs, uiclass);
}
for (final UIClassification leaf : leafs) {
html.append("");
buildHtml(html, leaf);
html.append("");
}
replaceComponentTagBody(_markupStream, _openTag, html);
}
/**
* @param _leafs classification leaf
* @param _uiclass current classification
*/
private void findSelectedLeafs(final List _leafs,
final UIClassification _uiclass)
{
boolean add = true;
for (final UIClassification child : _uiclass.getChildren()) {
if (child.isSelected()) {
findSelectedLeafs(_leafs, child);
add = false;
}
}
if (add) {
_leafs.add(_uiclass);
}
}
/**
* @param _bldr StringBUilder for the snipplet
* @param _uiclass classifcation
*/
private void buildHtml(final StringBuilder _bldr,
final UIClassification _uiclass)
{
UIClassification tmp = _uiclass;
String path = "" + tmp.getLabel() + "";
while (!tmp.isRoot()) {
tmp = tmp.getParent();
path = "" + tmp.getLabel() + "" + path;
}
_bldr.append(path);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy